JavaScript is a powerful programming language that allows developers to create dynamic and interactive web applications. However, writing efficient JavaScript code is essential to ensure optimal performance and deliver a smooth user experience. In this article, we will explore the best practices for writing efficient JavaScript code and provide examples to illustrate each practice.
1. Introduction
JavaScript is widely used in web development due to its versatility and ease of use. However, inefficient code can lead to slow page load times, unresponsive user interfaces, and increased resource consumption. By following best practices, developers can optimize their JavaScript code and deliver high-performance applications.
2. Use Proper Variable Declarations
One of the fundamental best practices in JavaScript is to use proper variable declarations. In modern JavaScript, it is recommended to use let and const
instead of the outdated var
keyword. let
allows block scoping, which limits the scope of variables to the nearest enclosing block, while const
ensures that a variable is not reassigned.
For example:
// Good
let count = 0;
const MAX_VALUE = 100;
// Bad
var total = 10;
Using let
and const
not only improves code clarity but also helps prevent accidental variable reassignments and reduces the chances of encountering scope-related issues.
3. Optimize Loops
Loops are commonly used in JavaScript to iterate over arrays, objects, and other data structures. However, inefficient looping can result in performance bottlenecks, especially when dealing with large datasets. To optimize loops, consider the following techniquesTo optimize loops, consider the following techniques:
- Use a for loop instead of a forβ¦in loop when iterating over arrays. The for loop provides better performance and avoids iterating over inherited properties.
// Good
const numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
// Do something with numbers[i]
}
// Bad
const numbers = [1, 2, 3, 4, 5];
for (const index in numbers) {
// Do something with numbers[index]
}
- Cache the array length before the loop to avoid repeatedly accessing the length property.
// Good
const numbers = [1, 2, 3, 4, 5];
const length = numbers.length;
for (let i = 0; i < length; i++) {
// Do something with numbers[i]
}
// Bad
const numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
// Do something with numbers[i]
}
- Consider using array iteration methods like
forEach
,map
,filter
, etc., as they often provide more concise and readable code.
const numbers = [1, 2, 3, 4, 5];
// Good
numbers.forEach((number) => {
// Do something with number
});
// Bad
for (let i = 0; i < numbers.length; i++) {
const number = numbers[i];
// Do something with number
}
By implementing these loop optimization techniques, you can significantly improve the performance of your JavaScript code, especially when dealing with large datasets.
4. Minimize DOM Manipulation
Manipulating the Document Object Model (DOM) can be resource-intensive and impact the performance of your web application. To write efficient JavaScript code, it's important to minimize unnecessary DOM manipulations.
Frequent updates to the DOM can cause layout recalculations and repaints, leading to a sluggish user interface. To mitigate this, consider the following practices:
- Use document fragments when inserting multiple elements into the DOM simultaneously. Document fragments allow you to append multiple elements to the fragment, make modifications, and then append the entire fragment to the DOM in a single operation. This reduces the number of layout recalculations and repaints.
// Create a document fragment
const fragment = document.createDocumentFragment();
// Add elements to the fragment
for (let i = 0; i < 1000; i++) {
const element = document.createElement('div');
element.textContent = 'Element ' + i;
fragment.appendChild(element);
}
// Append the fragment to the DOM
document.getElementById('container').appendChild(fragment);
- Batch DOM updates by making modifications to elements outside the DOM and then appending them to the DOM all at once. This reduces the number of layout recalculations and repaints. ```
// Create and modify elements outside the DOM
const elements = [];
for (let i = 0; i < 1000; i++) {
const element = document.createElement('div');
element.textContent = 'Element ' + i;
elements.push(element);
}
// Append all elements to the DOM at once
const container = document.getElementById('container');
elements.forEach((element) => {
container.appendChild(element);
});
By minimizing unnecessary DOM manipulations, you can optimize the rendering process and improve the overall performance of your JavaScript code.
