Let's face it, packaging your kivy application for android can be pretty rough. First you create the app, then you install buildozer only to realize that python-for-android doesn't even run on windows! Now you're looking into installing a virtual Linux distribution. If you already use Linux, well lucky you, but if not, you are probably pounding your keyboard in frustration.
Is there a better way to do it? The answer is yes. In this article I'm going to show you how to package an application for android using Google Colab.
First thing's first, let's code up our application. I assume you already have kivy and kivymd installed in a virtual environment.
Create two files:
- main.py
- main.kv
Inside main.py
, add the following code:
from kivymd.app import MDApp
from kivymd.uix.list import OneLineIconListItem, IconLeftWidget
class MainApp(MDApp):
def build(self):
self.theme_cls.primary_palette = "Purple"
def add_item(self, text):
new_list_item = OneLineIconListItem(text=text)
new_list_item.add_widget(
IconLeftWidget(icon = "language-python")
)
self.root.ids.listcontainer.add_widget(new_list_item)
self.root.ids.listinput.text = ''
if __name__ == "__main__":
app = MainApp()
app.run()
Inside main.kv
add the following lines:
MDBoxLayout:
orientation: 'vertical'
id: box
MDTopAppBar:
title: 'SampleApp'
pos_hint: {'top': 1}
MDTextField:
id: listinput
hint_text: 'Add Item'
mode: 'rectangle'
size_hint_x: .9
pos_hint: {'center_x': .5}
text_validate_unfocus: False
on_text_validate: app.add_item(listinput.text)
ScrollView:
MDList:
id: listcontainer
This is a simple application that gets text from the user and creates a list item and adds it to the list.
When we run our application, it looks like this:
Now for packaging!π¦
Open google colab on your web browser and create a new notebook or you can use my notebook.
Now create a cell and run the following lines:
!sudo apt update
!sudo apt install -y git zip unzip openjdk-17-jdk python3-pip autoconf libtool pkg-config zlib1g-dev libncurses5-dev libncursesw5-dev libtinfo5 cmake libffi-dev libssl-dev
!pip3 install --user --upgrade Cython==0.29.33 virtualenv
Luckily for you, the developers of kivymd decided to make the process easier so we can install buildozer dependencies in one fell swoop. Create a cell in your notebook and add the following lines and execute:
# git clone, for working on buildozer
!git clone https://github.com/kivy/buildozer
%cd buildozer
!python setup.py build
!pip install -e .
%cd ..
And now, everything is ready for the packaging process.
Upload your files to colab by clicking on the files icon and then right clicking and clicking upload.(Uuuh what?π«)
After the files have been uploaded, run the following command:
!buildozer init
This will create a file named buildozer.spec. Double click the file to edit it. You can change the application name and the package name. I changed mine as follows:
Next, scroll down to the requirements and change them as follows:
requirements = python3, kivy==2.1.0, kivymd==1.1.1, sdl2_ttf==2.0.15, pillow
Note: If your application requires special permissions such as internet or storage, scroll down to
android.permissions
:
Uncomment the android.permissions line and add the permissions. For a full list of all the available permissions, check out this link.
Now to package our application. Run the following command:
!buildozer -v android debug
This process can take up to 20 minutes so you might need a little bit of patience. In the mean time you could skip to the bonus section below or watch an episode of my hero academia(I won't judgeπ).
After successful execution, the apk file is saved in the bin folder:
Download the apk, transfer it over to your android device and install it!
The application works!π
Full Code is available HERE.
Bonus section(Not really)
Debugging our application.
Packaging applications this way is faster than having to go through installing all the requirements on your PC but this does have a draw back. How do you debug the application? Imagine successfully installing the application and trying to run it only for it to crash. How do you know what the problem is? You can't run buildozer -v android deploy run logcat
which displays the logs so that you can search through them to identify errors. You can't do that on colab but you can if you're on Linux(perhaps your should just switch to linuxπ).
This is where adb comes in. Android Debug Bridge (adb) is a versatile command-line tool that lets you communicate with a device. You can get more information about it here but for now, we are going to be making use of adb platform tools. Download them here.
