PayPal is one of the most widely used and easiest payment gateway to integrate in your website or app. Plus it is spread all over the globe, and supports a wide variety of payment options. Together with Stripe, PayPal can take care of almost all your payment requirements, so you don’t have to go all

Get the amazing UI and source code of full Payment app for your Ionic 4 project. Start your own Payment app…store.enappd.com
What is Ionic 4?
You probably already know about Ionic, but I’m putting it here just for the sake of beginners. Ionic is a complete open-source SDK for hybrid mobile app development created by Max Lynch, Ben Sperry and Adam Bradley of Drifty Co. in 2013. Ionic provides tools and services for developing hybrid mobile apps using Web technologies like CSS, HTML5, and Sass. Apps can be built with these Web technologies and then distributed through native app stores to be installed on devices by leveraging Cordova.
So, in other words — If you create Native apps in Android, you code in Java. If you create Native apps in iOS, you code in Obj-C or Swift. Both of these are powerful but complex languages. With Cordova (and Ionic) you can write a single piece of code for your app that can run on both iOS and Android (and windows!), that too with the simplicity of HTML, CSS, and JS.
Ionic 4 and Payment Gateways
Ionic 4 can create a wide variety of apps, and hence a wide variety of payment gateways can be implemented in Ionic 4 apps. The popular ones are PayPal, Stripe, Braintree, in-app purchase etc. For more details on payment gateways, you can read my blog on Payment Gateway Solutions in Ionic 4.
PayPal can be integrated in websites as well as mobile apps. There are different ways of integration of PayPal SDK. In this blog we’ll learn how to integrate PayPal payment gateway in Ionic 4 apps and Ionic 4 PWA.

In this post we will learn how to implement Paypal payments for an Ionic 4 mobile app. We can break down the post in these steps:
Step 1 — Creating an Ionic 4 app. We will use the Ionic 4 side menu starter
Step 2 — Create a PayPal developer account and configure it for app and PWA integration
Step 3 — Use Ionic Native plugin for PayPal to enable payment in mobile apps
Step 4 — Build the app on android to test app payments.
Step 5 — Configure PayPal web integration with Javascript
Step 6 — Run the PWA on ionic serve
to test web payments
Step 1 — Create a basic Ionic 4 app
Creating a basic Ionic 4 app is very easy. Assuming you have all basic requirements installed in your system, run
$ ionic start MyApp sidemenu
This creates your app with titleMyApp
and sidemenu template.
For more details on how to create a basic Ionic 4 app, refer to my blog How to create an Ionic 4 app
With minor modifications, my homepage looks like this.

Step 2 — Configure a PayPal developer account
To configure PayPal payments in your Ionic 4 app, you need to create a business PayPal account. For testing purposes, you can use the Sandbox test accounts. Sandbox testing will look exactly like live payments, but it won’t deduct any money from your account or Credit Card.
To obtain your Sandbox credentials follow the steps below:
- Go to Sandbox Accounts and create a sandbox business and personal test accounts.
- Go to My Apps & Credentials and generate a v.zero SDK sandbox credential and link it to your sandbox test account. You need a Sandbox account to make payments in Sandbox mode of your app. In other words, when your app’s PayPal SDK is running in Sandbox mode, you cannot make payments with an “actual” PayPal account, you need a Sandbox account.
- Go to My Apps & Credentials and create an app. This is the app associated with your payments. A PayPal account can associate multiple such apps with it (you can redirect money from multiple mobile or web-apps in a single PayPal account)

Also note down your Client ID from the app details. This is mostly what you need to integrate PayPal in your app and test payments.

Step 3 — Enable PayPal payments in mobile app
Setup PayPal modules
To install Paypal plugin for Ionic, use
$ ionic cordova plugin add com.paypal.cordova.mobilesdk
$ npm install @ionic-native/paypal
This will install PayPal plugin. Import PayPal into your component using
import { PayPal, PayPalPayment, PayPalConfiguration } from '@ionic-native/paypal/ngx';

You will also need to include PayPal
in the list of providers
in your app.module.ts
file.

PayPal payment function
Invoke the payment function to initiate a payment. As mentioned in Step 2, You will require your client_id
from your PayPal account. (How to get my credentials from PayPal account). This function will be attached to Pay with PayPal button we saw earlier in the app screenshot.
In the payWithPaypal()
function, we will start by initializing the PayPal
object with the PayPal environment (Sandbox or Production) to prepare the device for processing payments. Βy calling the prepareToRender()
method we will pass the environment we set earlier. Finally, we will render the PayPal UI to collect the payment from the user by calling the renderSinglePaymentUI()
method.
Complete paypal.page.ts
file will look as follows
Notice the PayPalEnvironmentSandbox
parameter. This is used for Sandbox environment. For production environment, it will change to PayPalEnvironmentProduction
. Of course, do not forget to replace YOUR_SANDBOX_CLIENT_ID
with your Sandbox Client ID from Step 2.
Also notice, for the sake of a sample, we have taken PaymentAmount
andcurrency
as static in the logic, but these can be easily dynamic as per your app’s requirement.

