Prerequisite
This article aims to show the reader how to validate a simple form using regular expressions(regex) in JavaScript. To understand the contents of this article, the reader must have basic knowledge of HTML, CSS and JavaScript.
Table of Content
- Introduction
- What is a regular expression?
- How to create regular expressions(regex) in JavaScript
- Common regex patterns
- Building a form
- Validating the username
- Validating the password
- Conclusion
Introduction
As a developer, when building HTML forms, you may want to ensure that the value the user inputs into the form matches your preferred pattern. For example, a form may contain a field for the user to input a password. You may want the password to contain only lowercase letters, and numbers but no hyphens. You can create a pattern and then test it against the value inputted by the user. The easiest way to do this is to use matching patterns known as regular expressions(regex). By the end of this article, you will understand what regular expressions are, their syntax and how to use them by building a login form and validating the input fields with regular expressions.
What are regular expressions(regex)?
Regular expressions in JavaScript are sequences of characters used to create patterns. These matching patterns are then tested against a combination of characters in a string. For example, regex patterns can be used to check strings like emails or passwords to determine if their character combinations match the regex patterns defined. Regular expressions are objects. This means that they inevitably have methods. Regular expressions are not unique to JavaScript, they form part of other programming languages like Python and Java.
How to create regular expressions in JavaScript
There are two ways of creating regular expressions in JavaScript. They can be created using a constructor function or by using forward slashes (/
).
-
Regular expression constructor
Syntax:
new RegExp(pattern, flag)
Example
const str = 'JavaScript'
const regex = new RegExp('s' 'i');
console.log(regex.test(str)); // true
The above example tests whether the regex pattern s
with a flag of i
which means case insensitive matches any character in the string inside the str
variable. The test()
method returns a boolean true
because there is a match.
-
Regular expression literal
Syntax:
/pattern/flag
```
const text = "JavaScript";
const regPattern = /[a-z\d]/i;
console.log(regPattern.test(text));
The `regPattern` variable has a pattern that tests for letters from a to z as well as any numbers. It also has a flag of `i` which means case insensitive.
### Common regex patterns
Here are common regular expression(regex) patterns:
- **Telephone number validation pattern**
Regex pattern:
/^\d{11}$/
or
/^[0-9]{11}$/
The pattern above matches any number from 0-9 at the beginning of the input and the end of the input. The pattern is also specified to be eleven numbers long.
The `^` and `$` characters are known as an *assertion*. The former is used to signify the beginning of an input and the latter is used to match the end of an input.
- **Username validation pattern**
Regex pattern:
/^[a-z\d]{5,12}$/i
The pattern above matches any case-insensitive letter from a-z and any number from 0-9. The pattern is also specified to be between five and twelve characters long. The `i` character is a flag. The flag means that the letters should be case-insensitive.
- **Password validation pattern**
Regex pattern:
/^[\w@-]{8,20}$/
The pattern above matches any letter which is case insensitive, any number from 0-9, an underscore, an @ symbol or a hyphen. The pattern is specified to be between 8 and 20 characters long. The `\w` character simply means any capital or small letter, any number and an underscore.
The above patterns can be modified depending on what you want. If you want to delve deeper into regex patterns and syntax, you can check out the MDN docs: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Regular_expressions
### Building the form
- **Setting up**
Open your Integrated Development Environment(IDE). Ideally, you should have VS code installed. Open a new folder and give it whatever name you want. After doing that, create a new file, an index.html file and a style.css file. if you are familiar with using tailwindcss, then install it. For information on how to install tailwindcss in your IDE, visit the tailwind docs: https://tailwindcss.com/docs/installation.
For this tutorial, I will be using tailwindcss since it is faster and easier to use.
- **The HTML code**
After setting up, your HTML code will look something like this(of course you can write your HTML anyhow you like):
<!DOCTYPE html>
Document
Login Form
Login Form
Username
username valid!
type="text"
name="username"
id="username"
placeholder="username"
/>
username invalid
Password
password valid!
type="password"
name="password"
id="password"
placeholder="password"
/>
password invalid!
Sign In
Forgot Password?
