What is scope?
Scope is all about code accessibility. It determines which parts of the code are accessible and which parts are inaccessible. In this blog you'll learn about how the scope chain works within JavaScript. You will also explore some of the different scope types, such as global and local.
Global Scope: The code that exists outside of a function is referred to as global scope, and all
Local Scope: The code inside of a function is known as local scope or function scope. If a variable is defined within a function, then you can say it's scoped to that function. This is also known as local scope.
In the ES5 version of JavaScript, only functions can build local scope. The only way to declare a variable in JavaScript was to use the var keyword. First, you can use it in your code even before it is declared. Also, you can redeclare the same variable when you use VAR.
The ES6 version of JavaScript introduced a new variety of scope known as the block scope. Block scope states that a variable declared in a block of code is only accessible inside that block. All the other code outside of the code block cannot access it. Block scope is built when you declare variables using let or const. The scope of these variables is contained within curly braces. Its syntax is very similar to the var syntax. Only the keyword is replaced.
Let's learn about the var, let, and const variables and the different rules that they are bound to.
1)var
A variable declared with the var keyword can be accessed before initialization as long as the variable is eventually initialized somewhere in our code.
let's try to console log a variable that hasn't been declared or initialized.
Error: Reference Error
If we declare the var variable and don't initialize it then it won't give any error rather it prints "undefined"
Also, var variable can be redeclared
var user ="Neha"
var user = "Sunita"
var user = "Aditi"
console.log(user);
var user
//user will be updated and will take var value
2) let
we CANNOT access a let variable before we declare it
