C is a general-purpose programming language that was designed in the early 1970s by Dennis Ritchie at Bell Labs.
C is a high-level programming language that is used for developing system and application software. It is a procedural language, which means it follows a set of procedures or functions. C has a simple syntax and is easy to learn.
Programs written in C are efficient and fast, and it is widely used in the industry for developing operating systems, device drivers, embedded systems, and many other applications. C programming language has influenced many other programming languages like C++, Java, and Python.
This article is a beginner's guide to programming in C language. It provides a step-by-step guide to create a simple "Hello, World!" program, which is usually the first program a beginner creates in any programming language.
Getting Started with C Programming
Before creating our first program with C, we need to make sure that we have a C compiler installed on our machine. A compiler is a software that translates the source code written in a programming language into machine code that the computer can understand.
In the case of C, there are several popular compilers available for different operating systems, such as GCC for Linux, Clang for macOS, and Microsoft Visual C++ for Windows. Depending on your operating system, you can choose the one that suits you best. Once you have installed a C compiler, you are ready to start writing your first C program.
Before creating your first program with C, it is important to set up the necessary development environment. This includes installing a text editor or an integrated development environment (IDE) and a C compiler on your computer. The text editor or IDE will be used to write your code while the compiler will translate your code into machine-readable binary files that can be executed by the computer.
There are several text editors and IDEs available, including Visual Studio Code, Sublime Text, and Code::Blocks. For the C compiler, you can choose from GNU Compiler Collection (GCC), Clang, or Microsoft Visual C++. Once you have installed the necessary software, you are ready to start creating your first program with C.
Can I code in C language without install? Yes, with an C online compiler
Yes, you can! With Lightly IDE's C online compiler, you can also code in C language without any installations. There's no need to download a text editor, nor install a development environment for C language.
Simply open Lightly's workspace in your browser and create a C project, and you'll be ready to code.
Once you're finished writing your code, you can compile it into an executable file, which you can run on your computer or distribute to others.
Writing Your First Program
The "Hello World" program is a simple program that outputs the text "Hello, World!" on the screen. It is a classic example used in programming tutorials to demonstrate the basic syntax of a programming language.
The main purpose of this program is to help beginners to get started with programming and familiarize themselves with the process of creating and running a program.
Writing a "Hello World" program in C
In C, this program is simple and consists of just a few lines of code. To create a "Hello World" program in C, you start by including the standard input/output library using the include directive.
Then, you define the main() function, which is the entry point of the program. Within the main() function, you use the printf() function to output the text "Hello, World!" to the console. Finally, you return a value of 0 to indicate the program executed successfully.
With these few lines of code, you have successfully created your first program in C!
Once you have written your program, the next step is to compile and run it. In Lightly IDE, you can simply click the "Run" triangle button to compile and run your program. That's it.
Alternatively, to compile the program in other development environment, you'll need to open a command prompt and navigate to the directory where the program is saved.
Then, type the command gcc -o hello hello.c and press enter. This will compile the program and create an executable file called hello. To run the program, type the command ./hello and press enter. This will run the program and print the message "Hello, world!" to the console.
Congratulations, you have created and run your first program with C!
Understanding C Syntax
As you can see, the syntax of C is relatively simple and straightforward, with several basic elements that form the foundation of the language. These include variables, data types, operators, and control statements. Understanding these basic elements is essential for writing effective C programs.
Variables in C Language
In C programming, variables are used to store values such as numbers, characters, and other data types. Before using them in a program, variables must be declared by specifying their data type, such as int for integers and char for characters.
Here is an example of how to declare variables in C:
int age; // declares an integer variable called age
char grade; // declares a character variable called grade
float price; // declares a floating-point variable called price
Once variables are declared, they can be assigned values using the assignment operator =. For example:
age = 25; // assigns the value 25 to the age variable
grade = 'A'; // assigns the character 'A' to the grade variable
price = 9.99; // assigns the value 9.99 to the price variable
Variables can also be initialized with a value at the same time they are declared, like this:
c
int age = 25; // declares and initializes the integer variable age with the value 25
char grade = 'A'; // declares and initializes the character variable grade with the value 'A'
float price = 9.99; // declares and initializes the floating-point variable price with the value 9.99
### Data Types in C Language
In C programming, a data type specifies the type of data that a variable can store. C has several built-in data types, including integer, floating-point, character, and void. Integers are used to store whole numbers, floating-point data types are used to store decimal numbers, characters are used to store letters and symbols, and the void data type is used to indicate that a function returns no value.
In addition to the built-in data types, C also allows users to define their own custom data types using structures and unions. It is important to declare the correct data type when creating variables in C to avoid errors and ensure that the program runs correctly.