This will download a zipped file. Unzip it then copy the apk that we just made and paste it inside the unzipped folder. The selected item is the apk file in the image below:
Connect your device, make sure usb debugging is enabled. Now open a command prompt terminal and navigate to the folder containing the adb files we extracted:
Run adb install name-of-the-app.apk
This will install the application on your device! You can open the application and try it out. To view the application log, run the following command in the terminal:
adb logcat
Now you can see the application log. If any error occurs while running the application, you'll probably find the details in the log, but be warned, it can be like looking for a needle in a haystack!
If you want to learn more about adb and it's commands check out this link.
And that just about wraps it up. I hope you found this article helpful. If you're facing any challenges don't hesitate to reach out in the comments section.
Top comments (46)
NN,
Completed the build but had to change the version of Cython from 0.29.19 to 0.29.21 to complete compile.
ERROR: pip's dependency resolver does not currently take into account all the packages that are installed. This behaviour is the source of the following dependency conflicts.
tables 3.8.0 requires cython>=0.29.21, but you have cython 0.29.19 which is incompatible.
!pip install --upgrade Cython==0.29.21 virtualenv
I suspect that's why the resulting APK builds but bombs after the splash screen.
Do you have any suggestions?
RAHRAH
Hi,
So a few things have changed since I've written the article. I have updated it. You can fix the issues you are facing by changing the requirements to:
requirements = python3, kivy==2.1.0, kivymd==1.1.1, sdl2_ttf==2.0.15, pillow
And for installing the initial build packages, instead of using the commands with Cython, use the following:
This should fix the errors your were encountering. Hope that helps!
i have been trying to follow your tutorial on the above project and there
I have tried to follow the required steps from the tutorial including this update but google colab is not creating the apk file as the bin folder is empty.
Please help
Hey. Are you getting any errors during execution?
No errors but it creates an empty bin folder
have you tried running the code from your end again?
I can't run it at the moment but could you try this notebook.
thank you very much the apk file was created but the application doesn't on my mobile after installation. i tries to open then shuts down
Could you try installing your app with
adb
, then runningadb logcat
, before opening it. The error should be printed within the console.Thank you very much
all working good now i think i missed something along the way
you're are the best keep doing the good job
My question is what did you change in the code to make it work this time
Hi, I have a question about an error which appears while running the App on my Android Phone (Huawei P8lite).
The adb logcat log shows me the two Errors [ E libEGL : validate_display:255 error 3008 (EGL_BAD_DISPLAY) ] and [ ModuleNotFoundError: No module named 'PIL' ] and than crashes.
Do you have an idea how to solve this Error?
I have already done some research and tried to get some information but PIL is dead and the requirement for KivyMD is Pillow, like the devolopers published in Github.
I would appreciate your help and thank you in advance.
If you need some logs to look at I will provide it to you.
Hello. Did you try adding pillow to the requirements in the buildozer.spec file?
I've tried it with
[requirements = kivy==2.0.0, github.com/kivymd/KivyMD/archive/m...]
as the developers published it on Github(github.com/kivymd/KivyMD)
and with
[requirements = python3, kivy==2.0.0, kivymd==0.104.2, sdl2_ttf == 2.0.15, pillow]
In both cases the adb logcat log showed me the two Errors.
Have i forgotten something?
Have you tried running:
between the changes? If not, try running it and using the following requirements:
If you have tried this and still gotten the error, could you please provide the logs.
I'm pretty sure that adding
pillow
to the requirements was supposed to work.I've tried running buildozer with your mentioned requirements and it worked finally. Thank you very much for taking your time and for your help. π₯³
I'm so happy that the "compilation" is working.
A new small problem accoured with a more advanced kivy code. The code runs at the PC but not on my phone. [ TypeError: 'int' object is not iterable ]
Is this a typical error? And is the KivyMD/Python code the problem?
Awesome, I'm glad that worked.
I think the
TypeError
is probably a bug in your code. It means somewhere your code is trying to iterate over anint
object. Try to look through your code for possible causes if the log is not returning the line causing the error.Hey, could you help me with my app?, I've been trying several ways in builderdozer and I still can't get the app to work, it closes when I open it, I use kivymd, pillow in the requirements.
Hello.
