According to the Documentation, Cloud Firestore is a flexible, scalable database for mobile, web, and server development from Firebase and Google Cloud Platform.
Like Firebase Realtime Database it keeps your data in sync across client apps through realtime listeners and offers offline support for mobile and web so you can build responsive apps that work regardless of network latency or Internet connectivity, this is just amazing if you ask me, it solves the whole essence of PWA’s. You may ask how, well
Cloud Firestore caches data that your app is actively using, so the app can write, read, listen to, and query data even if the device is offline. When the device comes back online, Cloud Firestore synchronizes any local changes back to Cloud Firestore.
Cloud Firestore also offers seamless integration with other Firebase and Google Cloud Platform products, including Cloud Functions.
However, Cloud Firestore is still in beta release but if you’re enthusiastic (like me) and want to get hands on immediately, then this tutorial will help you get started with basic read, write, update and delete operations.
What you’ll learn
If you don’t know these yet then, you’ll
· Gain a better understanding of Firebase and Firestore
· Learn how to add firebase to your project
· Learn the FireStore database model and structure
· Learn how to write to Cloud FireStore
· Learn how to read from Cloud FireStore
· Learn how to update an existing data in Cloud FireStore
· Learn how to delete data from Cloud FireStore
· Learn how to listen for changes in realtime
· Learn how to combine all these features to create a Phonebook
As usual I like to prepare my tutorials to be very relative and not so abstract, so i won’t be talking too much, let’s start building something with FireStore.
Create a new android studio project and connect it to firebase. Once again there are two ways you can do this depending on the version of Android studio you’re on. If you’re using the latest version of Android Studio (version 2.2 or later), i recommend using the Firebase Assistant to connect your app to Firebase. The Firebase Assistant can connect your existing project or create a new one for you and automatically install any necessary gradle dependencies. Here’s how you can do it
To add firebase to your app with Firebase Assistant in Android Studio:
· Click Tools > Firebase to open the Assistant window.
· Click to expand one of the listed features (for example, Analytics), then click the provided tutorial link (for example, Log an Analytics event).
· Click the Connect to Firebase button to connect to Firebase and add the necessary code to your app.
That’s it!
If you are using the earlier versions of Android Studio or you prefer not to use the Firebase Assistant, you can still add Firebase to your app using the Firebase console.
To manually add Firebase to your app you’ll need a Firebase project and a Firebase configuration file for your app.
- Create a Firebase project in the Firebase console, if you don’t already have one. If you already have an existing Google project associated with your mobile app, click Import Google Project. Otherwise, click Create New Project.
- Click Add Firebase to your Android app and follow the setup steps. If you’re importing an existing Google project, this may happen automatically and you can just download the config file.
- When prompted, enter your app’s package name. It’s important to enter the package name your app is using; this can only be set when you add an app to your Firebase project.
- At the end, you’ll download a google-services.json file. You can download this file again at any time.
- If you haven’t done so already, copy this into your project’s module folder, typically app/.
Then you can add any of the Firebase libraries you’d like to use in your project to your build.gradle file, it is good practice to always add com.google.firebase:firebase-core.
This guides are according to the firebase documentary however, if all of this is still not clear to you, you can always find more content on how to setup your firebase project here
Now that you have your firebase project set up, let’s add the dependency for FireStore. As at the time of writing this tutorial, the latest version is compile ‘com.google.firebase:firebase-firestore:11.4.2’ so add it to your app level build.gradle file dependency block. It might have changed depending on when you’re reading this so always check the Docs for the latest dependency and sync.
Before we proceed let’s take a moment to understand the FireStore model
Cloud Firestore is a document database; it stores all your data in documents and collections. Documents operates more like containers of fields(key-value pairs) of diverse data types however a document cannot contain other documents but can point to a sub-collection. Collections on the other hand operate also like documents but in its case, it’s a container of documents hence, it can’t contain anything else but documents.
From the above image, “Users is a collection of 3 documents “Joe”, “Tammy and “Vic”. Document Joe however, points to a sub-collection “Workouts which in itself has 3 documents (“Yoga level 2”, “Beginner Jogging”, and “7-minute thighs”). Then document “7-minute thighs further more points to a sub-collection “History which in turn holds 3 more documents etc.
This is deep nesting and it may seem a little bit deep but it is very efficient for query performance in both simple complex query structures. With this idea of how Firestore works, let’s dive into the firebase project we created earlier and start writing some code.
What are we building?
For the purposes of understanding the basics of Firestore in the best simple way, we’ll be building a Phone Book database that stores contacts with 3 fields (Name, Email and Phone). We’ll demonstrate how to write, read, update and delete data from the database.
Let’s get cracking…
Usually we would have started with setting up EditTextViews in the layout files but we won’t be taking inputs from users yet so we’ll just supply all the data we want to store to the database from our java files….for now. So open up MainActivity and initialize Firestore
You may have noticed I also initialized a Textview object and some String variables there; we’ll use the Textview to read data from the database and the String variables as “keys for storing data into our document.
