In this post, we’ll learn how to setup Google Login in React Native apps using Firebase. We’ll implement the authentication using react-native-google-signin
npm package, and then test it on Android.
Complete source code of this tutorial is available here — React-Native-google-login (android branch)
I have another blog explaining how to implement Google Login in React Native for iOS, using Firebase.
If you have read the iOS version already, skip the next few sections and jump to Steps for Google authentication directly.
Google login — What and why
There are several reasons why you should use a Google login in your app
- Ease of use — When a new user uses your app, only two buttons clicks are required to login using Google. In other scenario, user will have to type in an email/password and login
- No “forgot password” — When your app uses Google login, the user does not have to worry about forgetting password for your app’s login
- No “verify email” — If you use a custom-email authentication of your own, you will have to verify the email if it is a valid one or not. Google login will always have a valid email/phone number associated.
- Single solution — Google login can allow your users to use single login credentials across multiple devices
- Google integration — If your app uses Google authentication, you can use Google APIs inside your app as well. This can include fetching user tweets etc.
- Trust — Nowadays, people generally trust social logins more over custom email logins. Social logins follows standard privacy protocols and hence are more reliable for information sharing
Authentication in React Native
React Native has been around for around 4 years, and has been very popular among developers for its ease of usage over Swift / Java. Also you get to keep a single source code for both Android and iOS app. What more can a developer ask for!
React Native 0.60 is the latest version (at the time of writing this post), and is much more reliable and robust than previous versions. It also includes breaking changes related to AndroidX, but don’t worry we’ll create an Android test app as well.
There are several ways of Authentication in React Native Apps
- Social logins — Social logins are a popular and easy way of authentication in mobile apps. You must have seen Google, Facebook, Instagram logins in almost all the modern apps. Social logins are easy to use and more reliable for quick integrations.
- Create you own back-end — You can create your own back-end in Node.js, Go, Django or Ruby-on-rails, and connect your app authentication to your own back-end. This method is favored by developers who need full control over the user authentication. But this method is the most time taking one as well.
- Back-end as a Service (BaaS) — You can use pre-built BaaS platforms which allows easy integration of authentication in your apps. Basically, these platforms provide you a ready-made back-end, so you don’t have to make one on your own. Firebase, Parse, Back4App are some BaaS platforms.
Firebase is the most popular among these for mobile apps, which we’ll study in next section
Firebase
Firebase is a Backend-as-a-Service (BaaS) platform. It started as a YC11 startup and grew up into a next-generation app-development platform on Google Cloud Platform. It is getting popular by the day because of the ease of integration and variety of functionalities available on it.
A lot of quick integrations are available with Firebase. Some of these are listed below:
- Real-time database
- Email Authentication
- Social logins
- In-app messages
- Push notifications
- Analytics
- Crashlytics
- Remote config
Firebase is quickly growing to become the most popular mobile app back-end platform.
Firebase Authentication Options
Firebase not only provides ready-made email authentication, but also provides authentication using a variety of social logins. You can see the authentication options available with Firebase

We will use Firebase to store user profile information once the Google login is done. This is the preferred method, as it is reliable for both apps and PWA.
Steps for Google authentication
We will follow these step-by-step instructions to create our React Native app with Google authentication
Step 1: Create Firebase Project and Add android platform
Step 2: Enable Google Sign-In in Firebase project
Step 3: Create a Basic React Native app
Step 4: Install the react-native-google-signin
package for Google Login
Step 5: Implement Google auth functions in RN app
Step 6: Test your app on Android
Step 7: Use Firebase to store user info and handle Auth
So let’s jump right in
Step 0: Create a SHA-1 Signature
This is an important step where many developers commit mistake. That’s why Step 0
This is a way for the app and Firebase to recognize the system from which the app was developed. It is generated based on the system and yourkeystore
file.
Generate the key using following command
$ keytool -exportcert -keystore <<keystore file location>> -list -v
Important : This is where many people commit mistake. The keystore
file in the above command is NOT the default keystore
file of your system. React Native automatically generates a debug.keystore
for your Android project, which resides in Android project folder
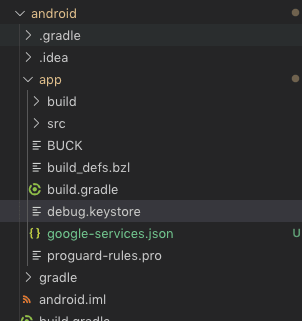
Use this keystore
file location to generate SHA-1 signature. Keep the signature safe for further steps. SHA-1 looks like this
RE:YY:16:06:XX:XX:RR:2C:XX:0D:54:XX:G6:BA:XX:F3:XX:AB:F6:XX
No I want only one debug.keystore in my life ! 😠
If however, you don’t want to include this keystore
and want to generate the signature using default keystore
file, then you need to update the default keystore
file’s location in
android → app → build.gradle → signingConfigs
Enter the correct values insigningConfigs
for your own key
signingConfigs {
debug {
storeFile file('debug.keystore')
storePassword 'android'
keyAlias 'androiddebugkey'
keyPassword 'android'
}
}
Step 1: Create Firebase Project
For authentication, we need a back-end. For this tutorial, Firebase is our back-end, so we need to configure few things in Firebase. First of all we’ll create a new Firebase project and new app inside it.
1.1 If you don’t have an existing Firebase Project
If you already have a Firebase project, skip to step 1.2. Otherwise, create a new Firebase project by going to Firebase Console. Give your project a name and that’s it !

