This is a continuation of Getting started with NuxtJS / Content module.
Add Markdown files to Nuxt/Content
We added the Nuxt/Content
module in:
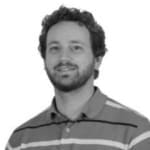
Getting started with NuxtJS / Content module
Adam Miedema ・ Jan 12 ・ 3 min read
Now, in this segment, we'll add some Markdown files and then import them into a dynamic Nuxt page where Nuxt/Content will then convert the Markdown to HTML and post to the page.
First, we need to add a new folder called content
to the project's root directory.
Nuxt/Content will look in this directory to find and grab Markdown files.
Within the content
directory, I am going to add another directory titled 'documentation'. The reason for this, is that it may be very possible that you have different types of files that you'll want to keep organized as well as include as different indices on your Algolia account.
For instance, with docs.cleavr.io, we have a documentation section and a guides section that we keep separate from each other as well as group separately within the search results.
Within the documentation
folder, I'll add the doc-example.md markdown file which contains some random content as well as YAML page meta data at the top.
Create a dynamic page
In the pages
directory, we will add a dynamic page. Which is, a page template, more-or-less, that Nuxt creates dynamic routes based on.
I'll add a page titled _doc.vue to the pages
folder.
Notice that the page starts with and underscore, "_", character. This lets Nuxt know that this page's route will be dynamically generated.
We'll use this dynamic route feature to have Nuxt automatically create a page for each of our markdown content pages. This way, when we are adding or removing markdown files to content/documentation
, we don't have to worry about also creating html pages for the docs. It will dynamically be created for us using this method.
The contents of _doc.vue
is pretty simple.
<template>
<div>
<h1 class="text-3xl font-semibold text-gray-9000 pb-8">
{{ doc.title }}
</h1>
<NuxtContent :document="doc" />
</div>
</template>
<script>
export default {
async asyncData( { $content, params } ) {
const doc = await $content('documentation', params.doc ).fetch()
return { doc }
}
}
</script>
Notice the async
function.
async asyncData( { $content, params } ) {
const doc = await $content('documentation', params.doc ).fetch()
return { doc }
}
Here, we are calling the content module that will look at the documentation
directory for any files that match params.doc
- which is the route name that we are pulling based on the URI.
Such as, if the url is example.com/doc-example
, then the params.doc
value will equal doc-example
. Nuxt/content will then see if there is a file by that name in the content/documentation
directory. If there is, then the doc
data will be passed to the <NuxtContent :document="doc" />
component that is called in the <template>
section.
Add some styling to the generated html from the markdown files
Nuxt/Content wraps some classes around the html that it generates from parsing the markdown file. But, by default, is nothing pretty to look at...
You can choose to tap into the applied classes and work your CSS magic.
Or, you can take the lazy approach and install Tailwind Typography to do the styling for you. Of course, this is the route I choose. 😉
Add Tailwind Typography dependency to package.json
In the package.json file, add in the following dependency:
"dependencies": {
"@tailwindcss/typography": "^0.2.0"
},
Then, in your terminal under your project's root directory, run the following command.
yarn install
This will install the dependency you just added.
Add tailwind.config.js file
On the project's root, add a new file tailwind.config.js.
Within the file, add
module.exports = {
purge: [],
darkMode: false,
theme: {
typography: {
default: {
css: {
'code::before': {
content: '""',
},
'code::after': {
content: '""',
},
},
},
},
extend: {},
},
variants: {
extend: {},
},
plugins: [
require(`@tailwindcss/typography`)
]
}
Notice we are requiring the plugin for @tailwindcss/typography
.
You may also be wondering about the code::before/after portion. This is for if you use back-ticks in markdown to display code snippets, to remove the back-ticks. You can remove this portion if you don't mind having the back-ticks.
Add 'prose' class to converted markdown
To apply Tailwind Typography, we'll simply add the prose class to the NuxtContent
component on the _doc.vue
file.
<NuxtContent :document="doc" class="prose" />
Voila!
Our converted markdown has been pretty-fied! 😍
Following along? View the GitHub Repo - phase 3 to view the code for this tutorial.
You can also view the full video tutorial series playlist on YouTube.
Looking for a tool to manage your VPS servers and app deployments? Check us out at cleavr.io
Top comments (0)