Output:
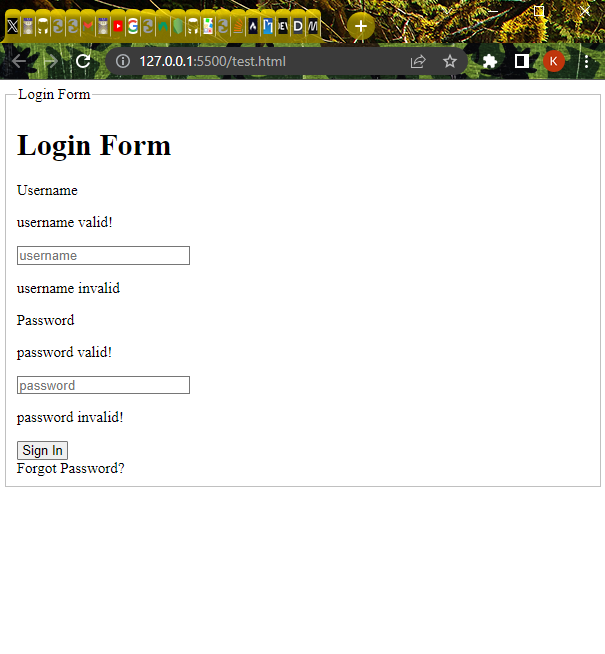
If you are using tailwindcss, you can take advantage of the plugin for forms. Open a terminal in your VS code and install the plugin form:
npm install -D @tailwindcss/forms
After doing that, add the plugin to your `tailwind.config.js` file:
// tailwind.config.js
module.exports = {
theme: {
// ...
},
plugins: [
require('@tailwindcss/forms'),
],
}
- **Adding CSS to the HTML**
<!DOCTYPE html>
TECHNICAL WRITING
class="grid sm:border-2 justify-center px-6 pb-20 pt-10 bg-gray-100 sm:bg-white sm:px-10 sm:rounded-md sm:shadow-md"
>
Login Form
class="sm:grid sm:justify-center sm:mb-10 hidden font-bold text-lg"
>
Login Form
Username
username valid!
<input
type="text"
name="username"
id="username"
placeholder="username"
class="form-input px-4 py-3 rounded-md bg-gray-300"
/>
<p class="text-red-700 hidden" id="userfeed1">
username invalid
</p>
</div>
<div class="py-2 grid" id="Pword">
<label class="text-lg font-semibold">Password</label>
<p class="text-blue-500 hidden" id="passwordfeed">
password valid!
</p>
<input
type="password"
name="password"
id="password"
placeholder="password"
class="form-input px-4 py-3 rounded-md bg-gray-300"
/>
<p class="text-red-500 hidden" id="passwordfeed1">
password invalid!
</p>
</div>
</div>
<div class="flex gap-8 mt-5">
<div>
<button
class="border-2 bg-blue-600 text-white p-1 hover:bg-slate-600"
>
Sign In
</button>
</div>
<div>
<a href="#" class="text-blue-500 hover:text-blue-800"
>Forgot Password?</a
>
</div>
</div>
</fieldset>
</form>
</div>
</div>
<script src="index.js"></script>
The result will look like this:
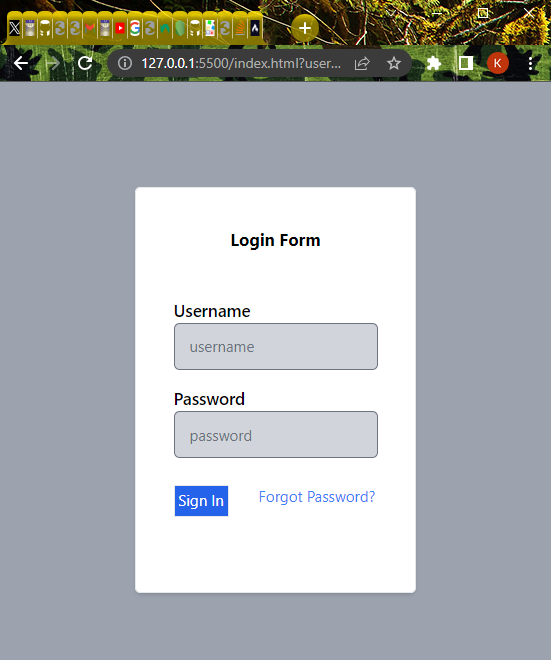
or if you are using a mobile phone like this:
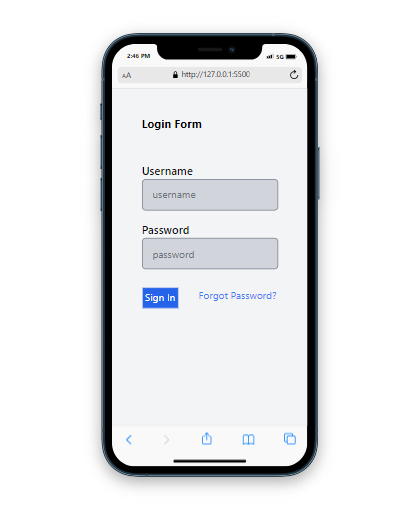
### Validating the username with regex
Firstly, you will create an `index.js` file and inside the file, you will reference all the elements you will need:
"use script";
const form = document.querySelector("#form");
const username = document.querySelector("#username");
const password = document.querySelector("#password");
const userfeed = document.querySelector("#userfeed");
const userfeed1 = document.querySelector("#userfeed1");
const passwordfeed = document.querySelector("#passwordfeed");
const passwordfeed1 = document.querySelector("#passwordfeed1");
Then we will go ahead and create an object called `pattern`. This object will store the patterns for the username and password. We want the username to contain case-insensitive letters, and numbers and the username must be between 5-12 characters long.
