This is 2nd post of the series where we will learn what is JSX and why to use it with react.
What is JSX ?
JSX stands for JavaScript XML. It allows us to write HTML inside JavaScript. It converts HTML tags into React Elements.
Why JSX is required?
If you remember in the last post we creates our Hello World App using create-react-app
. The code inside App.js file looks like this.
import React from "react";
function App() {
/////////////////////////////
return (
<div>
<h1>Hello World!</h1>
</div>
);
//////////////////////////////
}
export default App;
So what is you don't want to use JSX. Let's see how can we do that?
import React from "react";
function App() {
/////////////////////////////
return (
React.createElement('div', null,
React.createElement(
'h1', null, `Hello World!`
))
);
//////////////////////////////
}
export default App;
This is the same code written without JSX. So, JSX allows us to write HTML elements in JavaScript without using any createElement()
or appendChild()
methods.
You are not required to use JSX, but JSX makes it easier to write React applications. Each JSX element is just syntactic sugar for calling React.createElement.
So, anything you can do with JSX can also be done with just plain JavaScript.
Rules for coding JSX
While writing JSX code you should keep the following things in mind.
- Inserting JavaScript expressions. Any valid JavaScript expression can be inserted in JSX code by using the curly braces {}.
import React from "react";
function App() {
return (
<div>
<h1>The sum of 6 and 9 is {6+9}</h1>
</div>
);
}
export default App;
- Top level Element All HTML code inside JSX must be wrapped in ONE top level element. So while writing multiple elements, you must put them inside one single parent element.
// WRONG WAY
function App() {
return (
<h1>Hey, React!</h1>
<h1>I love JSX!</h1>
);
}
// CORRECT WAY
function App() {
return (
<div>
<h1>Hey, React!</h1>
<h1>I love JSX!</h1>
</div>
);
}
- A block of HTML code To write chunks of HTML on multiple lines, put the HTML inside parentheses and store it in a variable. This variable can be used anywhere in place of HTML.
const myelem = (
<div>
<h1>Hello React!</h1>
<p>I love JSX!</p>
<p>This is a block of code. </p>
</div>
);
function App() {
return myelem;
}
- All elements must be closed All HTML elements must be properly closed. JSX will throw an error if HTML is not properly closed, it misses a parent element of incorrect in any other way.
const myelem = (
<div>
<img src="img.png" alt="Yay" />
<input type="text" />
<br/>
</div>
);
function App() {
return myelem;
}
// NOTICE THAT EVEN SELF CLOSING TAGS ARE PROPERLY CLOSED
*React doesn't require using JSX, * but most people find it helpful as a visual aid when working with UI inside the JavaScript code.
It also allows React to show more useful error and warning messages.
You can use the comments for anythingπ
β‘Thanks For Reading | Happy Coding βΎ
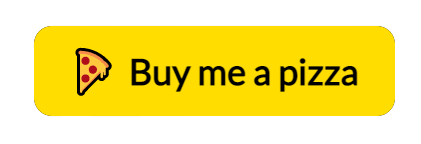
Top comments (6)
It's worth adding that JSX is not limited to React, it's language on its own, there are other libraries that use it like Preact or HyperApp.
JSX is an abomination
Thanks, great explanation I just started learning react
You can follow. Everyday a new post for beginners is coming up.
Some comments may only be visible to logged-in visitors. Sign in to view all comments.