Once payment is done, PayPal SDK will return a response. A sample sandbox response is shown in the gist above. One can use this response to show appropriate Toast or Alert to app users.
Here’s the HTML template for the PayPal App integration page
Step 4 — Build android app and test payments.
To build the app on android, run the following commands one after another
$ ionic cordova platform add android
$ ionic cordova prepare android
$ ionic cordova run android
The final command will run the app on either default emulator, or an android device attached to your system. Once you click the Pay with PayPal button, you will see the PayPal payment screens

You can choose to
- Pay with PayPal — using your PayPal account, OR
- Pay with Card — This will use your’s device’s camera to help read your credit card (to avoid typing your card number, expiry date etc)
- Pay with PayPal
You will need to login to your Sandbox Account to make a payment (because, remember, you are in a sandbox environment)

Once you are logged in, you’ll see the checkout options

Select one option, and pay for the dummy amount.
2. Pay with Card
In this case, your apps’ camera will open up to scan your card.

Once it is done scanning, it will confirm the card number, expiry date and ask for your CVV details etc. Lastly, it’ll show you a confirmation screen, and you proceed to pay the amount.

In both payment cases, you should receive a successful payment response similar to the one shown earlier in Step 3, or like the following

This completes the Mobile app part of PayPal payment.
Step 5 — Configure PayPal web integration
Since we implemented PayPal in the Ionic 4 app using a native plugin, it won’t work in browser environment. So we can’t use the plugin in an Ionic Progressive Web App (PWA). But we can use the PayPal front-end Javascript SDK in such case.
Warning : In websites or PWA, it is recommended to use payment gateway functions in back-end, because front-end implementation can reveal your client-ID, secret etc
PayPal web implementation page
For PWA implementation, I have created a paypal-web
folder in the app, and attached the route in the sidemenu.

For website front-end implementation, PayPal provides Payment Buttons (the yellow button in the above image). These are pre-configured PayPal buttons + functions, attached to a JS file we import in our PWA’s index.html
Import PayPal Script
To start, import the PayPal JS SDK in your index.html
like this
<script src="https://www.paypal.com/sdk/js?client-id=sb&currency=USD"></script>
Now, PayPal official documentation tells you to code the remaining part of the logic in index.html itself. But the default implementation is good for two reasons
- Ionic app takes time to compile and load in the webview environment, so the render function cannot find the button container
- We need to pass variables like amount, currency etc to the functions. So it makes more sense to keep the functions inside the page.ts file of PWA
Render Payment Buttons
In the HTML template, we replace the ion-button
with
<div id="paypal-button-container"></div>
This id
is used to identify the button, and attached correct render and payment functions to the button.
In the logic for web implementation, we attach createOrder
and onApprove
functions to the PayPal buttons. The web-implementationpage.ts
file looks like following
Notice that the .render(‘#paypal-button-container’)
part is actually what renders the button in the webpage.
onApprove
function carries out the success or error part after a transaction. Here, you can call your server with a REST API and save a successful payment in your DB.
You can actually try different styles of payment buttons at PayPal Payment Button demo page
Step 6 — Test web/PWA payments
When you click on the Payment Button, PayPal script automatically pops-up a modal with required functionality

Once you login with your Sandbox account, you’ll see the same options as you saw in the app’s implementation
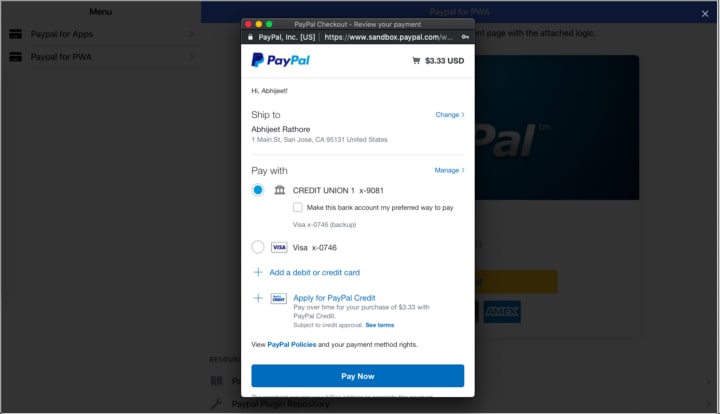
Select the appropriate method, and your payment is done. The response object will look like this

You can easily use the response to determine if the payment was successful.
Ionic 4 Full Payment app Starter - Enappd | Ionic, React native, Firebase themes, templates and…Get the amazing UI and source code of full Payment app for your Ionic 4 project. Start your own Payments app…store.enappd.com
Going Live
After testing on app and PWA, when you want to go live, you will need to perform following steps
- Copy the
production
client-ID from your PayPal account and use it in your app

- In app implementation, change the
PayPalEnvironmentSandbox
toPayPalEnvironmentProduction
inprepareToRender
function - In web-implementation, change the script import with
<script src=”https://www.paypal.com/sdk/js?client-id=LIVE_CLIENT_ID"> </script>
You’re all set now to make and accept payment from your Ionic 4 app and PWA. Go enjoy, homie !

Conclusion
In this post, we learnt how to integrate PayPal in an Ionic 4 app, as well as in an Ionic 4 progressive web app. Testing can be performed easily using Sandbox accounts, and we can go live by simply changing sandbox client-ID with live-ID.
Stay tuned for more Ionic 4 blogs !
FOUND THIS POST INTERESTING ?
Check out my other post on Ionic 4 Stripe payment integration — for Apps and PWA
Also check out our other blog posts related to Firebase in Ionic 4, Geolocation in Ionic 4, QR Code and scanners in Ionic 4 and translations in Ionic 4
NEED FREE IONIC 4 STARTERS?
You can also find free Ionic 4 starters on our website enappd.com
You can also make your next awesome app using Ionic 4 Full App
Top comments (0)