π¨βπ»π‘π₯ Hey, fellow coders! Want to take your skills to the next level? Check out my latest post where I share some awesome coding tips and tricks that will blow your mind! π₯π» Don't forget to hit the β€οΈ button if you found it helpful, drop a comment with your favorite coding tip, and share it with your friends who are also into coding. Let's spread the knowledge and make the coding community even stronger! πͺπ€
[
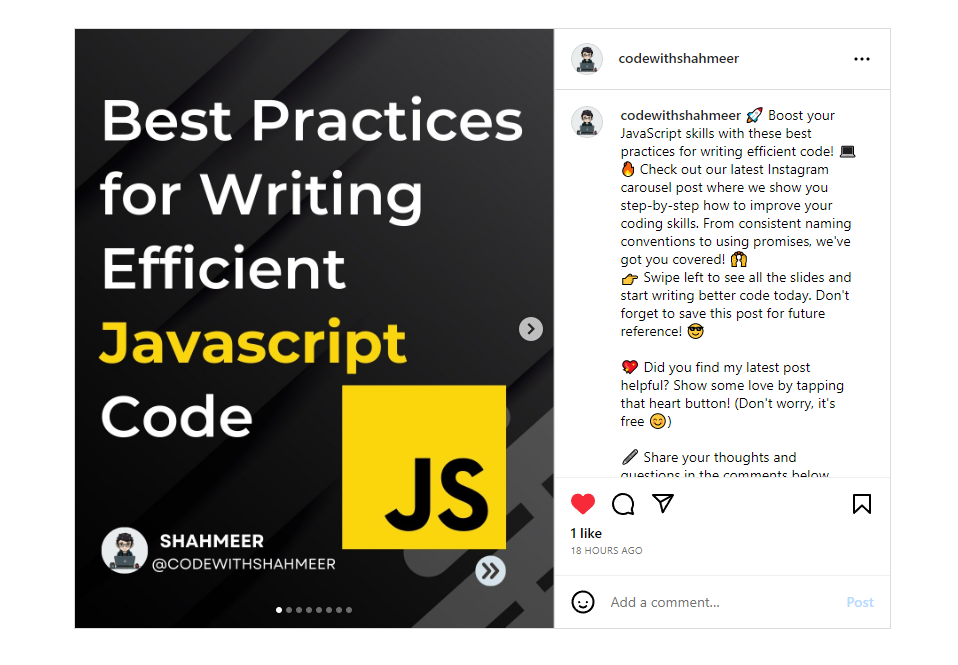](https://www.instagram.com/p/CsJIvIvow86/)
##5. Avoid Synchronous Operations
In JavaScript, synchronous operations can block the execution of other code until they are completed, resulting in poor performance and a less responsive user interface. It is crucial to avoid synchronous operations whenever possible and utilize asynchronous functions and callbacks instead.
Asynchronous operations allow the code to continue executing while waiting for tasks to complete, ensuring that the application remains responsive. Here are some practices to follow:
- Use asynchronous APIs and functions provided by JavaScript and browser APIs, such as setTimeout, setInterval, and fetch. These APIs allow you to perform tasks asynchronously, preventing blocking operations.
// Bad: Synchronous operation
const result = expensiveSyncOperation();
// Good: Asynchronous operation
expensiveAsyncOperation((result) => {
// Handle the result asynchronously
});
- Utilize Promises and `async/await` syntax for managing asynchronous operations. Promises provide a clean and structured way to handle asynchronous code, while `async/await` simplifies the syntax and improves readability.
// Using Promises
fetch(url)
.then((response) => response.json())
.then((data) => {
// Handle the data asynchronously
})
.catch((error) => {
// Handle errors
});
// Using async/await
try {
const response = await fetch(url);
const data = await response.json();
// Handle the data asynchronously
} catch (error) {
// Handle errors
}
By embracing asynchronous operations and avoiding synchronous code, you can ensure that your JavaScript code performs efficiently and keeps the user interface responsive.
