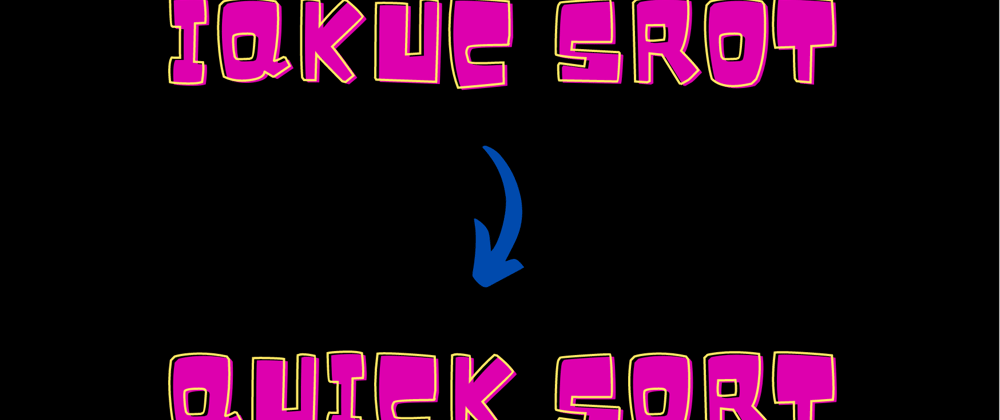
Hello Everyone Today I am going to show you how to write QuickSort Algorithm in Javascript.
QuickSort is a Divide and Conquer algorithm. It picks ...
For further actions, you may consider blocking this person and/or reporting abuse
isn't the point of quicksort that you don't need extra memory? left and rightArray aren't necessary imo.
can you show this correction in code as i am not getting the point😆
Sure,
So this one is memory efficient
Tbf - Although I agree with you in "the real world" I read this as "How to create a quicksort algorithm using Javascript" - Which this article does.
It is not a "How to sort an array using Javascript" to which your comment is related.
This is by far the best implementation of quick sort (in terms of simplicity and comprehension) that I have seen. Thank you very much.
Thank you sir
Good use of destructuring with recursion.
maybe u meant spread with recursion :)
Maybe you meant spread with recursion? 🙂
Yeah But Data Structure is important because Understanding the logic of One algorithm can help you to write more algorithms related to your problems
Brother its just a Simple Quick sort algorithm
Code for learning purpose
I didn't said it is better than sort method or any other sorting Algorithm
It is a just part of the DSA which I showed here and as I am learning Javascript also
So, for practice purpose I used Javascript to demonstrate the implementation of this Algorithm.
That's a narrow minded thinking. If there are 100 thousand elements you would ha e to resort to a proper algorithm.
😂
legand 😂
You have an objectively better method, never reinvent the wheel
Yeesss... It is more quick i think 😄
Yeah I also use Array.prototype.sort method and it is easy to use and good