1.2 Create and attach new app to the project
Now that the project is created, create a new Android app in the project dashboard.

Add the SHA1
key you generated in Step 0 and add it in Debug signing certificate SHA-1
field

Just mention the correct package name of your app in the Android package name
input. For me, it is com.rngooglelogin. Give it a nickname to remember.
Next step, download the google-services.json
file and put it in the android/app
folder of your project. You can skip rest of the steps for now. Your app is created now, and you should see it in the dashboard.
Step 2: Enable Google Login in Firebase
Now that your app is connect to Firebase project, we need to go into our Firebase console and enable Google authentication for our app.
Once you’re inside the app’s dashboard, you’re going to go into
Authentication → Sign-In Method → Google
and click the Enable
toggle.

Give a name to for your Auth consent screen and save your web client Id as well. This will be used to integrate the React Native app with Firebase.
Step 3: Create a basic React Native app
First, make sure you have all pre-requisites to create a react-native app as per the official documentation.
At the time of this post, I have React-Native version 0.60
Create a blank react-native app (Replace myApp
with your own name)
$ react-native init RNGoogleLogin
This will create a basic React-native app which you can run in a device or simulator. (let’s run Android)
Let’s run the app in Android using (I’m using a real device)
$ react-native run-android
You’ll see the default start screen
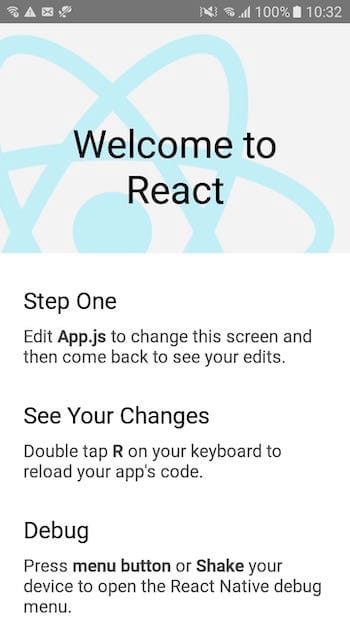
Change the UI of this front page by changing App.js
and the page will look like this. (The Google login button here actually comes after the package integration, as explained in next section)

Step 4: Install the package for Google Login
To enable Google Login, we’ll install a package named react-native-google-signin. This is a widely used package for Google Login, and also very reliable.
4.1 Install package
Install the package using
$ yarn add react-native-google-signin
Link the package with your platforms using
$ react-native link react-native-google-signin
Note: For React Native ≥0.60, you don’t need to manually link most packages with React Native. It is done automatically on app build
4.2 Make sure you have latest SDK and other android packages
You should always try to stay updated with latest Android SDK and other packages. At the time of this post, my Android SDK is 9.0 (Pie) and here’s a list of my other packages

