String concatenation is the process of combining two or more strings into a single new string. In Go, strings are immutable, which means that once a string is created, its contents cannot be changed. Therefore, concatenation in Go involves creating a new string that is the result of combining two or more existing strings. This can be achieved using the + operator or the fmt.Sprintf function.
It is important to note that using the + operator to concatenate strings can result in inefficient code, especially when dealing with a large number of strings. In such cases, using the strings.Builder type or the bytes.Buffer type can be more efficient.
In this article, we will explore different ways to concatenate strings in Golang, and compare their performance to help developers make informed decisions when selecting the most efficient method for their applications. This guide will cover various techniques and best practices for string concatenation in Golang, including the + operator, fmt.Sprintf function, strings.Join function, and bytes.Buffer type.
Basic Concatenation with the + Operator
One way to concatenate strings in Go is by using the + operator. This operator allows you to concatenate two or more strings together into a single string. When you use the + operator to concatenate two strings, a new string is created that contains the contents of the two original strings.
For example, suppose you have two strings str1 and str2. You can use the + operator to concatenate the two strings as str1 + str2. The resulting string will contain the contents of both str1 and str2. Keep in mind that this method of string concatenation can become inefficient when concatenating multiple strings because it creates a new string every time.
package main
import "fmt"
func main() {
firstName := "John"
lastName := "Doe"
// using the + operator
fullName := firstName + " " + lastName
fmt.Println("Full Name:", fullName)
// using the fmt.Sprintf() function
fullName = fmt.Sprintf("%s %s", firstName, lastName)
fmt.Println("Full Name:", fullName)
// using the strings.Join() function
nameParts := []string{firstName, lastName}
fullName = strings.Join(nameParts, " ")
fmt.Println("Full Name:", fullName)
}
The output of the code above should be:
Full Name: John Doe
Full Name: John Doe
Full Name: John Doe
Although Golang string concatenation is efficient and convenient, it has some limitations and drawbacks. One of the main limitations is its immutability, which means that it cannot be modified once created. This can be a problem when dealing with large strings, as it may require the creation of multiple intermediate strings.
Additionally, the use of the + operator for concatenation may lead to memory allocation and deallocation issues, especially if done repeatedly in a loop. Another drawback is the lack of a built-in string builder, which can make the concatenation process less efficient and more error-prone.
## String Concatenation in Go Using StringBuilder
In Golang, StringBuilder is a data structure that allows you to concatenate strings efficiently. It is a dynamic array of bytes that can be used to generate strings by appending or inserting characters into the array. The initial capacity of the StringBuilder can be set to an estimate of the final string size, which can help reduce memory allocation and improve performance.
Additionally, StringBuilder provides a convenient way to modify strings without creating new objects, which can be particularly useful when dealing with large strings or high-performance applications. Overall, the use of StringBuilder in Golang can lead to faster and more efficient string concatenation, making it a valuable tool for developers.
The + operator method can be inefficient if there are many concatenations involved. This is where the strings.Builder type comes in handy. It allows for efficient string concatenation by pre-allocating memory and minimizing memory allocations.
To use strings.Builder, you first create an instance of it using the strings.Builder() function. Then, you can use its WriteString() method to append strings to the builder. Finally, you can get the concatenated string using the String() method. Here's an example:
go
var builder strings.Builder
builder.WriteString("Hello, ")
builder.WriteString("world!")
result := builder.String()
fmt.Println(result) // Output: Hello, world!
In this example, we create an instance of strings.Builder, append two strings using WriteString(), and then get the final concatenated string using String(). The result is printed to the console, which outputs "Hello, world!".
The use of the + operator to concatenate strings creates a new string each time it is called. This can lead to excessive memory allocation and affect the overall performance of an application.
## Concatenate String in Go Using Strings.Join()
In Go, strings.Join() is a powerful built-in function used for concatenating strings efficiently. This function takes a slice of strings as its first argument and a separator string as its second argument. The function then concatenates all the strings in the slice with the separator in between each string.
One of the main benefits of using strings.Join() is that it is more efficient than using the + operator or fmt.Sprintf() for concatenation, particularly when dealing with a large number of strings. This is because strings.Join() uses a buffer to build the final string, making it faster and more memory-efficient than other concatenation methods.
