With our Bold Reports platform, you can easily embed reporting components in projects to create, bind data to, view, and export pixel-perfect, paginated reports.
In this blog, I will walk you through the integration of our Angular Report Viewer component into an Angular CLI application. The Angular CLI application will render reports in a browser, and the processing of the report is handled using a Web API application.
If you are using an earlier version of Bold Reports for Angular (v3.3.32), then refer to the Getting Started tutorial for earlier versions.
Prerequisites
- Node JS (version 8.x or later)
- NPM (v3.x.x or higher)
- A browser (Chrome, Edge, Firefox, etc.)
Install the Angular CLI
The Angular CLI is a command-line scaffolding tool used to initialize, develop, and maintain Angular applications directly from a command shell.
Run the following command to install the Angular CLI globally.
npm install -g @angular/cli@latest
Create a New Application
To create a new Angular application, run the following command in the command prompt.
ng new project-name
E.g.: ng new angular-reportviewer
In the command prompt, add the Angular routing to your application by entering “y” in the prompt window. Then select the Enter key.
Now, choose the CSS stylesheet format using the arrow keys and then select Enter. The Angular CLI installs the required Angular npm packages and other dependencies.
Choosing CSS stylesheet format
Let’s dive into the integration of the Bold Reports Angular Report Viewer component.
Configure Bold Report Viewer in Angular CLI
- To configure the Report Viewer component, change the directory to your application’s root folder.
cd project-name
E.g.: cd angular-reportviewer
- Then, install Bold Reports typings by executing the following command. The Bold Reports typings package contains the TypeScript definitions for Bold Reports Embedded Reporting components.
npm install --save-dev @boldreports/types
- To install the Bold Reports Angular library, run the following command.
npm install @boldreports/angular-reporting-components --save-dev
Open the application in an editor. In this blog, I am using Visual Studio Code for editing purposes. You can use any editor.
Now, let’s register the @bold-reports/types under the typeRoots array and add the typings, jquery, and all to the tsconfig.app.json file.
{
...
...
"compilerOptions": {
...
...
"typeRoots": [
"node_modules/@types",
"node_modules/@boldreports/types"
],
"types": [
"jquery",
"reports.all"
]
},
...
...
}
Register the typeRoots and types
- The Report Viewer requires a window.jQuery object to render. Import jQuery in the src > polyfills.ts file as in the following code snippet.
import * as jquery from 'jquery';
let windowInstance = (window as { [key: string]: any });
windowInstance['jQuery'] = jquery;
windowInstance['$'] = jquery;
Add the Theme Reference
A theme gives life to any Angular component. Open the angular.json file from your application’s root directory and reference the Report Viewer component styles file, bold.reports.all.min.css, under the styles node of the projects section as in the following code snippet.
"styles": [
"styles.css",
"./node_modules/@boldreports/javascript-reporting-controls/Content/material/bold.reports.all.min.css"
],
Note: In the previous code, the Material theme is used. You can modify the theme based on your application. Reference the following syntax:
./node_modules/@boldreports/javascript-reporting-controls/Content/[theme-name]/bold.reports.all.min.css
In Bold Reports, the report processing and rendering in the browser will be handled using the server-side Web API and client-side HTML page, respectively.
Adding Report Viewer Component in the Client-Side
To add the Report Viewer component on the client-side:
- Open the module.ts file and import the viewer module.
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { BoldReportViewerModule } from '@boldreports/angular-reporting-components';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
// Report viewer
import '@boldreports/javascript-reporting-controls/Scripts/bold.report-viewer.min';
// data-visualization
import '@boldreports/javascript-reporting-controls/Scripts/data-visualization/ej.bulletgraph.min';
import '@boldreports/javascript-reporting-controls/Scripts/data-visualization/ej.chart.min';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
BoldReportViewerModule,
AppRoutingModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
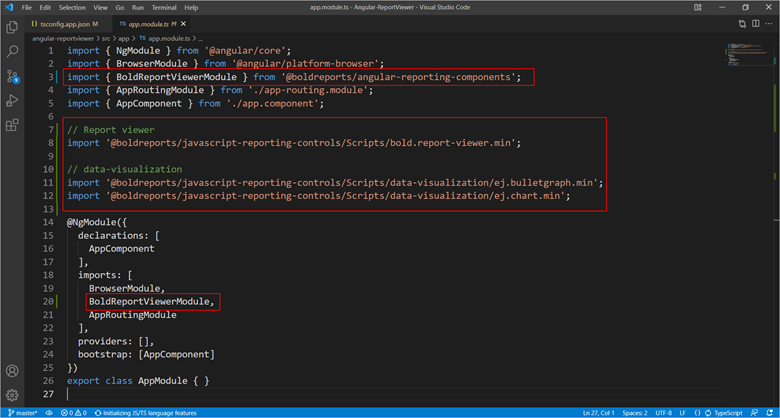Import Viewer Module
- Open the html file and reference the following scripts in the tag.