Are you developing the application described in this article or another?
Hey, could you help me with my app?, I've been trying several ways in builderdozer and I still can't get the app to work, it closes when I open it, I use kivymd, pillow in the requirements.
Are there no errors appearing in the log?
Hey bro.
I'm having a problem packaging my python program in Colab.
i got the apk but it crashes and even when i'm trying to build on wsl: ubuntu 22.04, it was unsuccessful. Can i get your whatsapp number ?
please its urgent
Hey. Have you tried debugging with adb logcat?
Yes. But I'm still a beginner I couldn't debug
Can I submit the program here ?
share on link my email: ngonidzashedelight@gmail.com
Sent. Please help me
Bro. Please reply my mail π
Greetings, I am currently attempting to transform my python code into an APK, however, after converting it and installing the file, it unfortunately crashes right after the kivy logo appears. I am using google collab to convert my code into APK.
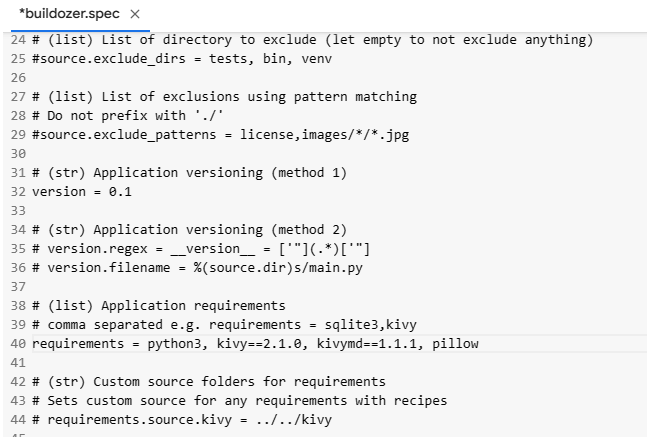
Spec file
main.py
please help me bro
Hi, I am creating an android app using kivy and buildozer to manage the TelloEDU drone and everything is working fine until im trying to get the image. On my computer, when i ask for get_frame_read().frame (works with opencv VideoCapture) after a couple seconds and some:
[h264 @ 00000272a264c700] non-existing PPS 0 referenced
[h264 @ 00000272a264c700] non-existing PPS 0 referenced
[h264 @ 00000272a264c700] decode_slice_header error
[h264 @ 00000272a264c700] no frame!
The image start flowing.
I think that is the problem then on my android. Just at the moment that I am asking for the image, it crashes with "A/libc: Fatal signal 7 (SIGBUS), code 1 (BUS_ADRALN)".
That is the partcode to upload the frames:
from kivy.app import App
from kivy.lang import Builder
from kivy.uix.screenmanager import Screen, ScreenManager
from tellopy import tello
import cv2
from kivy.clock import Clock
me = tello.Tello()
class WindowManager(ScreenManager):
pass
class ControlWindow(Screen):
kv = Builder.load_file("my.kv")
class MyApp(App):
def build(self):
self.Title = "Control BΓ‘sico Tello EDU"
return kv
if name == 'main':
MyApp().run()
The kv file is:
WindowManager:
ControlWindow:
:
Image:
id: video
source: "imagen.jpg"
The buildozer .spec file is:
[app]
(str) Title of your application
title = Tello EDU Basic Control
(str) Package name
package.name = TelloEDUBasicControl
(str) Package domain (needed for android/ios packaging)
package.domain = org.test.TelloEDUBasicControl
(str) Source code where the main.py live
source.dir = .