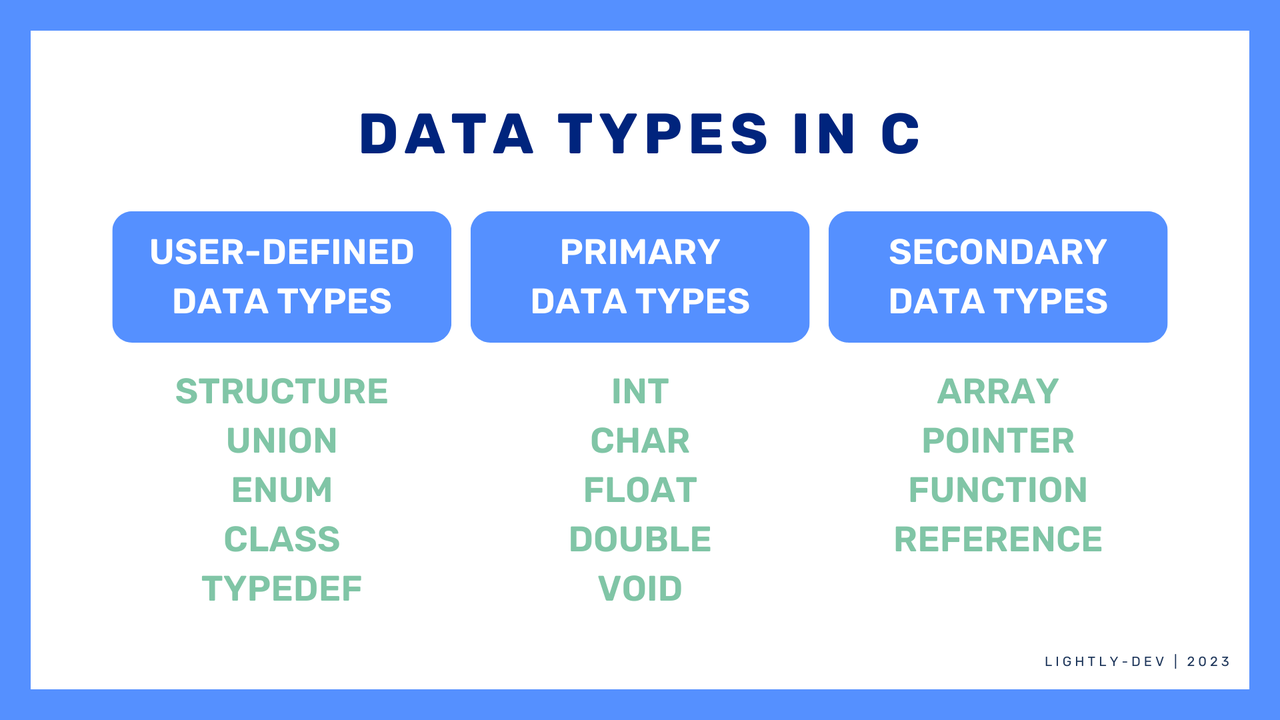
### Operators in C Language
Operators in C are symbols used to perform specific operations on operands. There are different types of operators in C, such as arithmetic, relational, logical, bitwise, and assignment operators. Arithmetic operators are used to perform basic mathematical operations like addition, subtraction, multiplication, and division.
Relational operators are used to compare two values, while logical operators are used to combine two or more conditions. Bitwise operators are used to manipulate bits, and assignment operators are used to assign values to variables. Understanding operators is essential to writing efficient and effective programs in C.
### Control Statements in C Language
Control statements are used to control the flow of execution of a program. There are three main types of control statements in C: decision-making statements, looping statements, and jump statements.
Decision-making statements help the program to make decisions based on a condition. Looping statements help to execute a block of code repeatedly until a condition is met. Jump statements help to transfer the control of the program from one point to another. Understanding these control statements is essential in writing efficient and effective programs.
## Debugging Your Program in C Language
C programming can be challenging, especially for beginners. As you start writing more complex programs, you are likely to encounter a variety of errors. Here are some of the most common mistakes you might encounter while writing C programs:
- Syntax errors: These are caused by incorrect use of keywords, missing semicolons or brackets, incorrect variable types, and other issues that result in C code that cannot be compiled.
- Linker errors: These occur when the linker is unable to find the necessary libraries or functions to link your program.
- Runtime errors: These are errors that occur when your program is running, such as division by zero or accessing an array element that is out of bounds.
- Logic errors: These are errors that occur when your program runs without any errors, but produces unexpected results. They are often caused by incorrect program logic or data processing.
To avoid these errors, it is important to pay careful attention to the syntax of your code and to test your programs thoroughly.
Debugging is an essential part of software development. It is the process of identifying and fixing bugs or errors in your code. There are several tools and techniques available to help developers debug their programs. One commonly used technique is to use print statements to output the values of variables and the flow of control in the program.
Another technique is to use a debugger, which allows developers to step through their code line by line and inspect the values of variables in real-time. Some popular debugging tools for C include GDB, Valgrind, and Clang/LLVM. These tools can help developers identify memory leaks, undefined behavior, and other common programming errors.
That's all for today's article about writing your first program with C. Stay tuned to our blog and check out other interesting articles that we've posted.
Read more: [Saying Hello to the World: Creating Your First Program with C](https://www.lightly-dev.com/blog/hello-world-in-c/)
Top comments (0)