No matter what programming language you pick up to develop strong software, you always end up using functions to make code more effective.
A function is a piece of code that performs a task, it's made to be reusable and saves many coding lines. Do you remember your algebra classes? Well, a JavaScript function is similar to those of your math lectures in a certain way.
So, in this blog you're going to learn about JavaScript functions and how to use them.
Understanding a JavaScript function syntax
A function has a name, input arguments, its logics and something to output. So, basically, the syntax of a function in JavaScript is:
function myFunc(arg1, arg2, arg3) {
//Function logics
}
Where:
-
function
is the reserved word to make JavaScript understand it's a function. -
myFunc
is your function's name. You can use any name, but you may want a name that makes sense to you. -
arg1
,arg2
,arg3
... are the input arguments. They can be as many as you need. Or your function may need no argument at all. -
//Function logics
is into curly braces{}
and this is where all the magic of the function happens. It contains the code to be executed.
Function Expression
In JavaScript you can store a function in a variable and invoke it with the variable name:
const multiplicacion = function mult(num1, num2) {
return num1 * num2
}
```
Now, if we want to invoke this function, we code this:
```
const operacion = multiplicacion(3, 4)
//Expected output: 12
```
**BE CAREFUL!** You can't call `mult` as a function, it will return an error:
```
const operacion2 = mult(3, 4)
//Expected output: Uncaught ReferenceError: mult is not defined
```
Notice the keyword `return`. This makes a function to return something.
## Anonymous Function
Yes, you can define a function with no name. They're useful while passing a callback function or creating a closure.
```
const anonimo = function () {
console.log('It is an anonymous function')
}
anonimo()
//Expected output: It is an anonymous function
```
## Arrow Functions
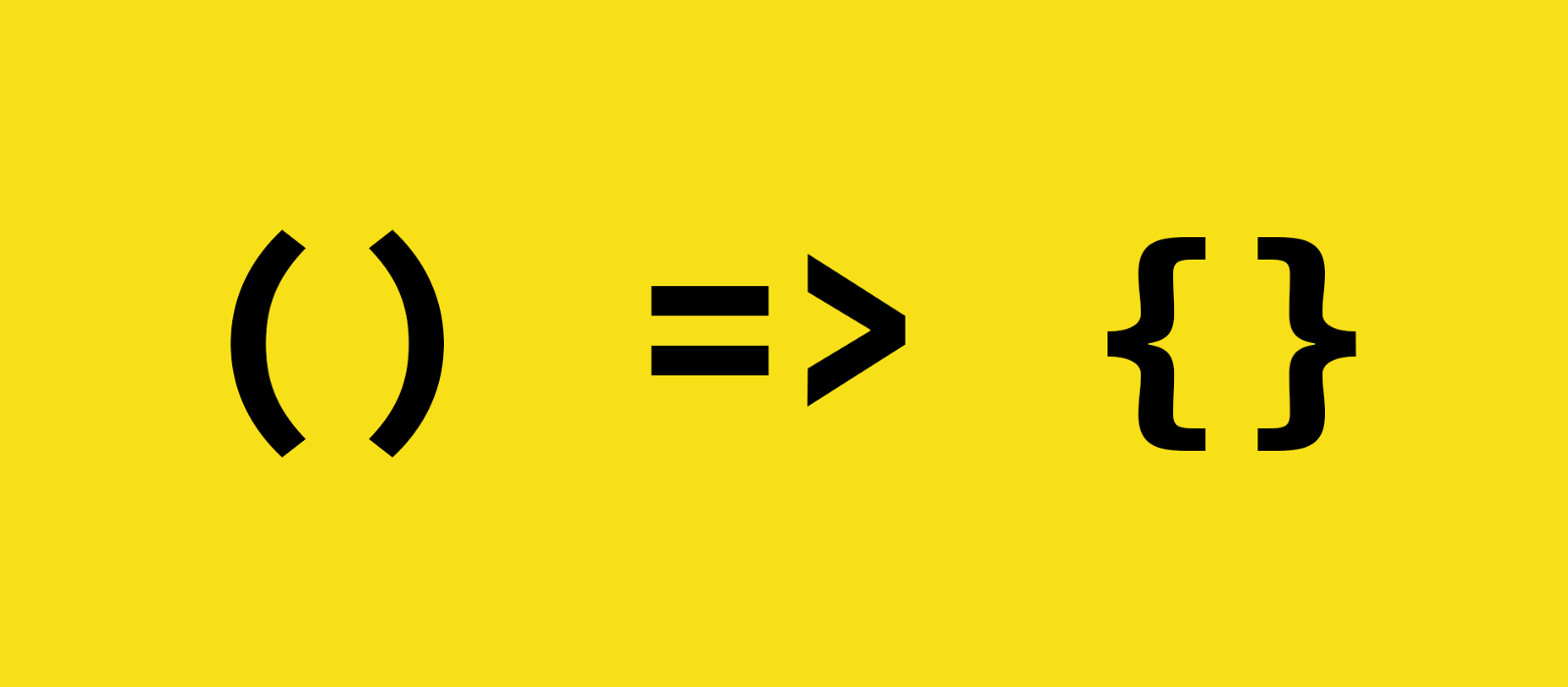
Arrow functions were implemented in **ECMAScript 6**. The main benefit is less code since you can create a function in just one line!
Let's compare an arrow function to a traditional one:
```
//Traditional Function
const traditionalGreeting = function (name){
return `Hi, ${name}`
}
//Arrow Function
const arrowGreeting = (name) => `Hi, ${name}`
traditionalGreeting('Maria')
//Expected output: Hi, Maria
arrowGreeting('Axel')
//Expected output: Hi, Axel
```
See how we can create the same traditional function in just one code line. If you'd like me to write a post about arrow functions let me know in the comments section.
## Function Scope
When you declare a variable inside a function, you can't access to it from anywhere outside. Let's see this example which raises a number to the second power
```
const funcScope = secondPower(numero) {
const power = 2
return numero * power
}
//Here you can't access power
```
Accessing `power` outside the function is not be possible.
## Invocation vs Referencing
To invoke a function means to call it and to execute it. On the other hand, to reference a function is just that, to make your program know there's a function anywhere else.
Imagine you have a function called `myFunc`. So, if you just want to make reference to it, you type `MyFunc`. On the contrary, if you want to invoke it, you type `myFunc()`. Notice the parenthesis `()`. But to understand it better, let's see an example using events.
In this example there's a button and when the user clicks it, it shows an alert saying 'Hello!'.
We have this structure in HTML:
Click Me
In JavaScript we have this:
const sayHello = () => alert('Hello!')
const boton = document.querySelector('button')
boton.addEventListener('click', sayHello)
As you see, in the line `boton.addEventListener('click', sayHello)` the arguments are `'click'` (the event) and `sayHello` (a function). But the latest is just being referenced, since **we don't need it to be executed** unless the user clicks the button.
So far, you have learned the basics of JavaScript functions. The key to become a good developer is practice. So I ask you to **write your own functions** and to practice all you need.
If you liked what you read, you can subscribe my posts. Or you can follow me on [Twitter](https://twitter.com/axlyaguana11). I'll be glad to hear you opinions.
Top comments (1)
Loved this post! JavaScript is my favorite wonky language, but that can make it hard to learn. I think it's good to mention that arrow functions and function expressions are not the same things 1-for-1, and scoping sees differences between the two.
Thanks for the concise write-up!