Here's an example usage:
go
package main
import (
"fmt"
"strings"
)
func main() {
words := []string{"hello", "world", "how", "are", "you"}
joined := strings.Join(words, " ")
fmt.Println(joined)
}
In this example, we have a slice of strings called words and we want to concatenate them with a single space as a separator. We pass the slice and the separator to the strings.Join() function and store the result in a variable called joined. Finally, we print the concatenated string using fmt.Println().
The output of this program will be:
go
hello world how are you
As you can see, the strings.Join() function is a simple and effective way to concatenate a slice of strings with a specified separator in Golang.
## Concatenate Go String with Bytes.Buffer
Bytes.Buffer is a type in the Go programming language that provides an efficient way to concatenate strings without creating new string variables in memory. This type operates on byte slices, allowing for efficient byte-level manipulation.
One benefit of using Bytes.Buffer is that it avoids the overhead of creating new strings when concatenating, especially when dealing with larger strings. Additionally, it allows for more precise control over the buffer size and can even be used to write to files or other byte streams.
The bytes.Buffer type is a flexible way to build and manipulate strings. It has a Write method that appends bytes to the buffer and a String method that returns the buffer's contents as a string. Here's an example of using bytes.Buffer to concatenate strings:
go
package main
import (
"bytes"
"fmt"
)
func main() {
var buffer bytes.Buffer
buffer.WriteString("Hello, ")
buffer.WriteString("Gophers!")
fmt.Println(buffer.String())
}
The output of this program will be Hello, Gophers!. With bytes.Buffer, you can append strings in a loop, concatenate several strings together, or build a string piece by piece. It's a powerful and efficient tool for string manipulation in Go.
## Performance comparison between concatenate methods in Go
When it comes to concatenating strings in Go, there are several methods available, each with its own performance characteristics. Here's a comparison of some commonly used concatenation methods:
### + Operator
The simplest method is to use the + operator to concatenate strings. While easy to use, it can result in inefficient memory allocation when concatenating multiple strings. Each concatenation creates a new string, which can lead to performance issues when dealing with large strings or frequent concatenation operations.
### fmt.Sprintf()
The fmt.Sprintf() function allows string concatenation using format specifiers. It provides more flexibility in formatting but can be slower compared to other methods due to the overhead of format parsing and string allocation.
### strings.Join()
The strings.Join() function is designed specifically for concatenating multiple strings. It takes a slice of strings and concatenates them efficiently using a single memory allocation. This method performs well when joining a large number of strings and is generally faster than the + operator and fmt.Sprintf() for such scenarios.
### bytes.Buffer type
The bytes.Buffer type provides a buffer that can be used to efficiently build strings. It offers methods like WriteString() to append strings to the buffer. The bytes.Buffer type minimizes memory allocations by dynamically growing its internal buffer. This approach is particularly useful when concatenating a large number of strings or when performance is critical.
In terms of performance, the strings.Join() and bytes.Buffer methods generally outperform the + operator and fmt.Sprintf() when dealing with frequent or large-scale concatenation. However, the exact performance can vary depending on the specific use case, the number of concatenations, and the sizes of the strings being concatenated.
For the best performance, it is recommended to use strings.Join() when concatenating multiple strings or to use bytes.Buffer for more complex scenarios where efficient string building is required. It's worth noting that benchmarks and profiling specific to your use case can provide more accurate insights into the performance characteristics of different concatenation methods.
## Recommendations for efficient string concatenation
Here are some recommendations to follow for efficient string concatenation in Go:
- Use the strings.Builder type instead of concatenating strings with the + operator or fmt.Sprintf()
- Pre-allocate the necessary space for the strings.Builder to avoid unnecessary allocations
- If concatenating many strings at once, consider using the strings.Join() function instead of a loop with strings.Builder.
- Be mindful of the number of strings being concatenated, as excessive concatenation can cause performance issues.
- If you must concatenate large numbers of strings, consider using a third-party library such as github.com/cespare/xxhash/v2 to create a hash of the strings instead.
## Learn Go Programming with [Go Online Compiler](https://www.lightly-dev.com/go/)
Are you having trouble dealing with errors and debugging when coding? Don't worry, because conquering them is way easier than scaling Mount Everest!
