Complete source code of this tutorial is available here — Ionic 4 Qrcode Barcode
This post is all about scanning QR code or Barcode and creating QR code in your cool new app. In this post, you will learn
- How to implement
phonegap-plugin-barcodescanner and cordova-plugin-qrscanner
in our app. - How to create
QR Code usng
these plugin into an ionic app.
Before start, first you will need an ionic app to start with, hence you can follow how to create an ionic app for beginners and start after that from here.
What is Ionic 4
I know most of the readers reading this blog will know what is Ionic 4, but just for the sake of beginners, I explain this in every blog.
Ionic is a complete open-source SDK for hybrid mobile app development. Ionic provides tools and services for developing hybrid mobile apps using Web technologies like CSS, HTML5, and Sass. Apps can be built with these Web technologies and then distributed through native app stores to be installed on devices.
In other words — If you create native apps in Android, you code in Java. If you create native apps in iOS, you code in Obj-C or Swift. Both of these are powerful, but complex languages. With Cordova (and Ionic) you can write a single piece of code for your app that can run on both iOS and Android (and windows!), that too with the simplicity of HTML, CSS, and JS. I’m a huge fan of Ionic and been developing Ionic apps for last 4 years.
QR code and Barcode— What and why?
QR code or barcode scanning is something almost every smartphone user has done at least once. We scan QR codes in supermarkets, on products in general, and oh, Amazon delivery! It’s a very handy way to recognize products instead of entering 16 digit long ID numbers etc. Similarly, reading ID numbers from Passports, etc could be very handy if you are an international hotel owner and require guests to carry passports as IDs. Or maybe you want to read off a vehicle registration number using your phone.
Here are some potential use cases for these plugins in an Ionic 4 app
- Super market app — QR/barcode scanners can provide product info to users
- Delivery app — Barcode scan can track/sign off a package
- Quick access to offers — Scan QR codes and go to a webpage
- Web authentication of a mobile app — Similar to Whatsapp Web
- Event app — Scan tickets or events passes
…… and many more
All this can now be done in Ionic apps, with the latest plugins available in Ionic 4. In this article, we will focus on such plugins. Some of these plugins have been around since Ionic 1, and have been changing ever since, and some are new. So here are some of the scan plugins and functionalities you can implement with Ionic 4.
Step 1.1 Barcode Scanner
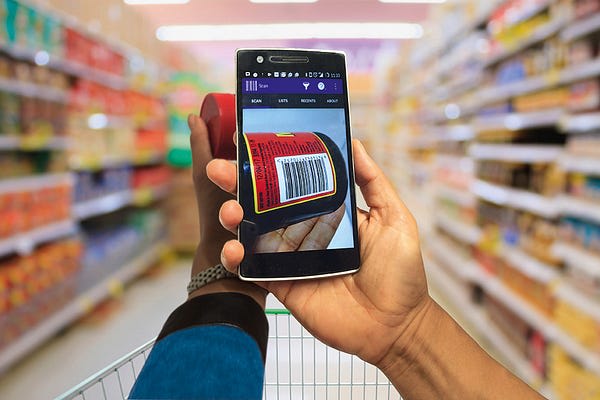

Barcodes and QR codes are widely used for multiple purposes like to add a link where a user doesn’t need to type the whole URL it can be easily scanned from a QR code. Barcodes can also be seen everywhere on the day to day items like eatables clothes etc, we can wimple scan these barcodes to get information like item code date of manufacturing, prices, etc.
The Barcode Scanner Plugin opens a camera view and automatically scans a barcode, returning the data back to you.
A barcode is an image consisting of a series of parallel black and white lines that, when scanned, relay information about a product. A barcode is used to automate the transfer of product information, for eg. its price, from the product to an electronic system such as a cash register. Barcodes are traditionally read by optical devices such as a barcode reader or scanner. New advances in technology allow consumers to scan barcodes with their smartphones and tablets using in-built cameras. Now you can do the same with your Ionic 4 app as well.
To start using this first you need to create an Ionic 4 project. To create an Ionic 4 project you can follow our tutorial on How to create Ionic 4 app for beginners.
Then install the following packages by executing this command:-
ionic cordova plugin add phonegap-plugin-barcodescanner
npm install @ionic-native/barcode-scanner
Now import the Barcode module in your projects app.module.ts
and then inject it inside the provider, so that you can use it as a dependency injection inside the constructor and access it all over the app components.
Add Button to scan a Barcode and to create a QR code
Now you are ready to use the Barcode function provided by the Ionic Plugin and get the result. Before this let us make a beautiful view to access the barcode function by clicking a button and getting the data in response.

After this, you can simply use the following function to complete a scan process.
This function will open the phone’s camera, allow you to scan a barcode, and in result will provide success
or error
.
You can also pass an options
object in the scan({options})
function. These options are
preferFrontCamera : true, // iOS and Android
showFlipCameraButton : true, // iOS and Android
showTorchButton : true, // iOS and Android
torchOn: true, // Android, launch with the torch switched on (if available)
saveHistory: true, // Android, save scan history (default false)
prompt : "Place a barcode inside the scan area", // Android
resultDisplayDuration: 500, // Android, display scanned text for X ms. 0 suppresses it entirely, default 1500
formats : "QR_CODE,PDF_417", // default: all but PDF_417 and RSS_EXPANDED
orientation : "landscape", // Android only (portrait|landscape), default unset so it rotates with the device
This plugin will, of course, require a camera and file saving access on the device, so take care of that as per your device. Since iOS 10 it’s mandatory to add NSCameraUsageDescription
.NSCameraUsageDescription
describes the reason that the app accesses the user's camera. When the system prompts the user to allow access, this string is displayed as part of the dialog box. If you didn't provide the usage description, the app will crash before showing the dialog. Also, Apple will reject apps that access private data but don't provide a usage description.
To add this entry you can use the edit-config
tag in the config.xml
like this:
<edit-config target="NSCameraUsageDescription" file="*-Info.plist" mode="merge">
<string>To scan barcodes</string>
</edit-config>
Let's go and check what we get on clicking on barcode scan button

When I type in the text field my name shadman to generate a QR code of my name then the result that I got is amazing….

