In this post, we’ll learn how to setup Push notification in Ionic 4 app using Firebase. We’ll send notification using Firebase console and receive them in the app. We will also learn how to handle push notifications in your Ionic 4 apps. Because all this can get reallllly long, I’ll limit the discussion to Android apps alone. I will post another blog for push in iOS apps in Ionic 4.
Complete code for this blog can be found totally FREE at Github

What are Push Notifications
Ok, I’ll try to define it in my own language. How do you know when you get a new message on Whatsapp, Facebook or Twitter, if the app is closed? Yes, those small messages that pop down from the top of the screen, showing new messages, updates or news are called Push notifications (or simply Push)

One might wonder why to integrate Push notifications in an app. Let me tell you, push notifications is the single biggest feature to keep your users engaged with your app. People are obsessed with notifications. People keep checking their phones regularly so they don’t miss any notification. If your app sends regular (and interesting) push, your users will be happy, and you’ll see much less app uninstalls than you’ll see without push.
What is Ionic 4?
You probably already know about Ionic, but I’m putting it here just for the sake of beginners. Ionic is a complete open-source SDK for hybrid mobile app development created by Max Lynch, Ben Sperry and Adam Bradley of Drifty Co. in 2013. Ionic provides tools and services for developing hybrid mobile apps using Web technologies like CSS, HTML5, and Sass. Apps can be built with these Web technologies and then distributed through native app stores to be installed on devices by leveraging Cordova.
So, in other words — If you create Native apps in Android, you code in Java. If you create Native apps in iOS, you code in Obj-C or Swift. Both of these are powerful but complex languages. With Cordova (and Ionic) you can write a single piece of code for your app that can run on both iOS and Android (and windows!), that too with the simplicity of HTML, CSS, and JS.
Structure
I will go ahead in step-by-step fashion so you can follow easily.
- Create a Firebase project and find Push options
- Create a basic Ionic 4 app
- Connect your Ionic 4 app with Firebase and install Push plugin
- Build the app on Android
- Send notifications from Firebase console
- Receive different type of notifications in app
- Handle Push notifications in your app
1. Create a Firebase project and find Push options
Go to Firebase and create your first project (or use an existing one). Your console should look like this

Note — It’s really easy to create a Firebase project, but if you still face any issue, follow step 1–4 of this blog
Click on your project and you’ll enter the project’s dashboard. Look for Cloud Messaging tab. This is where the magic will happen !

Push Notifications Settings
Firebase console also contains push notifications settings for web, android and iOS. Here you can find your sender_id, upload iOS push certificates etc. For setting up options, you’ll first have to create an Android app in Firebase console.

During the process, it will ask you to enter app’s package name and provide google-services.json. Make sure you keep the package name same as what you are setting in config.xml
of your app. (Ionic 4 default package name is io.ionic.starter, don’t use the default package name)

2. Create a basic Ionic 4 app
Creating a basic Ionic 4 app is very easy. Assuming you have all basic requirements installed in your system, run
$ ionic start MyApp blank
This creates your app with titleMyApp
and blank template.
For more details on how to create a basic Ionic 4 app, refer to my blog How to create an Ionic 4 app
With slight modifications, your homepage will look like this.

The file structure looks something like this, just for an idea

3. Install Push plugin in your app
We will implement Push using Ionic Native FCM plugin. To install this plugin, run
$ ionic cordova plugin add cordova-plugin-fcm-with-dependecy-updated
$ npm install @ionic-native/fcm
This will install the plugin. Import the plugin in app.module.ts