Introducing Lightly IDE, your ultimate coding companion that transforms the learning curve into a thrilling adventure. With Lightly IDE, you don't have to be a coding genius to program smoothly. Discover the wonders of Lightly IDE at [lightly-dev.com](https://www.lightly-dev.com/) today!
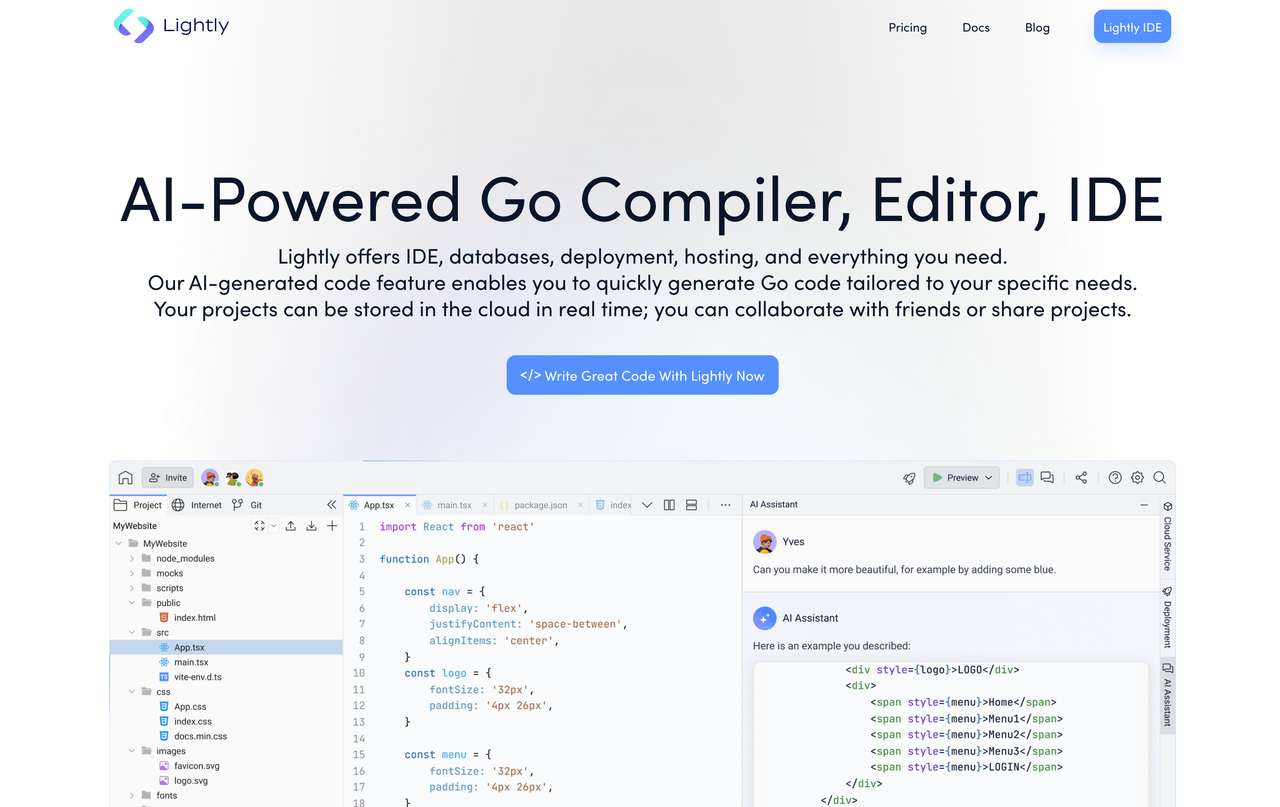
Lightly IDE is truly remarkable, especially with its seamless integration of artificial intelligence (AI). This means that even if you're not a tech whiz, you can effortlessly navigate and utilize all its features. It's like having a magical power at your fingertips, but instead of wands, you're wielding lines of code.
If you're curious about programming or eager to enhance your skills, Lightly IDE provides the perfect entry point. With its Go online compiler, it's like stepping into a playground designed for aspiring programming prodigies. This platform has the incredible ability to transform a complete beginner into a coding expert in no time at all.
Read more: [A Comprehensive Guide for Golang String Concatenation](https://www.lightly-dev.com/blog/golang-concatenate-string/)
Top comments (1)
Nice article, however, check your code formatting, looks missplaced