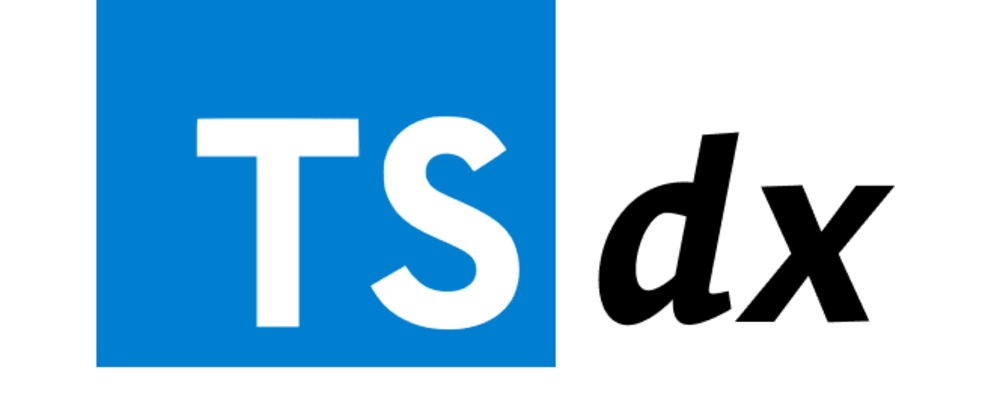
I started my personal hooks library and decided to publish it as an npm package. Doing this in TypeScript was not straightforward, until: palmerhq/...
For further actions, you may consider blocking this person and/or reporting abuse
I will prefer to use
create-react-hook
instead because it will setup anexample
folder that you can deploy to GitHub pages. I once use it for a React hook for manipulating browser cookies.Get, Set, Update and Delete Cookie using React Hooks.
@devhammed/use-cookie
Install
Usage
🎣CLI for easily creating reusable react hooks.
create-react-hook
How and why I made this tool.
Features
cjs
andes
module formatsInstall
This package requires
node >= 4
, but we recommendnode >= 8
.Creating a New Hook
Answer some basic prompts about your module, and then the CLI will perform the following steps:
Development
Local development is broken into two parts (ideally using two tabs).
First, run rollup to watch your
src/
module and automatically recompile it intodist/
whenever you…Awesome! I didn't know create-react-hook. Thank you!
Had the same issue while developing react-virtuoso with tsdx. Came up with a very crude hack, described in this issue.
Ultimately, however, I switched to storybook - it lives inside the project directory and works with the same React instance.
Thank you! I can't believe I haven't tried storybook yet.
Thanks for the article.
I'm dealing with the same issue except it happens even when publicly deployed on npm.
I've been using Parcel but I may give a try to create-react-hook instead