##6. Optimize Event Handlers
Event handlers play a crucial role in web applications, but inefficient handling of events can impact performance. To optimize event handling in JavaScript, consider the following practices:
- Implement event delegation, which involves attaching a single event handler to a parent element instead of multiple handlers to individual child elements. This technique reduces the number of event listeners and improves performance, especially for dynamically generated or large numbers of elements.javascriptCopycode
// Bad: Individual event handlers for each element
const buttons = document.querySelectorAll('.button');
buttons.forEach((button) => {
button.addEventListener('click', (event) => {
// Handle the click event
});
});
// Good: Event delegation on a parent element
const parent = document.getElementById('parent');
parent.addEventListener('click', (event) => {
if (event.target.classList.contains('button')) {
// Handle the click event
}
});
- Apply throttling or debouncing techniques to limit the frequency of executing event handlers, especially for events like `scroll `or `resize `that can trigger frequently. Throttling restricts the execution to a fixed interval, while debouncing postpones the execution until a certain period of inactivity
// Throttling example
function throttle(callback, delay) {
let lastExecutionTime = 0;
return function () {
const currentTime = Date.now();
if (currentTime - lastExecutionTime > delay) {
callback.apply(this, arguments);
lastExecutionTime = currentTime;
}
};
}
window.addEventListener(
'scroll',
throttle(function () {
// Handle the scroll event with throttling
}, 200)
);
Optimizing event handlers ensures efficient handling of user interactions and contributes to a smoother user experience in JavaScript applications.
##7. Use Efficient Data Structures and Algorithms
Choosing the right data structures and algorithms can have a significant impact on the performance of your JavaScript code. By selecting efficient options, you can optimize operations and improve overall execution speed. Here are some practices to follow:
Utilize appropriate data structures based on the requirements of your code. For example, use arrays for indexed access or stacks for last-in-first-out (LIFO) operations. Use objects or maps for key-value pair lookups or sets for storing unique values.
// Good: Using an array for indexed access
const numbers = [1, 2, 3, 4, 5];
const thirdNumber = numbers[2];
// Good: Using a map for key-value pair lookups
const userMap = new Map();
userMap.set('John', { age: 30, email: 'john@example.com' });
const john = userMap.get('John');
- Implement efficient algorithms for common tasks, such as searching, sorting, and manipulating data. For example, use binary search for sorted arrays, employ efficient sorting algorithms like quicksort or mergesort, and optimize data manipulation operations to minimize unnecessary iterations.
// Good: Using binary search for efficient searching
function binarySearch(array, target) {
let start = 0;
let end = array.length - 1;
while (start <= end) {
const mid = Math.floor((start + end) / 2);
if (array[mid] === target) {
return mid;
} else if (array[mid] < target) {
start = mid + 1;
} else {
end = mid - 1;
}
}
return -1;
}
By leveraging efficient data structures and algorithms, you can optimize the execution speed and resource utilization of your JavaScript code.
##8. Minify and Bundle JavaScript Files
Minifying and bundling JavaScript files is a common practice to optimize performance and reduce file sizes. Minification involves removing unnecessary characters (whitespace, comments, etc.) from the code, while bundling combines multiple JavaScript files into a single file.
- Use tools like UglifyJS or Terser to minify your JavaScript code. Minification reduces file sizes, which results in faster downloads and improved page load times.
- Employ bundling tools like Webpack or Rollup to combine multiple JavaScript files into a single bundle. Bundling reduces the number of HTTP requests, enhances caching, and optimizes resource loading.
By minifying and bundling your JavaScript files, you can improve the efficiency and speed of your web application.
##9. Avoid Global Variables
Global variables can lead to naming conflicts, increase memory usage, and make code harder to maintain. To write efficient JavaScript code, minimize the use of global variables and embrace encapsulation and modularization.
- Wrap your code in immediately invoked function expressions (IIFE) or utilize modules to create private scopes for variables and functions.
// Using an IIFE to encapsulate code
(function () {
// Variables and functions here are within the IIFE scope
const privateVariable = 10;
function privateFunction() {
// Code here is only accessible within the IIFE
}
// Expose public API if needed
window.myModule = {
publicFunction() {
// Publicly accessible code
},
};
})();
- Leverage module systems like CommonJS or ES modules (import/export) to organize your code into reusable and maintainable modules. This helps avoid polluting the global namespace and promotes better code organization.
// CommonJS module
// math.js
const add = (a, b) => a + b;
const subtract = (a, b) => a - b;
module.exports = { add, subtract };
// main.js
const { add, subtract } = require('./math.js');
console.log(add(5, 3)); // Output: 8
console.log(subtract(7, 2)); // Output: 5
- When necessary, use object namespaces or classes to encapsulate related variables and functions within a specific context.javascriptCopy code
// Using object namespace
const myApp = {
counter: 0,
increment() {
this.counter++;
},
reset() {
this.counter = 0;
},
};
myApp.increment();
console.log(myApp.counter); // Output: 1
By avoiding global variables and embracing encapsulation and modularization techniques, you can write cleaner, more maintainable, and efficient JavaScript code.
