I recently started to play around with C++, primarily for my own personal side projects and came to find that it is a really interesting language to learn, especially at this stage of my learning process. As opposed to interpreted languages like Ruby and Javascript, C++ is a compiled language. There is a ton of great information about the differences between compiled languages vs interpreted languages out there so I won’t spend too much time discussing it in this post. In very basic terms, compiled languages require a compiler vs and interpreter (sort of self explanatory), but while they are called different things, their functionality is fairly similar in that it is responsible for taking the piece of code you wrote and turning it into something a computer can execute upon. In the case of C++, and other 'compiled' languages, is that this is a separate process. You must first run the compiler, and if all goes well, you will have an executable piece of code.
Another interesting fact about C++ is that it was one of the first languages to implement an object-oriented approach to programming. While there are some key distinctions between the class structure of Javascript and C++, primarily that under the hood JS still uses prototypal inheritance, with ES6 some of their visual structure is similar. The main difference, however, is that for a class declaration, conventionally you create two different files. One is a header that creates the class and declares all of its functions, and then a separate C++ file that defines all of the 'public' and 'private' methods for the class. The 'public' are able to be accessed anywhere in the program, whereas 'private' may only be accessed within the class. C++ also has a third method type called 'protected' which is similar to that of 'private' methods, but they can be accessed inside of child classes or derived classes. Similar to ES6 classes, there are also static methods in C++, which are part of the class definition but not a part of the object that it creates.
class Base {
public:
// public members go here
protected:
// protected members go here
private:
// private members go here
};
~~~~
One very odd thing about C++, at least for someone coming from a background of primarily using languages like Ruby/Javascript, is that C++ requires that you import libraries to do some of the most basic functions. For example, you have to import a standard input/output library called iostream in order to build a CLI application. C++ is also a strongly typed language which means, among many others, in any function declaration you must declare the data-type that you want said function to return. C++ also deals with manual memory allocation which is not a concept that I am currently well versed in, but one that I am very interested in learning about.
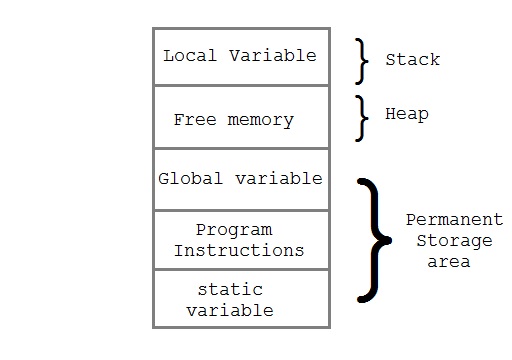
Essentially it involves understanding the different types of memory you have access to within a C++ program (heap/stack) and how the functions in your program take up space. There is also the concepts of references and pointers which deal with finding data based on specific memory addresses. In languages like Ruby or Javascript, all of these issues are things you will never have to think about while writing your program, but become an important topic when discussing scalability of C++ programs.
Top comments (5)
C++ is a wonderful language. A lot of modern and useful features are getting added to the language over the years. All with backward compatibility to C. The language has so many modern features with high performance, it is hard to believe that it is a 40 year old language!
It is also not all rosy though. It does have complex and confusing syntax at places. But all in all a great language to work with.
It is always great to see some interest for C++ on dev.to
I feel that C++ went from a decent language to a great one with the introduction of C++11. This introduced some real game changers.
And I agree that C++ definitely has some quirks. Unfortunately it seems that the C++ committee is unwilling to break backwards compatibility in order to fix some of these issues.
Still a great language to learn though!
C++ standard makes you never deal with memory allocation. You don't even use
malloc
like C, every memory allocation is made by thenew
keyword:But with C++ standard,
char *
and reallocation can be escaped:Same with arrays:
You still need to use manual memory allocation to instantiate many custom objects.
However, even if you do need to handle manual memory allocation, smart pointers handle nearly all of the scenarios where you'd need it.
Or, thanks to the relatively new
auto
keyword...std::shared_ptr
handles the allocation, deallocation, and lifetime. Alternatively, if you know you're only ever going to have one pointer to the object, you can usestd::unique_ptr
.Even still, it's good to know how to use
new
; every now and then, you still need it.If you are looking at C++ you should also take a look at Rust. Being an expert at C++ means you have a stackoverflow rep of xx,xxx. Being an expert at Rust means you don’t need to have a stackoverflow rep of xx,xxx.