(list) Source files to include (let empty to include all the files)
source.include_exts = py,png,jpg,kv,atlas,txt
(list) List of inclusions using pattern matching
source.include_patterns = assets/,images/.png
(list) Source files to exclude (let empty to not exclude anything)
source.exclude_exts = spec
(list) List of directory to exclude (let empty to not exclude anything)
source.exclude_dirs = tests, bin
(list) List of exclusions using pattern matching
source.exclude_patterns = license,images//.jpg
(str) Application versioning (method 1)
version = 0.1
(str) Application versioning (method 2)
version.regex = version = '"['"]
version.filename = %(source.dir)s/main.py
(list) Application requirements
comma separated e.g. requirements = sqlite3,kivy
requirements = python3,kivy,tellopy,opencv,numpy
(str) Custom source folders for requirements
Sets custom source for any requirements with recipes
requirements.source.kivy = ../../kivy
(list) Garden requirements
garden_requirements =
(str) Presplash of the application
presplash.filename = %(source.dir)s/data/presplash.png
(str) Icon of the application
icon.filename = %(source.dir)s/data/icon.png
(str) Supported orientation (one of landscape, sensorLandscape, portrait or all)
orientation = landscape
(list) List of service to declare
services = NAME:ENTRYPOINT_TO_PY,NAME2:ENTRYPOINT2_TO_PY
OSX Specific
author = Β© Copyright Info
change the major version of python used by the app
osx.python_version = 3
Kivy version to use
osx.kivy_version = 1.9.1
Android specific
(bool) Indicate if the application should be fullscreen or not
fullscreen = 0
(string) Presplash background color (for new android toolchain)
Supported formats are: #RRGGBB #AARRGGBB or one of the following names:
red, blue, green, black, white, gray, cyan, magenta, yellow, lightgray,
darkgray, grey, lightgrey, darkgrey, aqua, fuchsia, lime, maroon, navy,
olive, purple, silver, teal.
android.presplash_color = #FFFFFF
(list) Permissions
android.permissions = INTERNET, ACCESS_NETWORK_STATE, WRITE_EXTERNAL_STORAGE, READ_EXTERNAL_STORAGE, MANAGE_EXTERNAL_STORAGE
(int) Target Android API, should be as high as possible.
android.api = 31
(int) Minimum API your APK will support.
android.minapi = 21
(int) Android SDK version to use
android.sdk = 30
(str) Android NDK version to use
android.ndk = 23b
(int) Android NDK API to use. This is the minimum API your app will support, it should usually match android.minapi.
android.ndk_api = 21
(bool) Use --private data storage (True) or --dir public storage (False)
android.private_storage = True
(str) Android NDK directory (if empty, it will be automatically downloaded.)
android.ndk_path =
(str) Android SDK directory (if empty, it will be automatically downloaded.)
android.sdk_path =
(str) ANT directory (if empty, it will be automatically downloaded.)
android.ant_path =
(bool) If True, then skip trying to update the Android sdk
This can be useful to avoid excess Internet downloads or save time
when an update is due and you just want to test/build your package
android.skip_update = False
(bool) If True, then automatically accept SDK license
agreements. This is intended for automation only. If set to False,
the default, you will be shown the license when first running
buildozer.
android.accept_sdk_license = False
(str) Android entry point, default is ok for Kivy-based app
android.entrypoint = org.renpy.android.PythonActivity
(str) Android app theme, default is ok for Kivy-based app
android.apptheme = "@android:style/Theme.NoTitleBar"
(list) Pattern to whitelist for the whole project
android.whitelist =
(str) Path to a custom whitelist file
android.whitelist_src =
(str) Path to a custom blacklist file
android.blacklist_src =
(list) List of Java .jar files to add to the libs so that pyjnius can access
their classes. Don't add jars that you do not need, since extra jars can slow
down the build process. Allows wildcards matching, for example:
OUYA-ODK/libs/*.jar
android.add_jars = foo.jar,bar.jar,path/to/more/*.jar
(list) List of Java files to add to the android project (can be java or a
directory containing the files)
android.add_src =
(list) Android AAR archives to add (currently works only with sdl2_gradle
bootstrap)
android.add_aars =
(list) Gradle dependencies to add (currently works only with sdl2_gradle
bootstrap)
android.gradle_dependencies =
(list) add java compile options
this can for example be necessary when importing certain java libraries using the 'android.gradle_dependencies' option
see developer.android.com/studio/write... for further information
android.add_compile_options = "sourceCompatibility = 1.8", "targetCompatibility = 1.8"
(list) Gradle repositories to add {can be necessary for some android.gradle_dependencies}
please enclose in double quotes
e.g. android.gradle_repositories = "maven { url 'kotlin.bintray.com/ktor' }"
android.add_gradle_repositories =
(list) packaging options to add
see google.github.io/android-gradle-ds...