Considering the model we’ll be working with, it is best to think of the Phonebook we are building as the collection, so let’s add/save a newContact to our PhoneBook.
Here we first created a method addNewContact(), the method will create the newContact that we’d want to save in the PhoneBook and then pass the fields to it. Next we need to specify in which document we want to save the contact. We do this by extending the Firestore instance we created to accommodate both the collection and the document.
“db.set (newContact) this creates the document if it doesn’t already exist and also creates the PhoneBook collection so as to store the data in the appropriate path according to the db path we initialized.
Then we add an OnSuccess/FailureListeners to make a Toast and keep us informed if the contact was registered successfully or if the registration failed.
Looks like we’ve got it all set up, before we run the app, we’ll need to head on over to the firebase console and tweak our RULES Tab a little bit to allow us read and write to the database because at default, nobody can read or write to it. So go to your firebase console, open the project and make sure you select Firestore in the database tab
Then open the RULES Tab, by default it looks like this
Then add this block of code to override the default security settings and gain external read and write access to your database,
Match/your-collection/{anything=}{
allow read, write: if true;
}
Now your RULES Tab should look like this
This is a terrible idea security wise, if you’re working on a real project or something proprietary, you would definitely want to properly authenticate users and define your security measures clearly. This tweaking is only serving the purpose of this tutorial.
Haven set up the security rules in the console, publish it and wait for a few minutes after which you can now run the app and go back to the DATA Tab on the console. Hurrray!! App works…. You’ve just stored your your data to FireStore.
Now let’s try and read the data we’ve stored in the database to a TextView.
READ | First we create ReadSingleContact() method that’ll read the data from the database and pass the data to the TextView object we earlier initialized for this Purpose.
If we run the app, it populates the TextView object with the information stored on the database as is expected.
UPDATE | Next we’ll try to update the already stored Contact informations
Now running the app will update the earlier stored data with the newly provided values in the UpdateData() method.
In the DATA Tab on your firebase console, you’ll find that the changes have been updated.

And if you run the ReadSingleContact() method again, it’ll update the TextView with the current details.
REALTIME UPDATE
Next up, we listen for update on the document “Contacts”, if we update these changes without running the code to Read (fetch) data from the database (ReadSingleContact()), we might not be able to see the changes in the UI so we’ll create a method that listens for update in realtime and makes a Toast to notify users of the changes in the database.
Build the Phonebook app
Now let’s take input from users instead of just adding and updating the database from the studio. I’ll go ahead and create view objects on the mainActivity layout file to take Inputs for the 3 fields (name, email and phone) such that we can save whatever data the user types into any of these fields to the PhoneBook.
We’ve already done all the major work required so just create the view objects in the layout file and initialize them in the MainActivity.java file. Here’s my activity_main.xml
Next we initialize the views in the java file
Notice the additions of the view objects as compared to the code gist we had under the WRITE heading. Then to save the details coming from this Views to the database, we’ll just tweak our newContact.put() method a little bit to capture its value from the EditTextViews. So we’ll open up the addNewContact() method and edit it to capture these changes
Then we need to set the save button to call this method, so lets set an onClick listener to the button and call the method in it.
save.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
addNewContact();
}
});
Then finally let’s update the TextView with any changes we make to the database in realtime, such that if a user fills up the input fields and clicks the save button, the TextView will update the changes in realtime wether there’s internet Connectivity or not.
And that’s it!! At this point, Users can input their details and save it to the database as well as have that same details read from the database and displayed on their screen.
DELETE | Finally lets perform the delete operation to delete the Contact from the database.
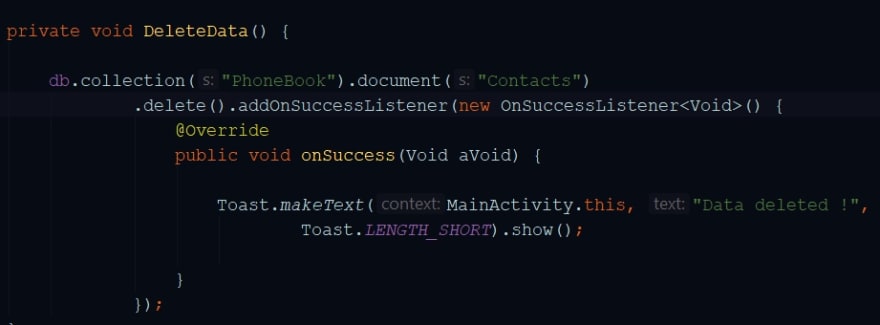
This method successfully deletes the contact from the PhoneBook database. So congratulations if you followed up to this point, this means you can now perform basic operations on one of the most sophisticated and newest online database in the world. This is just a glimpse of what you can do with Cloud FireStore, there are a lot more features and operations to perform. My intention is just to get you started with the basics.
Thanks for reading, you are welcome to make contributions and ask for clarifications anytime you deem fit. Like always, I’m available (twitter @kenny_io) to provide more clarifications and answer your questions if you have any.
The source code is also available on github if you need it.
Top comments (0)