const pattern = {
username: /^[a-z\d]{5,12}$/i,
};
Once that is done, we will go ahead and create a function that validates and checks if the input by the user matches the regex pattern that we want:
const validatingFunc = function (idvalid, idinvalid, field, fieldName) {
if (pattern[fieldName].test(field.value)) {
userfeed.classList.add("hidden");
passwordfeed.classList.add("hidden");
idvalid.classList.remove("hidden");
idinvalid.classList.add("hidden");
} else if (field.value === "") {
idinvalid.classList.add("hidden");
idvalid.classList.add("hidden");
} else {
userfeed.classList.add("hidden");
passwordfeed.classList.add("hidden");
idinvalid.classList.remove("hidden");
idvalid.classList.add("hidden");
}
};
The function above takes in four parameters; `idvalid`, `idinvalid`, `field`, `fieldName`. The first two parameters refer to the `id` of elements that are hidden from the DOM. The `field` parameter refers to the element that has an `addEventListener()` function attached to it when an event is triggered. The `fieldName` parameter refers to the name of the element. This name is then used to access the name of the property that contains the pattern.
The function is all set but how do we know it is working? well, we attach an `addEventListener()` function to the input fields which will listen for a `keyup` event. The `validatingFunc()` will be called inside the `addEventListener()` function.
username.addEventListener("keyup", function (e) {
validatingFunc(userfeed, userfeed1, e.target, e.target.attributes.name.value);
});
When we were referencing the elements, we referenced elements with a tag name of `p`. These elements were hidden from the DOM but the `validatingFunc()` makes them visible depending on whether the pattern entered by the user is *username valid* or *username invalid*. Once you type into the username input field, a *username invalid* message appears as long as the pattern is not a match but once it is a match, a *username valid* message appears.
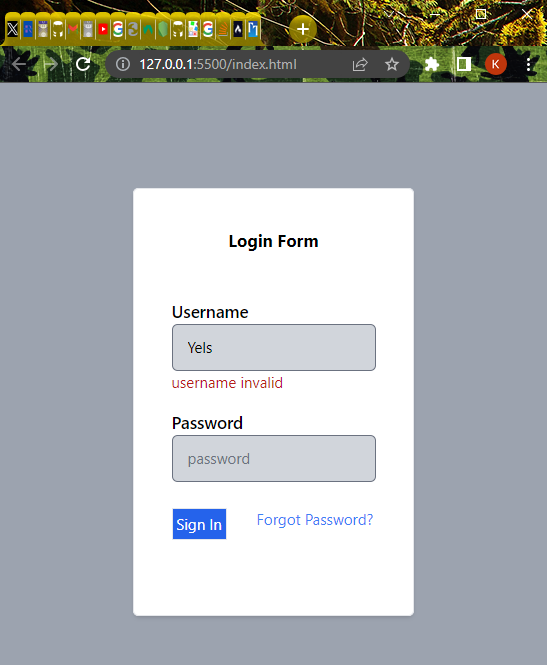
The above username is not a match because it is not at least 5 characters long. Under the input field, a message of _username invalid_ appears.