can be necessary to solve conflicts in gradle_dependencies
please enclose in double quotes
e.g. android.add_packaging_options = "exclude 'META-INF/common.kotlin_module'", "exclude 'META-INF/*.kotlin_module'"
android.add_gradle_repositories =
(list) Java classes to add as activities to the manifest.
android.add_activities = com.example.ExampleActivity
(str) OUYA Console category. Should be one of GAME or APP
If you leave this blank, OUYA support will not be enabled
android.ouya.category = GAME
(str) Filename of OUYA Console icon. It must be a 732x412 png image.
android.ouya.icon.filename = %(source.dir)s/data/ouya_icon.png
(str) XML file to include as an intent filters in tag
android.manifest.intent_filters =
(str) launchMode to set for the main activity
android.manifest.launch_mode = standard
(list) Android additional libraries to copy into libs/armeabi
android.add_libs_armeabi = libs/android/*.so
android.add_libs_armeabi_v7a = libs/android-v7/*.so
android.add_libs_arm64_v8a = libs/android-v8/*.so
android.add_libs_x86 = libs/android-x86/*.so
android.add_libs_mips = libs/android-mips/*.so
(bool) Indicate whether the screen should stay on
Don't forget to add the WAKE_LOCK permission if you set this to True
android.wakelock = False
(list) Android application meta-data to set (key=value format)
android.meta_data =
(list) Android library project to add (will be added in the
project.properties automatically.)
android.library_references =
(list) Android shared libraries which will be added to AndroidManifest.xml using tag
android.uses_library =
(str) Android logcat filters to use
android.logcat_filters = *:S python:D
(bool) Copy library instead of making a libpymodules.so
android.copy_libs = 1
(str) The Android arch to build for, choices: armeabi-v7a, arm64-v8a, x86, x86_64
android.arch = armeabi-v7a
(int) overrides automatic versionCode computation (used in build.gradle)
this is not the same as app version and should only be edited if you know what you're doing
android.numeric_version = 1
Python for android (p4a) specific
(str) python-for-android fork to use, defaults to upstream (kivy)
p4a.fork = kivy
(str) python-for-android branch to use, defaults to master
p4a.branch = master
(str) python-for-android git clone directory (if empty, it will be automatically cloned from github)
p4a.source_dir =
(str) The directory in which python-for-android should look for your own build recipes (if any)
p4a.local_recipes =
(str) Filename to the hook for p4a
p4a.hook =
(str) Bootstrap to use for android builds
p4a.bootstrap = sdl2
(int) port number to specify an explicit --port= p4a argument (eg for bootstrap flask)
p4a.port =
iOS specific
(str) Path to a custom kivy-ios folder
ios.kivy_ios_dir = ../kivy-ios
Alternately, specify the URL and branch of a git checkout:
ios.kivy_ios_url = github.com/kivy/kivy-ios
ios.kivy_ios_branch = master
Another platform dependency: ios-deploy
Uncomment to use a custom checkout
ios.ios_deploy_dir = ../ios_deploy
Or specify URL and branch
ios.ios_deploy_url = github.com/phonegap/ios-deploy
ios.ios_deploy_branch = 1.7.0
(str) Name of the certificate to use for signing the debug version
Get a list of available identities: buildozer ios list_identities
ios.codesign.debug = "iPhone Developer: ()"
(str) Name of the certificate to use for signing the release version
ios.codesign.release = %(ios.codesign.debug)s
[buildozer]
(int) Log level (0 = error only, 1 = info, 2 = debug (with command output))
log_level = 2
(int) Display warning if buildozer is run as root (0 = False, 1 = True)
warn_on_root = 1
(str) Path to build artifact storage, absolute or relative to spec file
build_dir = ./.buildozer
(str) Path to build output (i.e. .apk, .ipa) storage
bin_dir = ./bin
-----------------------------------------------------------------------------
List as sections
You can define all the "list" as [section:key].
Each line will be considered as a option to the list.
Let's take [app] / source.exclude_patterns.