##10. Handle Errors Properly
Proper error handling is essential for writing efficient and robust JavaScript code. By handling errors effectively, you can prevent application crashes, improve user experience, and facilitate debugging. Consider the following practices:
- Use try-catch blocks to catch and handle exceptions. Wrap potentially error-prone code within try blocks and provide appropriate error handling in catch blocks
try {
// Potentially error-prone code
const result = calculateSomething();
console.log(result);
} catch (error) {
// Handle the error
console.error('An error occurred:', error);
}
- Implement proper error logging to gather valuable information about errors occurring in your application. Use logging libraries or custom error logging mechanisms to track and analyze errors.
function logError(error) {
// Send the error to a logging service or perform custom logging
console.error('Error:', error);
}
try {
// Code that may throw an error
} catch (error) {
logError(error);
}
By handling errors properly, you can ensure that your JavaScript code gracefully handles unexpected situations and provides a better user experience.
##11. Optimize Network Requests
Efficiently managing network requests is crucial for improving the performance of web applications. Slow or excessive requests can result in poor user experience and increased server load. Consider the following practices for optimizing network requests:
- Minimize the number of requests by bundling or combining resources whenever possible. This reduces the overhead of multiple round trips to the server.
- Implement caching mechanisms to store and reuse server responses. Leverage browser caching or utilize techniques like HTTP caching headers to reduce redundant requests for static or infrequently changing resources.
- Compress and minimize the size of transferred data by enabling compression techniques like gzip or Brotli. Smaller file sizes result in faster downloads and improved performance.
- Use asynchronous requests, such as AJAX or Fetch API, to avoid blocking the main thread and ensure a smooth user experience. Asynchronous requests allow the page to continue rendering while data is being fetched from the server.
- Employ lazy loading techniques for resources like images or scripts that are not immediately visible or required. Load these resources only when they become necessary, reducing the initial page load time.
By optimizing network requests, you can significantly enhance the performance and responsiveness of your JavaScript applications.
##12. Optimize CSS and JavaScript Rendering
Efficient rendering of CSS and JavaScript plays a crucial role in delivering a smooth user experience. Inefficient rendering can lead to rendering delays, layout shifts, and poor performance. Consider the following practices to optimize rendering:
- Avoid render-blocking CSS and JavaScript that prevent the rendering of the page content. Move non-critical CSS and JavaScript to the bottom of the page or load them asynchronously.
- Utilize techniques like lazy loading or asynchronous loading for JavaScript and CSS files. Load these resources when they are needed rather than upfront, reducing the initial page load time.
- Optimize CSS selectors to avoid unnecessary specificity and improve selector matching performance. Use classes or data attributes instead of complex selectors whenever possible.
/* Bad: Overly specific selector */
ul#myList li.active {
color: red;
}
/* Good: Simplified selector */
.myList-item.active {
color: red;
}
- Minify and compress CSS and JavaScript files to reduce their file size. Minification removes unnecessary characters like whitespace and comments, while compression techniques like gzip or Brotli further reduce the file size for faster downloads.
- Utilize techniques like CSS sprites or image optimization to reduce the number of HTTP requests for images. Combine multiple images into a single sprite sheet or use optimized image formats to minimize file sizes.
- Employ techniques like caching and browser storage (e.g., local storage or session storage) to store and reuse rendered CSS and JavaScript resources. This reduces the need for repeated rendering and improves performance.
By optimizing the rendering of CSS and JavaScript, you can enhance the loading speed and overall performance of your web pages.
##13. Use Efficient Libraries and Frameworks
Choosing efficient and lightweight libraries and frameworks can significantly impact the performance of your JavaScript code. Here are some practices to consider:
- Evaluate the size and performance characteristics of libraries and frameworks before incorporating them into your project. Choose smaller and optimized options whenever possible.
- Consider using modern frameworks that offer performance optimizations out of the box, such as React or Vue.js. These frameworks implement virtual DOM diffing and efficient rendering techniques to minimize unnecessary updates.
- Opt for specialized libraries that provide specific functionality instead of larger, all-in-one solutions. This reduces the overhead of unused features and improves performance.