console.log(user);
let user
We CAN'T redeclare a let variable
let user;
let user="Sam" // ERROR
```
But we CAN re-assign it
```javascript
let user="Jerry"
user = "Jane";
console.log(user);
```
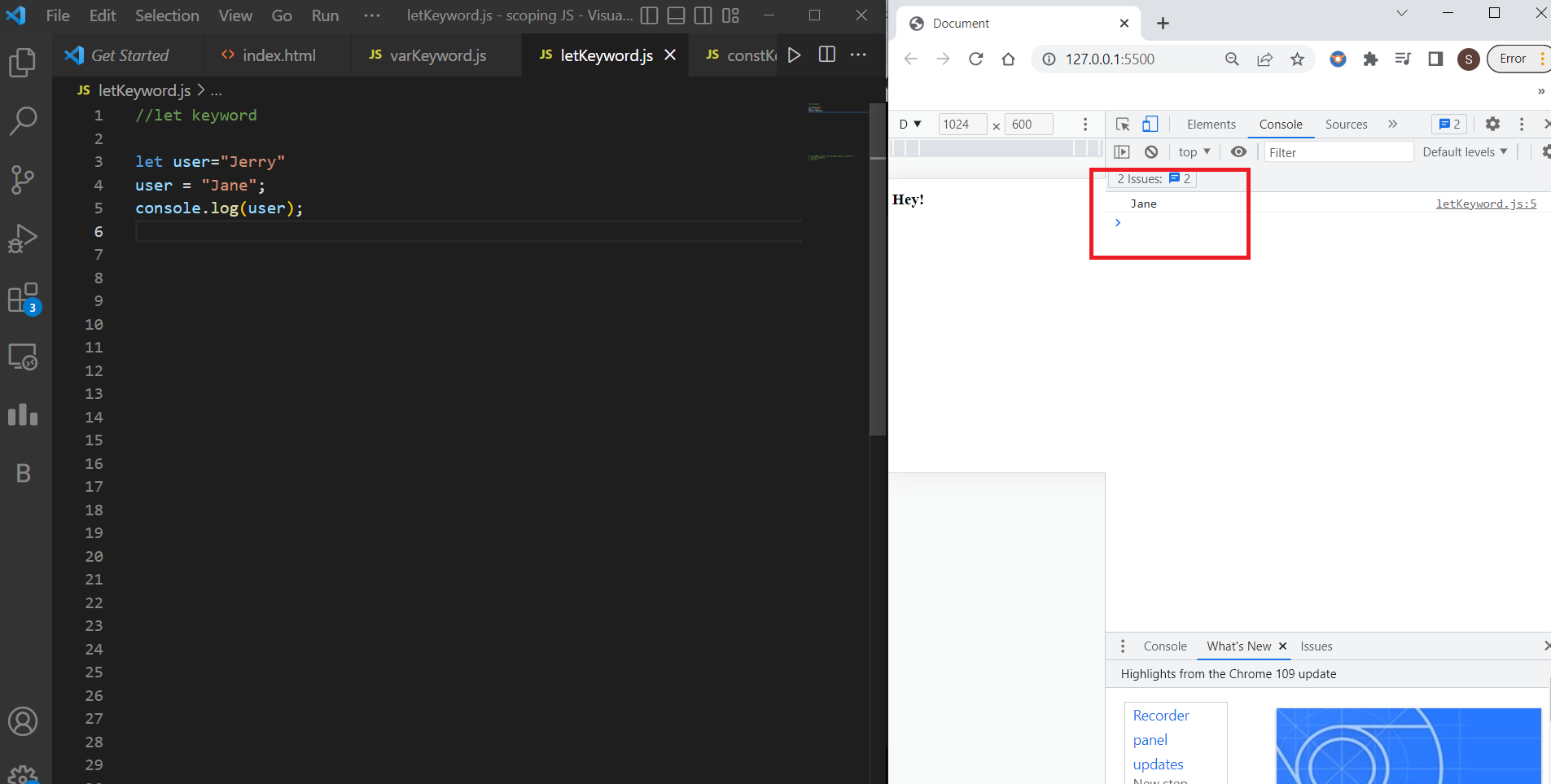
##3)const
we CAN'T access the const variable BEFORE initialization
```javascript
const user;
console.log(user);// ERROR
```
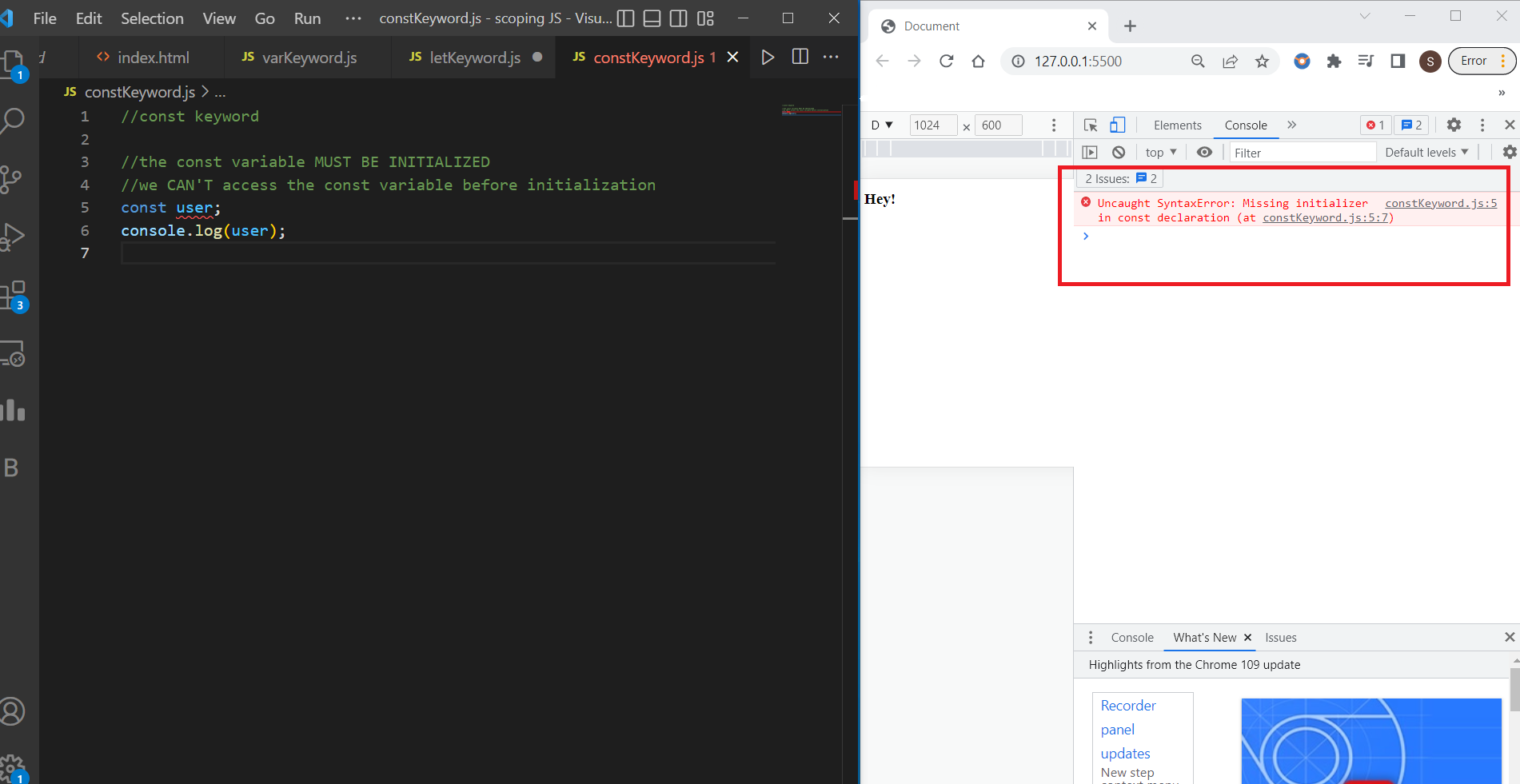
Although, we CAN declare an unassigned variable with let
```javascript
let user;
console.log(user);
```
we CAN'T redeclare a const variable
```javascript
const user="Andrew";
console.log(user);
user = "John"
console.log(user);
```
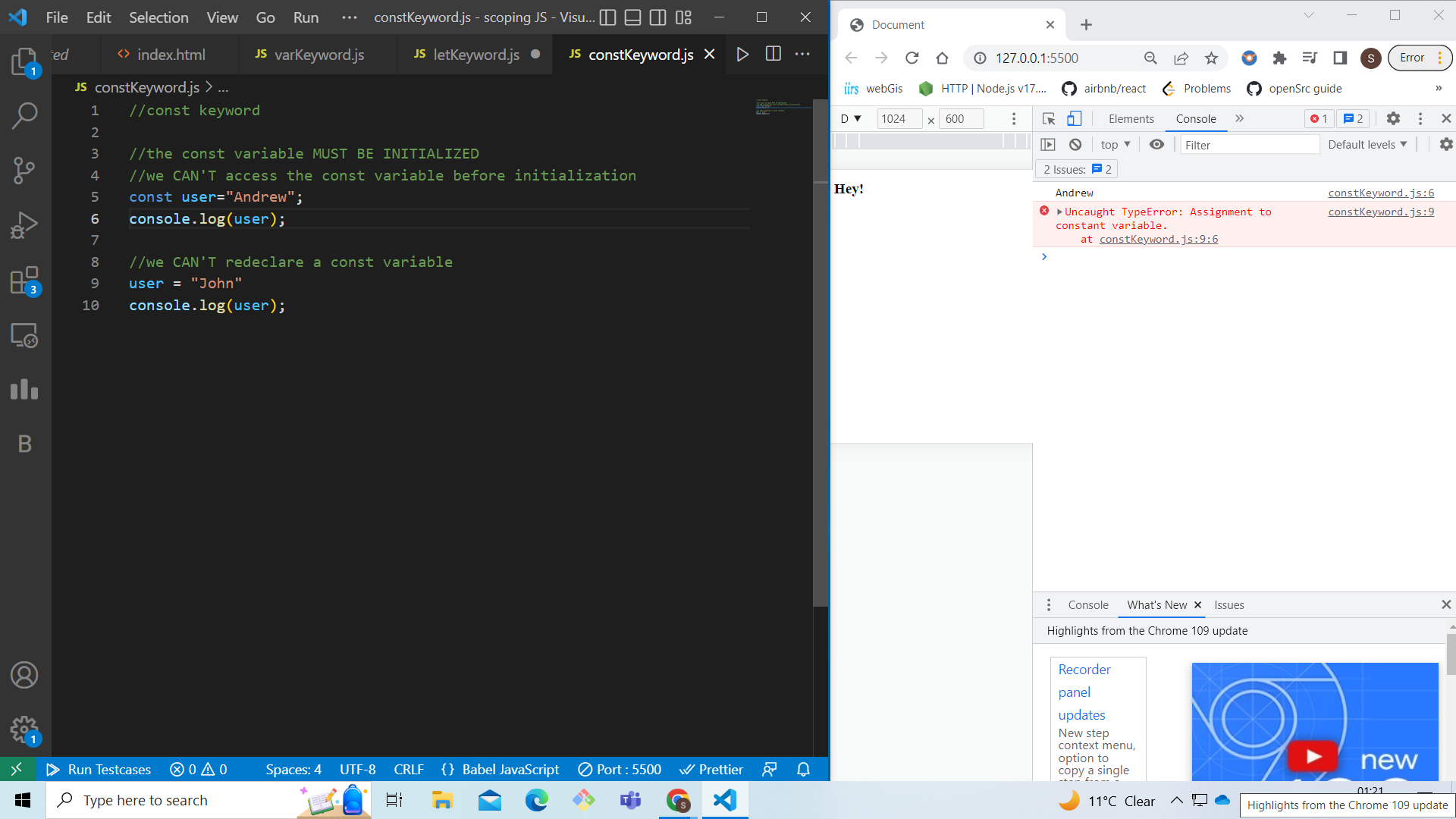
If you have any query let me know in the comment section. I'll try my best to answer them.
If you find this blog helpful, please ❤️ like it.
You can follow me if you wish to enhance your fundamental knowledge of JavaScript
In the coming blogs I'll be covering most asked concepts of JavaScript.
Top comments (0)