Due to the technicality of web designs, it can be challenging to use other CSS properties for creating layouts. To overcome this, the CSS Flexbox came into the picture. CSS Flexbox makes it possible for us to build flexible layouts without the use of CSS float
and positioning. In this article, we’ll provide a CSS Flexbox cheat sheet and explain hos it is useful. In addition, we’ll learn the Flexbox architecture as well as the different properties of its components.
What is Flexbox?
A Flexible Box Module, also known as a Flexbox, is a mechanism for building layouts in one dimension. Unlike the CSS Grid Layout, which creates a two-dimensional layout by creating rows and columns simultaneously, the Flexbox creates a layout as either a flex row or flex column. Flexbox enables the arrangement of spaces and alignment between elements in a webpage.
Four layout models (block, inline, table, positioned) existed before the Flexbox. However, they lack the flexibility that Flexbox offers, such as resizing, changing orientation, and shrinking and stretching elements of web pages.
Why Use Flexbox?
The Flexbox offers many conveniences for building web pages, and can be used for the following:
- To arrange elements in web pages neatly by aligning them
- To create responsive layouts
- To space the items on web pages
- To wrap many lines (even though it is a single line by default)
- To get maximum flexibility in building layouts
- To set order and sequence while arranging elements of web pages
- To reorder items
- Mobile-friendly development
Flexbox Architecture
The Flexbox architecture comprises two axes:
Main Axis
The flex items (content) move as a group on this axis. The flex-direction
property determines the layout position. Therefore, if you have a row, the main axis will be along the row, and if you have a column, it will be along the column. The main axis moves from left to right by default. The starting point is called the main start
and the endpoint is called the main end
. The distance between the main start and the main end is called main size
.
Cross Axis
The cross axis moves perpendicular to the main axis. Thus, if the flex-direction
is a row, it moves along the column, and vice-versa. On the cross axis, you can align items against each other and the flex container by moving them individually or as a group. You can also control the flex spacing in wrapped flex lines by treating them as a group. The cross axis moves from top to bottom by default. The starting point is called the cross start
and the endpoint is called the cross end
. Cross size
is the distance between the cross start
and the cross end
.
Let’s look at a flexbox example regarding the architecture:
Components of Flexbox
Flexbox is comprised of two components:
CSS Flex Container
The CSS flex container is also known as the parent element
. Therefore, for us to create a Flexbox, we need the parent element with display: flex
set on it. The flex layout allows the container to change the width or height of the item to establish order and fill the best available space, primarily to accommodate all display devices and screen sizes. Simply put, the flex container expands items to fill any open space, or shrinks the space to prevent overflow.
CSS Flex Items
The direct children of the parent element (flex container) are flex items
, also called child elements
. The flex items appear in a row and start from the main axis' starting edge.
Let’s look at an example of how the CSS flex container and items work:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
background-color: Purple;
width: 800px;
}
.flex-container > div {
background-color: Orange;
margin: 30px;
padding: 20px;
font-size: 20px;
}
</style>
</head>
<body>
<h1>Flex Container</h1>
<p>Four Flex Items contained in a Flex Container</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
The flex items are the flexible boxes (in brown, blue, yellow, and red) inside the purple flex container.
Flex Container Properties
The CSS flex container has several properties that help explain its role in building layouts on our web pages. We’ll discuss these flexbox properties below.
Flex Direction
This CSS flex property establishes how we place flex items in flex containers by determining the direction of the main axis. The flex direction is set to a row by default. However, if we change it to a flex column, the main axis changes and displays the item in a column. The following values are possible with the flex-direction
property:
-
row value
: This value arranges the flex items horizontally, from left to right. Here is an example of how row value works:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
flex-direction: row;
background-color: Purple;
width: 800px;
}
.flex-container > div {
background-color: Orange;
width: 50px;
margin: 20px;
padding: 20px;
font-size: 20px;
}
</style>
</head>
<body>
<h1>Flex Direction</h1>
<p>Flex-direction row value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:

-
row-reverse value
: This value arranges the flex items horizontally, but from right to left. Let’s see how this works with this example:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
flex-direction: row-reverse;
background-color: Purple;
width: 800px;
}
.flex-container > div {
background-color: Orange;
width: 50px;
margin: 20px;
padding: 20px;
font-size: 20px;
}
</style>
</head>
<body>
<h1>Flex Direction</h1>
<p>Flex-direction row-reverse value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:
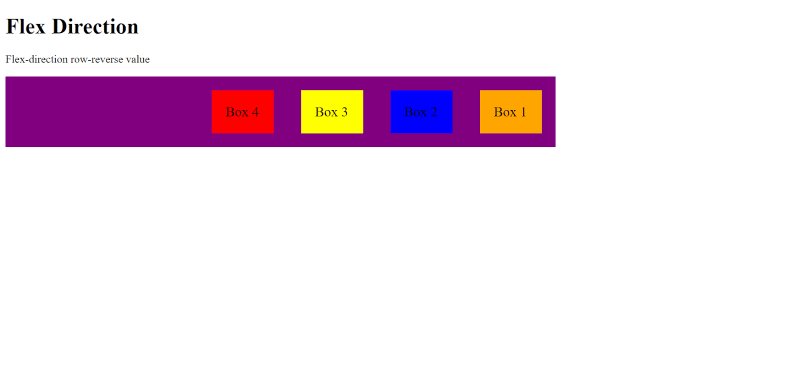
-
column value
: This value arranges the flex items vertically, from top to bottom. Here is an example of column value:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
flex-direction: column;
background-color: Purple;
width: 800px;
}
.flex-container > div {
background-color: Orange;
width: 50px;
margin: 20px;
padding: 20px;
font-size: 20px;
}
</style>
</head>
<body>
<h1>Flex Direction</h1>
<p>Flex-direction column value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:

-
column-reverse value
: This value arranges the flex items vertically, but from bottom to top. Here is an example, below:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
flex-direction: column-reverse;
background-color: Purple;
width: 800px;
}
.flex-container > div {
background-color: Orange;
width: 50px;
margin: 20px;
padding: 20px;
font-size: 20px;
}
</style>
</head>
<body>
<h1>Flex Direction</h1>
<p>Flex-direction column-reverse value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:
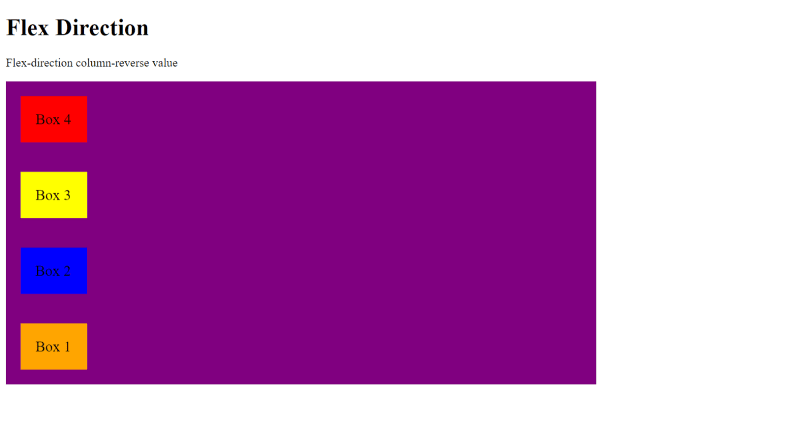
Flex-wrap
This Flex property defines whether or not a flex item should wrap in the case of insufficient space on one flex line.
To understand flex-wrap
, let’s look at the different values for this flex property:
-
nowrap value
: This is the default setting of flex-wrap. It defines that the flex items will not wrap, as shown below:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
flex-wrap: nowrap;
background-color: Purple;
width: 800px;
}
.flex-container > div {
background-color: Orange;
width: 50px;
margin: 20px;
padding: 20px;
font-size: 20px;
}
</style>
</head>
<body>
<h1>Flex Wrap</h1>
<p>Flex-wrap nowrap value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:

-
wrap value
: This defines that the flex items will wrap if needed:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
flex-wrap: wrap;
background-color: Purple;
width: 200px;
}
.flex-container > div {
background-color: Orange;
width: 50px;
font-size: 20px;
height: 50px;
}
</style>
</head>
<body>
<h1>Flex Wrap</h1>
<p>Flex-wrap wrap value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:

-
wrap-reverse value
: This defines that the flex items will wrap in the reverse order if necessary, as shown below:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
flex-wrap: wrap-reverse;
background-color: Purple;
width: 200px;
height: 200px
}
.flex-container > div {
background-color: Orange;
width: 50px;
font-size: 20px;
height: 50px;
}
</style>
</head>
<body>
<h1>Flex Wrap</h1>
<p>Flex-wrap wrap-reverse value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:

Justify Content
This CSS flex property helps to align the flex items based on the main axis within a Flex container. This occurs when the flex items do not use all open space on the main axis, thereby aligning horizontally. In addition, the property allows you to adjust to the left, center, right or add space between items.
Several values can explain the CSS justify-content property, including the following:
-
flex-start value
: This is the default value of the CSS justify-content property, and it shows the alignment of the flex items at the beginning of the container, as shown below:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
justify-content: flex-start;
background-color: Purple;
width: 800px;
}
.flex-container > div {
background-color: Orange;
width: 60px;
font-size: 20px;
height: 50px;
padding: 20px;
margin: 20px;
}
</style>
</head>
<body>
<h1>Justify Content</h1>
<p>Justify-content flex-start value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:

-
flex-end value
: This value shows the alignment of the flex items at the end of the container, like this:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
justify-content: flex-end;
background-color: Purple;
width: 800px;
}
.flex-container > div {
background-color: Orange;
width: 60px;
font-size: 20px;
height: 50px;
padding: 20px;
margin: 20px
}
</style>
</head>
<body>
<h1>Justify Content</h1>
<p>Justify-content flex-end value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:
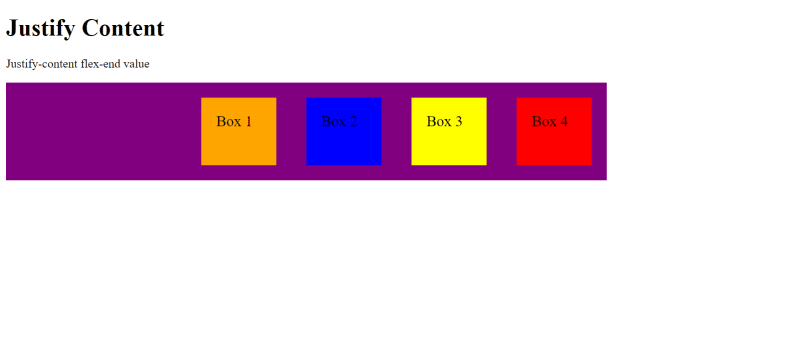
-
center value
: This value aligns the flex items at the center of the container:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
justify-content: center;
background-color: Purple;
width: 800px;
}
.flex-container > div {
background-color: Orange;
width: 60px;
font-size: 20px;
height: 50px;
padding: 20px;
margin: 20px;
}
</style>
</head>
<body>
<h1>Justify Content</h1>
<p>Justify-content center value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:

-
space-around value
: This value shows the flex items with space before, between, and after the lines, as seen in this example:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
justify-content: space-around;
background-color: Purple;
width: 800px;
}
.flex-container > div {
` background-color: Orange;
width: 60px;
font-size: 20px;
height: 50px;
padding: 20px;
margin: 20px
}
</style>
</head>
<body>
<h1>Justify Content</h1>
<p>Justify-content space-around value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:
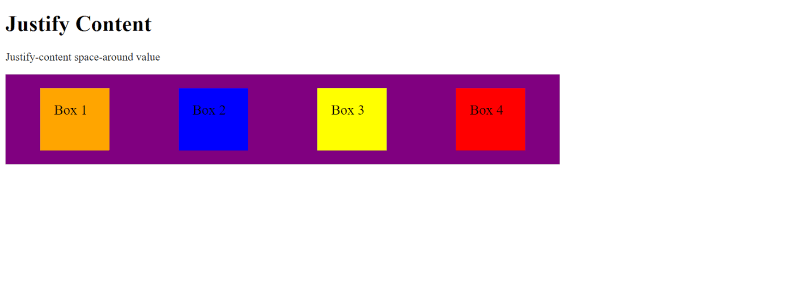
-
space-between value
: This value shows the flex items with space between the lines:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
justify-content: space-between;
background-color: Purple;
width: 800px;
}
.flex-container > div {
background-color: Orange;
width: 60px;
font-size: 20px;
height: 50px;
padding: 20px;
margin: 20px
}
</style>
</head>
<body>
<h1>Justify Content</h1>
<p>Justify-content space-between value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:
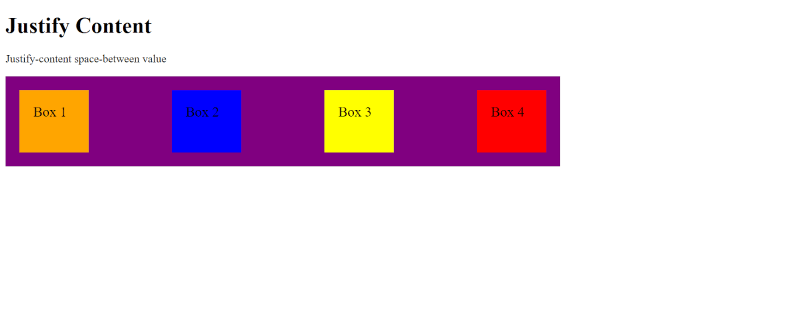
Align Items
The align-items
property aligns the flex items vertically due to the item's inability to use all available space on the cross-axis. Let’s check out examples of different values of the CSS align-item property in order to understand it better:
-
stretch value
: This shows the default value of the CSS align-items property, and it fills the flex container by stretching the flex items, as we can see below:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
align-items: stretch;
background-color: Purple;
height: 300px;
}
.flex-container > div {
background-color: Orange;
width: 80px;
font-size: 20px;
}
</style>
</head>
<body>
<h1>Align items</h1>
<p>Align-items stretch value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:

-
center value
: This value helps to align the flex items in the middle of the container, like this:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
align-items: center;
background-color: Purple;
height: 300px;
}
.flex-container > div {
background-color: Orange;
width: 80px;
font-size: 20px;
height: 50px;
padding: 20px;
margin: 10px
}
</style>
</head>
<body>
<h1>Align items</h1>
<p>Align-items center value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:

-
flex-start value
: This value helps to align the flex items at the top of the container:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
align-items: flex-start;
background-color: Purple;
width: 800px;
height: 300px;
}
.flex-container > div {
background-color: Orange;
width: 60px;
font-size: 20px;
height: 50px;
padding: 20px;
margin: 20px
}
</style>
</head>
<body>
<h1>Align items</h1>
<p>Align-items Flex-start value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:

-
flex-end value
: This value helps to align the flex items at the bottom of the container, as shown here:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
align-items: flex-end;
background-color: Purple;
width: 800px;
height: 300px;
}
.flex-container > div {
background-color: Orange;
width: 60px;
font-size: 20px;
height: 50px;
padding: 20px;
margin: 20px
}
</style>
</head>
<body>
<h1>Align items</h1>
<p>Align-items Flex-end value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code The outcome:

Align Content
The CSS align-content property helps to align the flex lines by applying flex-wrap; it wraps if there are many flex lines. This is similar to align-items, but it aligns lines instead of aligning flex items. The CSS align-content property can have the following values:
-
stretch value
: This value shows the CSS align-content property's default value and involves stretching the flex lines to take up the available space, as in the example below:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
flex-wrap: wrap;
align-content: stretch;
background-color: Purple;
}
.flex-container > div {
background-color: Orange;
width: 80px;
font-size: 20px;
height: 200px;
margin: 20px;
}
</style>
</head>
<body>
<h1>Align content</h1>
<p>Align-content stretch value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:
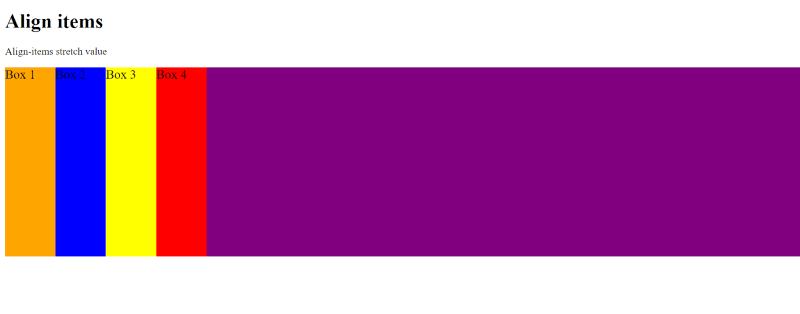
-
space-between value
: This value shows equal space between flex lines:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
flex-wrap: wrap;
align-content: space-between;
background-color: Purple;
width: 200px;
height: 300px;
}
.flex-container > div {
background-color: Orange;
width: 80px;
font-size: 20px;
margin: 10px;
height: 80px;
}
</style>
</head>
<body>
<h1>Align content</h1>
<p>Align-content space-between value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:

-
space-around value
: This value shows space before, between, and after the flex lines. We can see this in the example below:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
flex-wrap: wrap;
align-content: space-around;
background-color: Purple;
width: 200px;
height: 300px;
}
.flex-container > div {
background-color: Orange;
width: 80px;
font-size: 20px;
margin: 10px;
height: 80px;
}
</style>
</head>
<body>
<h1>Align content</h1>
<p>Align-content space-around value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:

-
center value
: This value shows the flex lines in the middle of the container:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
flex-wrap: wrap;
align-content: center;
background-color: Purple;
width: 400px;
height: 300px;
}
.flex-container > div {
background-color: Orange;
width: 80px;
font-size: 20px;
margin: 10px;
height: 80px;
}
</style>
</head>
<body>
<h1>Align content</h1>
<p>Align-content center value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:

-
flex-start value
: This value shows the flex lines at the beginning of the container, as shown below:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
flex-wrap: wrap;
align-content: flex-start;
background-color: Purple;
width: 400px;
height: 300px;
}
.flex-container > div {
background-color: Orange;
width: 80px;
font-size: 20px;
margin: 10px;
height: 80px;
}
</style>
</head>
<body>
<h1>Align content</h1>
<p>Align-content flex-start value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:
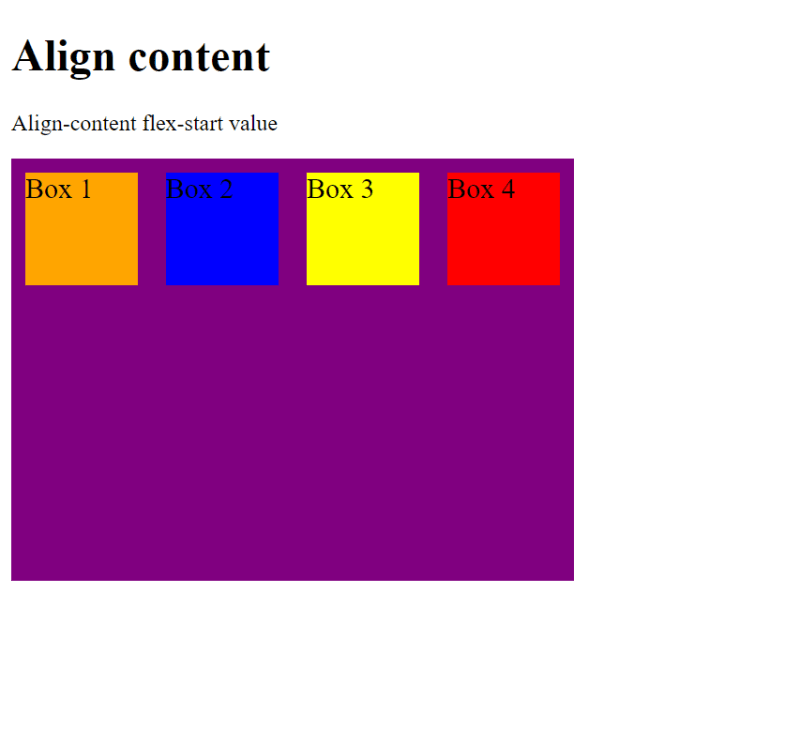
-
flex-end value
: This value shows the flex lines at the end of the container. Here is an example:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
flex-wrap: wrap;
align-content: flex-end;
background-color: Purple;
width: 400px;
height: 300px;
}
.flex-container > div {
background-color: Orange;
width: 80px;
font-size: 20px;
margin: 10px;
height: 80px;
}
</style>
</head>
<body>
<h1>Align content</h1>
<p>Align-content flex-end value</p>
<div class="flex-container">
<div style="background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style="background-color: red">Box 4</div>
</div>
</body>
</html>
Save this code
The outcome:

Flex Item Properties
The CSS flex item has many properties that help explain how flex items are arranged to build layouts. We’ll break down these properties below.
Order Property
The order-flex
property defines the order of the flex items in the same container. The order value must be a number, and there is a preset value of 0. By default, all flex items are arranged in the order of document flow, and when we apply the order property, we do not need to change the HTML structure.
For example, the order property allows us to change the order of the flex items:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
align-items: stretch;
background-color: Purple;
height: 150px;
}
.flex-container > div {
background-color: Orange;
width: 80px;
font-size: 20px;
margin: 20px;
height: 60px;
}
</style>
</head>
<body>
<h1>Flex Item Property</h1>
<h3>Order property</h3>
<div class="flex-container">
<div style="background-color: Orange; order: 3">Box 1</div>
<div style="background-color: blue; order: 4">Box 2</div>
<div style="background-color: yellow; order 2">Box 3</div>
<div style="background-color: red; order: 1">Box 4</div>
</div>
</body>
</html>
The outcome:
Align-self Property
The align-self property defines the alignment for the selected item inside the flexible container. This property overrides the default alignment set by the container's align-items property, as we can see in this example:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
align-items: stretch;
background-color: Purple;
height: 200px;
}
.flex-container > div {
background-color: Orange;
width: 80px;
font-size: 20px;
margin: 20px;
height: 60px;
}
</style>
</head>
<body>
<h1>Flex Item Property</h1>
<h3>Align-self property</h3>
<div class="flex-container">
<div style=" align-self: center; background-color: Orange">Box 1</div>
<div style="background-color: blue">Box 2</div>
<div style="background-color: yellow">Box 3</div>
<div style=" align-self: center; background-color: red">Box 4</div>
</div>
</body>
</html>
The outcome:
Flex-grow Property
The flex-grow property defines the rate a flex item will grow compared to the rest of the flex items in the same container. The value must also be a number with a default value of 0. Flex-grow value of 0 means the flex item's width as per content only. A value of 1 means the flex item's width distribution will be equal:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
align-items: stretch;
background-color: Purple;
height: 150px;
}
.flex-container > div {
background-color: Orange;
width: 80px;
font-size: 20px;
margin: 20px;
height: 60px;
}
</style>
</head>
<body>
<h1>Flex Item Property</h1>
<h3>Flex grow property</h3>
<div class="flex-container">
<div style="background-color: Orange; flex-grow: 1">Box 1</div>
<div style="background-color: blue; flex-grow: 4">Box 2</div>
<div style="background-color: yellow; flex-grow: 1">Box 3</div>
<div style="background-color: red; flex-grow: 1">Box 4</div>
</div>
</body>
</html>
The outcome:
Flex-shrink
The flex-shrink property defines the rate a flex item will shrink compared to the other flex items in the same flex container. The value must be a number with a default value of 1. We can see how this looks below:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
width: 350px;
height: 150px;
border: 1px solid black;
background-color: Purple;
display: flex;
}
.flex-container>div {
background-color: brown;
width: 80px;
font-size: 20px;
margin: 20px;
height: 60px;
}
.flex-container div:nth-of-type(2) {
flex-shrink: 4;
}
.flex-container div:nth-of-type(3) {
flex-shrink: 3;
}
</style>
</head>
<body>
<h1>Flex Item Property</h1>
<h3>Flex shrink property</h3>
<div class="flex-container">
<div class="box 1" style="background-color: Orange;">Box 1</div>
<div class="box 2" style="background-color: blue;">Box 2</div>
<div class="box 3" style="background-color: yellow;">Box 3</div>
<div class="box 4" style="background-color: red;">Box 4</div>
</div>
</body>
</html>
The outcome:
Flex-basis
The flex-basis property defines the initial length or width of a flex item. The default value of the flex-basis property is auto. In addition, for the percentage we need, we’ll assign flex-shrink. For example, 30% means the width of the item will be 30%, and the sum of the widths of other items will be 70%, as shown below:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-container {
display: flex;
align-items: stretch;
background-color: Purple;
}
.flex-container>div {
background-color: brown;
width: 80px;
font-size: 20px;
margin: 20px;
height: 60px;
}
</style>
</head>
<body>
<h1>Flex Item Property</h1>
<h3>Flex basis property</h3>
<div class="flex-container">
<div class="box 1" style="background-color: Orange;">Box 1</div>
<div class="box 2" style="flex-basis: 300px; background-color: blue;">Box 2</div>
<div class="box 3" style="background-color: yellow;">Box 3</div>
<div class="box 4" style="background-color: red;">Box 4</div>
</div>
</body>
</html>
The outcome:
Conclusion
CSS Flexbox allows us to build layouts on our web pages in many spectacular and flexible ways. In this article, you’ve learned a variety of ways that you can create layouts using this CSS flexbox cheat sheet. So, flex your development muscles with all these flexible ways of building responsive layouts on your websites!
Top comments (0)