Also, import the plugin in your home.page.ts
where we’ll implement certain actions. home.page.ts
code will look like following
Note that we are calling the push plugin methods after platform is ready. This is a good practice when you are initializing the push plugin right after the app starts.
IMPORTANT — Place the google-services.json file you downloaded from Firebase in root of your project. This is extremely important, as this is how the app and Firebase recognize each other. Without this, the app will not build on device.
More plugin details can be found in its Github repository
4. Build the app on Android
If you have carried out the above steps correctly, Android build should be a breeze.
Run following command to create Android platform
$ ionic cordova platform add android
Once platform is added, run the app on device (Make sure you have a device attached to the system. Push notifications might not work on simulator)
$ ionic cordova run android
Once your app is up and running on the device, we’ll send notifications from Firebase console.
5. Send notifications from Firebase console
Now that the app is ready to receive Push notification, let’s see how to send them.
Head to your Firebase console → Cloud messaging section → Get started
You’ll see a form with which you can send a push notification
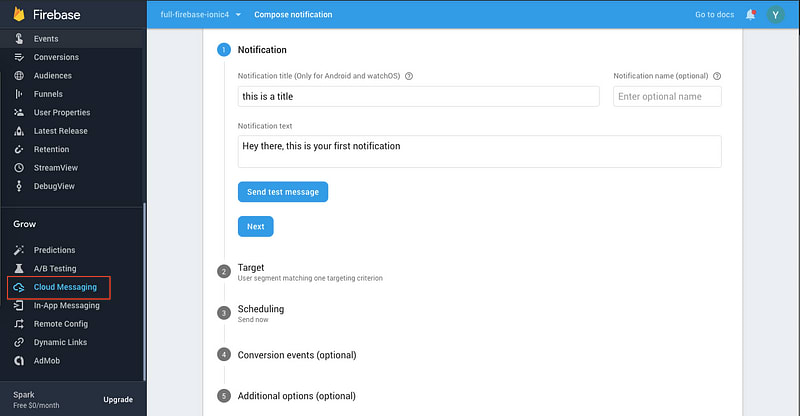
I won’t go into details of all the options available in the dashboard. Let’s setup the basics
- Enter a Notification title
- Enter a Notification text
Leave all the options as is, for now. Now hit Review → Publish the notification. Make sure your app is closed at this point in the device. Your device will receive a notification within a few seconds
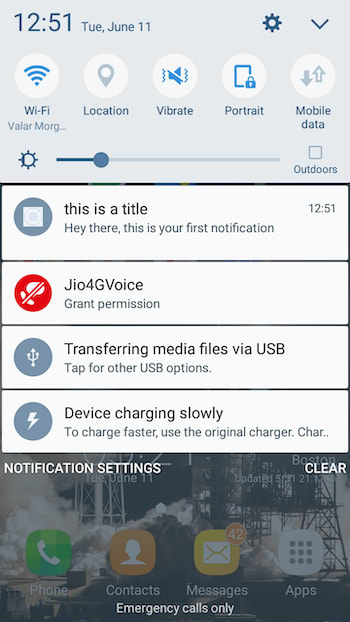
Notice there is no icon for the push because we haven’t defined any icon yet. The notification is showing the default icon of ionic apps.
Congratulations! You just sent your first Push in Ionic 4 🎉 🎉
6. Receive different types of Push in Ionic apps
With FCM, you can send two types of messages to clients:
- Notification Messages, sometimes thought of as “display messages.” These are handled by the FCM SDK automatically. This is what we just sent.
- Data Messages, which are handled by the client app. You can send custom data with these messages, but these require custom back-end (or Firebase cloud functions) to be sent
Notification messages contain a predefined set of user-visible keys. Data messages, by contrast, contain only your user-defined custom key-value pairs. Notification messages can contain an optional data payload. Maximum payload for both message types is 4KB, except when sending messages from the Firebase console, which enforces a 1024 character limit.
Sending and Receiving a Notification message
This is what we just did in the above section — the first notification we sent. Let’s see how it looks in the browser console.

Note, you don’t have any custom data fields here that you can handle
Sending and Receiving a Data message
To send a data message, head over to Additional Options section in Firebase cloud messaging form

When the notification is received, the data appears as follows

These new data fields can be used by the app for any purpose. Currently, all these fields come as String field only, so be careful
7. Handle Push notifications in your app
Push notification can be received in three states in your Ionic app
- When app is in the foreground (open and visible)
- When app is in the background (open but minimized)
- When app is closed (killed)
As you know from daily experience, notifications are required to work in all 3 cases. So we handle them a little differently for each case
7a. When app is in foreground
The plugin we are using, allows foreground notifications to be received silently by default. That means, you do get the data sent by the push, but there is no notification shown in the app.
If you want to show a notification in such case, you can show an alert or customized modal message to the user, like what Tinder shows when you get a match.