Step 1.2 QRCode Scanner

Looks familiar? A Quick Response (QR) Code is a type of barcode that contains a matrix of dots. It can be scanned using a QR scanner or a smartphone with a built-in camera. Once scanned, software on the device converts the dots within the code into numbers or a string of characters. For example, scanning a QR code with your phone might open a URL in your phone’s web browser.
QR codes are able to contain more information than traditional barcodes. And now your Ionic 4 app can scan QR codes very efficiently.
Come let's go to check how a QR Scanner works!
Step 1:- Install the QR Scanner plugin by executing this command
ionic cordova plugin add cordova-plugin-qrscanner
npm install @ionic-native/qr-scanner
Now import the QR Scan module in your app.module.ts
as we have done above for Barcode Scanner.
Add Button to scan a QRCode and to get the result
Now you are ready to use the QRCode Scanner function provided by the Ionic Plugin and get the result. Before this let us make a beautiful view to access the QRCode function by clicking a button and getting the data in response.
The Result for the above code is:
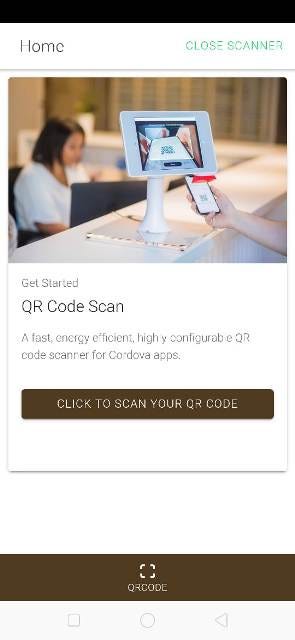
As we have done before for Barcode, you can simply use the Below function to complete a QR Code scan process.
If camera permission was permanently denied you must use QRScanner.openSettings()
method to guide the user to the settings page then they can grant the permission from there.
You can use QRScanner.useFrontCamera()
to use front-camera for scanning as well.
Use pausePreview
to pause the scanning while the camera is ON.
Use resumePreview
to resume the scanning.
Use destroy
to run hide
, cancelScan
, stop video capture, remove the video preview, and to deallocate as much as possible.
For more details, you can visit https://github.com/bitpay/cordova-plugin-qrscanner
Now when you click on the button to scan a QR code the result you will get will be amazingly like the below image but before this, it will prompt you for camera permission.
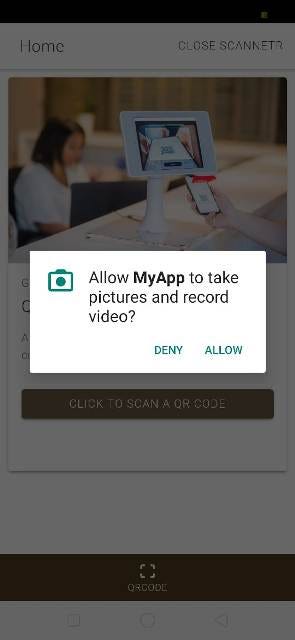

The result will be:

Here in the result, I have scanned the QR code generated in the barcode tutorial above. You can follow all the steps and achieve this very easily or you can clone my repo on Github for both the plugins here and enjoy 😎 😎 😎 🕺 🕺 🕺..…
Conclusion
In this post, we learned how to integrate Barcode scanner and QR Code scanner and how to generate your own QR Code using phonegap-plugin-barcodescanner
in Ionic 4 apps. Since both the plugins are amazingly light and have great documentation, it is developers’ first choice when it comes to creating QR code or Scanning a barcode or QR Code.
Complete source code of this tutorial is available here — Ionic 4 QRcodeBarcode
Next Steps
Now that you have learned the implementation of phonegap-plugin-barcodescanner and cordova-plugin-qrscanner
in Ionic 4, you can also try
- Ionic 4 PayPal payment integration — for Apps and PWA
- Ionic 4 Stripe payment integration — for Apps and PWA
- Ionic 4 Apple Pay integration
- Twitter login in Ionic 4 with Firebase
- Facebook login in Ionic 4 with Firebase
- Geolocation in Ionic 4
- QR Code and scanners in Ionic 4 and
- Translations in Ionic 4
If you need a base to start your next Ionic 4 app, you can make your next awesome app using Ionic 4 Full App
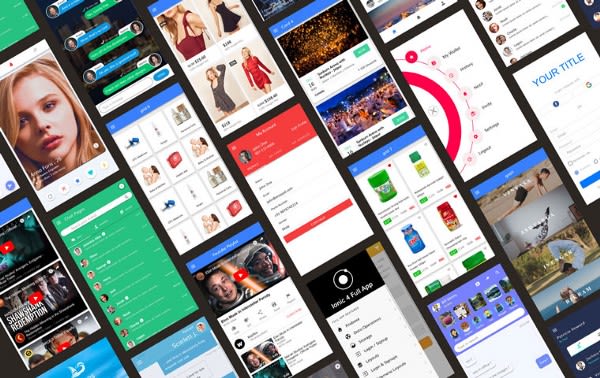
Top comments (0)