Instead of doing:
[app]
source.exclude_patterns = license,data/audio/.wav,data/images/original/
This can be translated into:
[app:source.exclude_patterns]
license
data/audio/*.wav
data/images/original/*
-----------------------------------------------------------------------------
Profiles
You can extend section / key with a profile
For example, you want to deploy a demo version of your application without
HD content. You could first change the title to add "(demo)" in the name
and extend the excluded directories to remove the HD content.
[app@demo]
title = My Application (demo)
[app:source.exclude_patterns@demo]
images/hd/*
Then, invoke the command line with the "demo" profile:
buildozer --profile demo android debug
Do you know where is the problem?
Hello.
Honestly I have no idea where the problem could be. I haven't worked with tello before but I'm probably guessing it could be something to do with cv2 or how the video is being loaded with get_frame_read. Check out this link which has a demo on using opencv with kivy, it might help.
hello, im making an app with pandas, kivymd. colab just doesnt work, on research i found i have to edit spec file to p4a.branch=develop but it still doesnt work, i did put kivymd and pandas in requirements with their versions, please help i have put a lot of efforts in this app and its frustrating to see it crash after presplash
Hi.
Did you try to locate the error with
adb logcat
?I managed to create an apk using Coollaboratory and it works, but the Android device cannot install subsequent versions to the previous one, even if nothing changes in the code. How can I organize the apk update on the device?
ModuleNotFoundError: No module named '_ctypes'
The above error keeps getting raised after following the steps you have outlined. Kindly assist
Hey
I've updated the article. I believe one of the required packages was missing. Try to run your program with the latest notebook.
Thank you! This worked out for me.
Am also using pyrebase in my app. Is there a way to know all the dependencies required?
To use pyrebase in your application, you just need to add it to the requirements in the spec file. When you run buildozer, it will automatically download any dependencies that pyrebase needs.
However, if you encounter an error with pyrebase, it could be because it's a Python package that requires compilation, and there is no specific support for it in Python-for-android. In such cases, the package won't work as expected.
If you want to make pyrebase work with Python-for-android, you can contribute to the Python-for-android project by creating a recipe specifically for pyrebase. By creating a recipe, you provide the necessary instructions for Python-for-android to properly compile and include pyrebase in the final application package.
Okay
app open and close direct
I/python (32368): [Clang 12.0.8 (android.googlesource.com/toolchain... c935d99d
I/python (32368): [INFO ] [Python ] Interpreter at ""
I/python (32368): [INFO ] [KivyMD ] 1.1.0.dev0, git-Unknown, 2022-09-24 (installed at "/data/data/org.test.eiranstudio/files/app/python_bundle/site-packages/kivymd/init.pyc")
I/python (32368): [INFO ] [Factory ] 186 symbols loaded
I/python (32368): Traceback (most recent call last):
I/python (32368): File "/content/.buildozer/android/app/main.py", line 1, in
I/python (32368): File "/content/.buildozer/android/platform/build-arm64-v8a_armeabi-v7a/build/python-installs/EiranStudio/armeabi-v7a/kivymd/init.py", line 66, in
I/python (32368): File "/content/.buildozer/android/platform/build-arm64-v8a_armeabi-v7a/build/python-installs/EiranStudio/armeabi-v7a/kivymd/font_definitions.py", line 10, in
I/python (32368): File "/content/.buildozer/android/platform/build-arm64-v8a_armeabi-v7a/build/python-installs/EiranStudio/armeabi-v7a/kivy/core/text/init.py", line 85, in
I/python (32368): File "/content/.buildozer/android/platform/build-arm64-v8a_armeabi-v7a/build/python-installs/EiranStudio/armeabi-v7a/kivy/graphics/init_.py", line 89, in
I/python (32368): ModuleNotFoundError: No module named 'kivy.graphics.instructions'
Hi. I have an issue where after building app (kivy,kivymd), it didn't work. I looked up for the logcat and noticed that there is an issue with kivymd2.0.1.dev0
it doesnt recognize MDFAButton. This is declared requirements:
requirements = python3,pynmea2,kivy_garden==0.1.5,kivy==2.3.0,kivymd==2.0.1.dev0
And another question is how to make a permission for apk app, for socket comm, gps (location) ?
Some comments have been hidden by the post's author - find out more