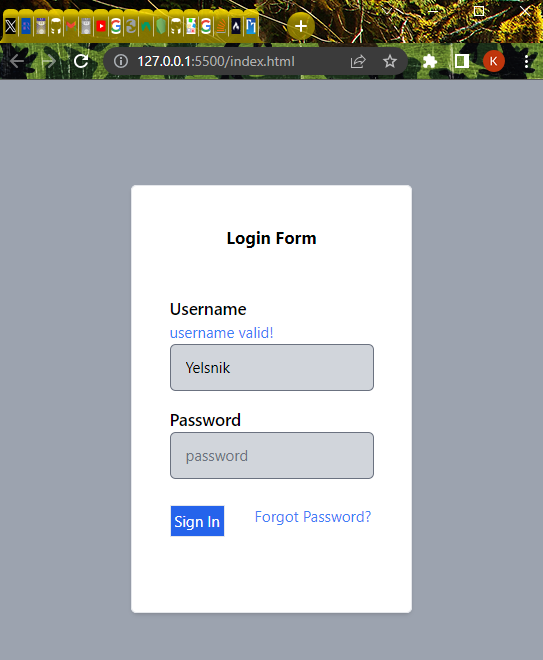
The username matches the pattern and a message of username valid appears above the input field.
### Validating the password
You have created and tested a regex pattern for the username now it is time to do the same for the password. It is not difficult to do this once you have validated the username. All you need to do is create a pattern for the password and include it inside the `pattern` object.
const pattern = {
username: /^[a-z\d]{5,12}$/i,
password: /^[\w@-]{8,20}$/,
};
We want our password to contain letters that are case-insensitive, numbers, underscores, an @ symbol and a hyphen. The password must be between 8 and 20 characters long. The `\w` character signifies any letter, number or underscore. The letters are case insensitive.
After creating the regex pattern, we will attach an `addEventListener()` function to the password input field. We had earlier referenced this input field with the `id` name `password`. The `validatingFunc()` will be called inside the `addEventListener()` function.
password.addEventListener("keyup", function (e) {
validatingFunc(
passwordfeed,
passwordfeed1,
e.target,
e.target.attributes.name.value
);
});
Of course, after this is done, we want to make sure it works so we will input a value into the password input field.
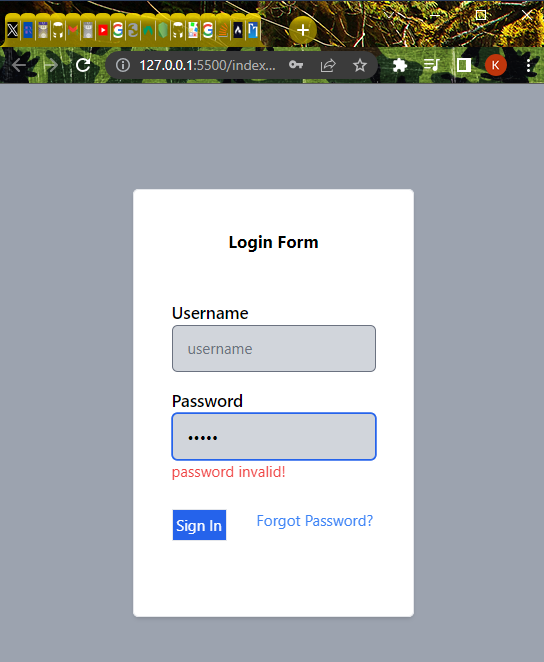
The password we input does not match the regex pattern we created so the message *password invalid* appears below the input field.
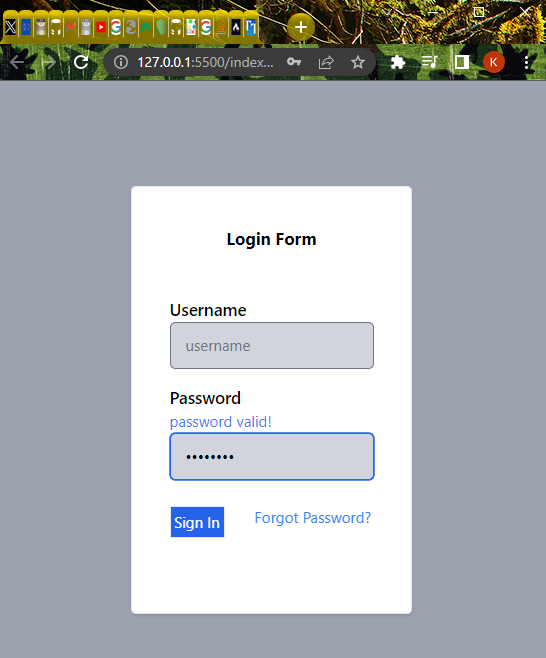
The password matches the regex pattern we earlier created so the message *password valid* appears above the input field.
### Conclusion
In summary, this article has provided background knowledge on regular expressions in JavaScript, how they are created and common regex patterns. You have also learnt how to test and validate a simple login form using regex in JavaScript. If you want to learn more about regular expressions, you can visit the MDN docs: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Regular_expressions
Top comments (0)