<!-- Data-Visualization -->
<script src="./../node_modules/@boldreports/javascript-reporting-controls/Scripts/common/ej2-base.min.js"></script>
<script src="./../node_modules/@boldreports/javascript-reporting-controls/Scripts/common/ej2-data.min.js"></script>
<script src="./../node_modules/@boldreports/javascript-reporting-controls/Scripts/common/ej2-pdf-export.min.js"></script>
<script src="./../node_modules/@boldreports/javascript-reporting-controls/Scripts/common/ej2-svg-base.min.js"></script>
<script src="./../node_modules/@boldreports/javascript-reporting-controls/Scripts/data-visualization/ej2-lineargauge.min.js"></script>
<script src="./../node_modules/@boldreports/javascript-reporting-controls/Scripts/data-visualization/ej2-circulargauge.min.js"></script>
<script src="./../node_modules/@boldreports/javascript-reporting-controls/Scripts/data-visualization/ej2-maps.min.js"></script>
Let’s look at the scripts and style sheets required to render the Report Viewer.
- ej2-base.min.js- Render the gauge item. Add these scripts only if your report contains the gauge report item.
- ej2-data.min.js- Render the gauge item. Add these scripts only if your report contains the gauge report item.
- ej2-pdf-export.min.js- Render the gauge item. Add these scripts only if your report contains the gauge report item.
- ej2-svg-base.min.js- Render the gauge item. Add these scripts only if your report contains the gauge report item.
- ej2-lineargauge.min.js- Renders the linear gauge item. Add this script only if your report contains the linear gauge report item.
- ej2-circulargauge.min.js- Renders the circular gauge item. Add this script only if your report contains the circular gauge report item.
- ej2-maps.min.js- Renders the map item. Add this script only if your report contains the map report item.
- ej.chart.min.js-Renders the chart item. Add this script only if your report contains the chart report item.
- bold.report-viewer.min.js-Mandatory to render the Bold Report Viewer.
Open app.component.html and initialize the Report Viewer using the following code snippet.
<bold-reportviewer id="reportViewer_Control" style="width: 100%;height: 980px">
</bold-reportviewer>
Open app.component.ts and initialize the service URL and report path. Then, include the constructor to initialize the report viewer properties.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'reportviewerapp';
public serviceUrl: string;
public reportPath: string;
constructor() {
// Initialize the Report Viewer properties here.
}
}
Include constructor to initialize the Report Viewer Properties
Set Report Path and Web API Service
Next, let’s set the report path and Web API service. This is where the RDL report is processed and rendered in the browser using the Web API service.
In this blog, I am using the render path unit, which requires the service URL and report path. The Web API service is hosted as an Azure web app.
The report path property sets the path of the report file, and the report service URL property specifies the report Web API service URL.
To render the report, set the reportPath and reportServiceUrl properties of the Report Viewer in the constructor as follows.
constructor() {
this.serviceUrl = 'https://demos.boldreports.com/services/api/ReportViewer';
this.reportPath = '~/Resources/docs/sales-order-detail.rdl';
}
Set reportPath and reportServiceUrl properties in Constructor
I am using the sales-order-detail.rdl
report from the demo server location. This file is located in the resources/docs/ path.
Open the app.component.html file to set the reportPath and reportServiceUrl properties of the Report Viewer as in the following.
<bold-reportviewer id="reportViewer_Control" [reportServiceUrl] = "serviceUrl" [reportPath] = "reportPath" style="width: 100%;height: 980px">
</bold-reportviewer>
Set properties in Report Viewer
Then save the application.
Serve the Application
- To serve the application:
- Go to the workspace folder (report-viewer). Launch the server by using the following CLI command with the –open option.
ng serve --open
The ng serve command serves the application and the –open option automatically opens your browser to http://localhost:4200/.
Visit:https://www.boldreports.com/contact/How to Add Report Viewer to an Angular Application?
Conclusion
In this blog, we learned how to integrate the Report Viewer component into an Angular application. To explore further, go through our sample reports and Bold Reports documentation.
If you have any questions, please post them in the comments section. You can also contact us through our contact page, or if you already have an account, you can log in to submit your support question.
Bold Reports now comes with a 15-day free trial with no credit card information required. We welcome you to start a free trial and experience Bold Reports for yourself. Give it a try and let us know what you think!
Stay tuned to our official Twitter, Facebook, LinkedIn, Pinterest, and Instagram pages for announcements about upcoming releases.
Top comments (1)
Thanks. I am using Angular 18.2.8.
I have successfully completed the previous steps, but I don't have polyfills.ts in src. Now I am blocked to continue with the following steps. What to do?