I am going to be shutting down this service as of 3/12/23. If you would like to run your own instance of this, go to the country flags repository, download the code and run it on your own VPS.
Introduction
I was recently working on a project that required me to implement country flags into the application. At the time, countryflags.io was a popular API that people were using, so I decided that I should rely on it for my project. While integrating the endpoints for countryflags.io into my application, I noticed that they only allowed user's to retrieve a country's flag by a two letter code. For example, if I wanted to get the flag of the United States, the endpoint would be https://www.countryflags.io/us/flat/64.png
. This was a problem because my data didn't identify countries by their two letter codes. Another problem I ran into is that the server of countryflags.io went down half way through the project. So to fix these problems, I created my own API that allows users to get a country's flag by the country's name, its two letter code (ISO Alpha 2 code), its three letter code (ISO Alpha 3 code), and its UN Code. The codes for each country can be found at countryflagsapi.com. Here are a few example endpoints
https://countryflagsapi.com/png/br
https://countryflagsapi.com/png/brazil
https://countryflagsapi.com/svg/076
https://countryflagsapi.com/svg/bra
Running Your Own Instance
If you wish to run your own instance of this API, you can download the source code here. If you would prefer to just pull a docker image of the API, you can get that here
Getting a Flag from User Input
I'll be using React for this demo. Keep in mind this is a basic demo, but this will probably be similar to what you will want
to use in your app.
Imports
import React, { useState } from 'react'
States and Functions
const [flagURL, setFlagURL] = useState('https://countryflagsapi.com/png/cuba')
const [identifier, setIdentifier] = useState('')
const handleButtonClick = () => {
// can use https://countryflagsapi.com/svg/ here too
const url = 'https://countryflagsapi.com/png/'
setFlagURL(url + identifier)
}
JSX
<div style={{ marginBottom: '20px' }}>
<input
name="country"
type="text"
onChange={(e) => setIdentifier(e.target.value)}
value={identifier}
placeholder="Enter a country name, UN Code, ISO Alpha-2, or ISO Alpha-3 Code"
/>
<button onClick={handleButtonClick}>Get Flag</button>
</div>
<img src={flagURL} alt="flag" />
Embedding an Image
Go to the flag that you want to embed and click the button labeled "Embed SVG" or "Embed PNG". A modal will then appear like the one shown in the image below. Go ahead and click copy and then paste the img element into your source code.
Latest comments (4)
Thank you so much!!
You can just use one line of JS - without any API at all:
I looked into this solution but Windows 10 doesn't seem to support flag emojis. This is the example on Windows
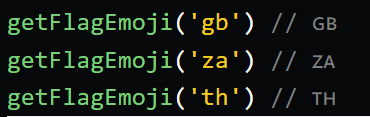
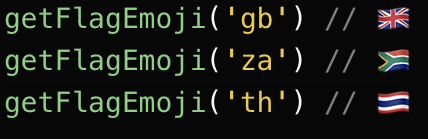
compared to other operating systems.
Also, one of the drawbacks that I encountered working with other flag APIs is that you can only access a flag by its 2 letter country code. This became a pain to work with because the API that was supplying me with country data didn't include a 2 letter country code. That's why at countryflagsapi you can get a country's flag by the country's name, two letter code, three letter code, or UN Code. But if the application has access to the two letter country code and doesn't mind not having flags being shown on Windows 10, I think your solution is the way to go.
Man, Windows is a total joke... why do people still use it?
Some comments may only be visible to logged-in visitors. Sign in to view all comments.