7b. When app is in background
When the app is in background, your phone shows a standard notification popping from the top (for most devices ! ). When you tap the notification, you won’t receive any data 😐
Turns out Firebase does not support all the options of Data notifications currently. To receive a callback in the app for this type of notification, you need a key-value pair click-action: FCM_PLUGIN_ACTIVITY
. But this is not possible to view Firebase console.
Ideally, a notification comprises of a lot of data. Following JSON shows most of it. If you are using a custom back-end, you can send all of this data in the notification, and your app will then receive the callback with data.
You can find more details on push notification content syntax in Firebase’s official documentation.
Don’t worry if you don’t have custom back-end for now. You can use https://cordova-plugin-fcm.appspot.com/ to send notifications for testing.
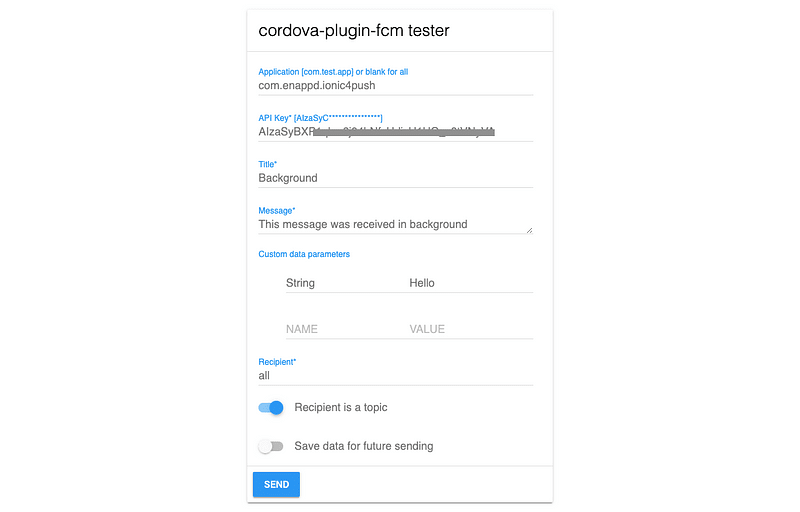
Get the API key from Firebase console → Project Settings → Cloud Messaging → Legacy server Key
Now when you receive the notification in background, and tap the notification, you receive the data inside the app like following

7c. When app is closed
When the app is closed, your phone shows a standard notification popping from the top (for most devices ! ). If you have sent the notification from Firebase, again, the data won’t show up in the callback.
But if you are sending the notification from cordova-plugin-fcm.appspot.com, or with your custom back-end, you receive the data in the same format as above. Now you can use the data to perform any action inside the app.
You are probably wondering how does our push notification list look like now. Since we are not saving anything in local storage, it will only record the current session’s push notification. The list looks like this after a few notifications.
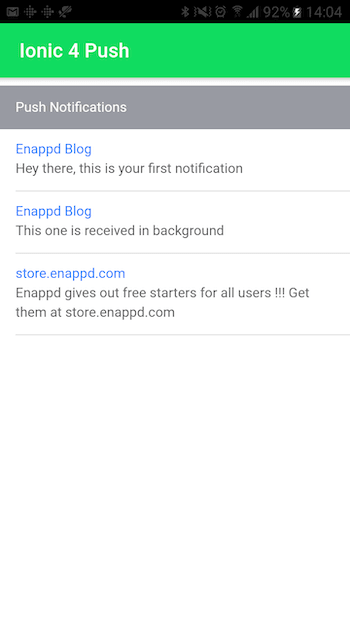
Conclusion
In this post, we learned how to setup Firebase push notifications in an Ionic 4 app, how to send notifications, how to receive different types of notifications and how to handle them in the app. We learned what is the syntax for push notifications is if you want to send it from a custom back-end. We also created a small Ionic 4 app with provisions to show the received push notifications as a list.
Complete code for this blog can be found totally FREE at Github
Stay tuned for more Ionic blogs!
Next Steps
Now that you have learned the implementation of Firebase push notifications in Ionic 4, you can also try
- Ionic 4 PayPal payment integration — for Apps and PWA
- Ionic 4 Stripe payment integration — for Apps and PWA
- Ionic 4 Apple Pay integration
- Twitter login in Ionic 4 with Firebase
- Facebook login in Ionic 4 with Firebase
- Geolocation in Ionic 4
- QR Code and scanners in Ionic 4 and
- Translations in Ionic 4
If you need a base to start your next Ionic 4 app, you can make your next awesome app using Ionic 4 Full App

References
This blog was originally published on enappd.com
Oldest comments (1)
Also a simple way to integrate push notifications in ionic Cordova.
Create an account (free, no credit card required).
indigitall.com/latinoamerica/plan-...
Here you can see the documentation in Córdova
docs.indigitall.com/en/indigitalls...