- Regularly update your libraries and frameworks to the latest versions to benefit from bug fixes, performance enhancements, and new features.
Remember to benchmark and test different libraries and frameworks to ensure they meet your performance requirements before integrating them into your project.
##14. Profile and Benchmark Your Code
Profiling and benchmarking your JavaScript code are essential steps in identifying performance bottlenecks and optimizing your application. Here's how you can do it:
- Utilize browser developer tools like Chrome DevTools to profile your code and identify areas that consume excessive resources or cause performance issues. Use the Performance and Memory tabs to analyze and optimize your code.
- Benchmark critical sections of your code to measure their execution time and identify areas that require optimization. Use tools like Lighthouse or custom benchmarking scripts to assess the performance of your code.
- Optimize the identified bottlenecks based on the profiling and benchmarking results. Apply the previously mentioned best practices specific to the identified areas to enhance the overall performance.
By profiling and benchmarking your code, you can uncover performance issues, optimize critical sections, and achieve better JavaScript code efficiency.
##15. Conclusion
Writing efficient JavaScript code is crucial for delivering high-performance web applications. By following the best practices outlined in this article, such as using proper variable declarations, optimizing loops, minimizing DOM manipulation, and employing efficient data structures and algorithms, you can improve the speed, responsiveness, and overall efficiency of your JavaScript code.
Remember to handle errors properly, optimize network requests, optimize CSS and JavaScript rendering, use efficient libraries and frameworks, and profile and benchmark your code to further enhance its performance.
By incorporating these best practices into your development workflow, you can write JavaScript code that is not only efficient but also scalable, maintainable, and optimized for the best possible user experience.
###FAQs
Q1: How can I measure the performance of my JavaScript code?
A: To measure the performance of your JavaScript code, you can utilize browser developer tools like Chrome DevTools. The Performance tab allows you to record and analyze the execution timeof your code, identify bottlenecks, and optimize performance. Additionally, you can use tools like Lighthouse, which provides performance auditing and suggestions for improving your web application's performance.
Q2: What are some tools for minifying JavaScript code?
A: There are several tools available for minifying JavaScript code. Some popular ones include UglifyJS, Terser, and Closure Compiler. These tools remove unnecessary characters, such as whitespace and comments, to reduce the file size of your JavaScript code.
Q3: How can I optimize network requests in my JavaScript code?
A: To optimize network requests, you can follow various practices. Some key ones include minimizing the number of requests by bundling or combining resources, implementing caching mechanisms to reuse server responses, compressing data with techniques like gzip or Brotli, and utilizing asynchronous requests like AJAX or Fetch API to prevent blocking the main thread.
Q4: How can I handle errors effectively in my JavaScript code?
A: Handling errors properly is crucial for maintaining a robust JavaScript codebase. One approach is to use try-catch blocks to catch and handle exceptions. Additionally, implementing proper error logging allows you to gather valuable information about errors occurring in your application, aiding in debugging and issue resolution.
Q5: Is it important to update libraries and frameworks regularly?
A: Yes, regularly updating your libraries and frameworks is important. Updates often include bug fixes, performance enhancements, and new features, which can improve the efficiency and functionality of your code. It is advisable to review changelogs, follow best practices for updating, and test your code after updating to ensure compatibility and performance.
π Get ready for some mind-blowing coding tips and tricks! π»π‘ In my latest post, I've condensed some awesome programming hacks into a concise read. Whether you're a coding newbie or a seasoned pro, you won't want to miss this! π€
So, head over to my social media accounts and enjoy the exciting content. Don't forget to like, share, and follow for more incredible updates! ππ
Check out my latest post! πβ¨
π· Instagram | π₯ YouTube | π΅ TikTok | πΌ LinkedIn | π» GitHub |
π Medium
Top comments (1)
While I agree with most of what you're saying, in point 4:
This does not add all the elements to the DOM at once, it adds them one at a time! If you want to do it all at once, create a container element, add the new elements as children of that element, then add the container to the DOM.
And in point 12:
You wouldn't write a selector like that. Ideally, you'd use
li.active
and have consistent behaviour of all list items on your site. If you need to have them behave differently in one specific place, then I'd have words with your designer first-off, but then I'd allow a specific class on the exception. That's what the cascade is for!