4.3 Add google-services.json file to project
If you haven’t done it yet, download the google-services.json
file from your Firebase project and put it in the android/app
folder of your RN project.
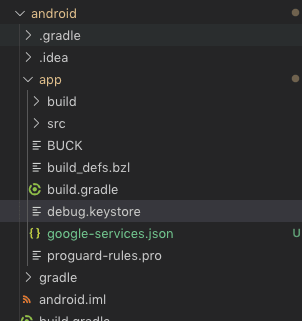
4.4 Update Android configuration files
- Update
android/build.gradle
with latest versions. My configuration is
buildscript {
ext {
buildToolsVersion = "28.0.3"
minSdkVersion = 16
compileSdkVersion = 28
targetSdkVersion = 28
supportLibVersion = "28.0.0"
googlePlayServicesAuthVersion = "17.0.0" // <--- use this version or newer
}
...
dependencies {
classpath 'com.android.tools.build:gradle:3.4.1' // <--- use this version or newer
classpath 'com.google.gms:google-services:4.3.0' // <--- use this version or newer
}
...
allprojects {
repositories {
mavenLocal()
google() // <--- make sure this is included
jcenter()
maven {
// All of React Native (JS, Obj-C sources, Android binaries) is installed from npm
url "$rootDir/../node_modules/react-native/android"
}
}
}
- Update
android/app/build.gradle
dependencies. My configuration looks like this
...
dependencies {
implementation fileTree(dir: "libs", include: ["*.jar"])
implementation "com.android.support:appcompat-v7:23.0.1"
implementation "com.facebook.react:react-native:+"
implementation(project(":react-native-google-signin"))
}
apply plugin: 'com.google.gms.google-services' // <--- this should be the last line
- Check whether
react-native link
linked the native module inandroid/settings.gradle
you should have
...
include ':react-native-google-signin', ':app'
project(':react-native-google-signin').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-google-signin/android')
- For older versions of React Native, in
MainApplication.java
you should have the following code. For my version 0.60, there is no need to editMainApplication.java
import co.apptailor.googlesignin.RNGoogleSigninPackage; // <--- import
public class MainApplication extends Application implements ReactApplication {
......
@Override
protected List<ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
new MainReactPackage(),
new RNGoogleSigninPackage() // <-- this needs to be in the list
);
}
......
}
Step 5: Implement Google auth functions in RN app
Now that the app is all connected to Google Auth, let’s write the actual code to call login / logout etc functions.
First of all, I have created a separate file LoginController.js
and imported it in App.js
, just to maintain a good code structure.
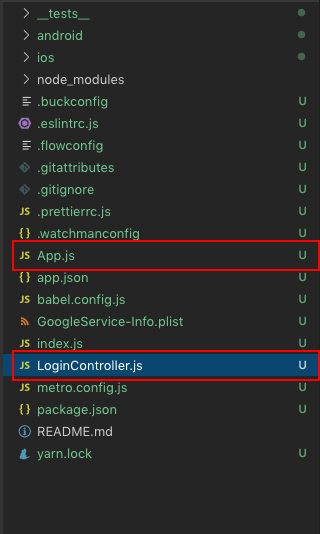
My App.js
looks like following (nothing fancy, I’m not even gonna waste a gist here)
import React, { Fragment } from 'react';
import LoginController from './LoginController';
const App = () => {return (<LoginController/>);};
export default App;
Yes, that’s it 😆
5.1 Implement Google Login button
In the LoginController.js
, I add Google login button using following code
import { GoogleSignin, GoogleSigninButton, statusCodes } from 'react-native-google-signin';
.....(inside render)
<GoogleSigninButton
style={{ width: 192, height: 48 }}
size={GoogleSigninButton.Size.Wide}
color={GoogleSigninButton.Color.Dark}
onPress={this._signIn}
disabled={this.state.isSigninInProgress} />
....
This shows the beautiful Google Button as per their marketing guidelines
5.2 Configure Sign In on App start
Before calling signIn
method, we need to call configure
method, which sets the parameters for Google Auth. You can call this method in componentDidMount
GoogleSignin.configure({
scopes: ['https://www.googleapis.com/auth/drive.readonly'], webClientId: 'XXXXXX-qXXXXXn7umiXXXXXXe1ekgubrXXe.apps.googleusercontent.com',
offlineAccess: true,
hostedDomain: '',
loginHint: '',
forceConsentPrompt: true,
accountName: '',
iosClientId: 'XXXXXX-krv1hjXXXXXXp51pisuc1104q5XXXXXXe.apps.googleusercontent.com'
});
Let’s look at this in more detail
- scopes: This tell what API you want to access on behalf of the user, leave it blank for just email and profile access. For more API access, you might have to get your app verified, or you’ll see this warning when logging in
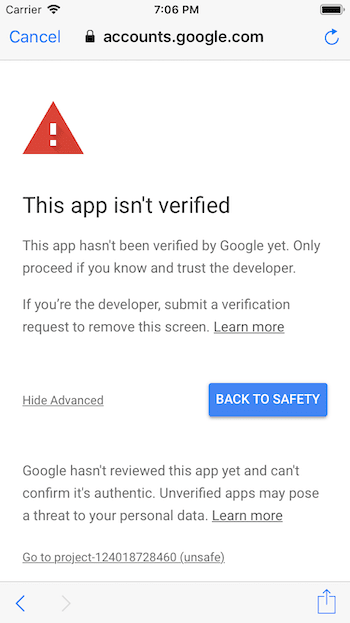
- webClientId: Client ID of type WEB for your server needed to verify user ID and offline access. This is what we copied from Firebase console in Step 2
- offlineAccess: If you want to access Google API on behalf of the user from your server
- hostedDomain: Specifies a hosted domain restriction for auth
- loginHint: Only for iOS — The user’s ID, or email address, to be prefilled in the authentication UI if possible. (See docs here)
- forceConsentPrompt: Only for Android — if you want to show the authorization prompt at each login.
- accountName: Only for Android — Specifies an account name on the device that should be used
-
iosClientId: Only for iOS — Optional, if you want to specify the client ID of type iOS (otherwise, it is taken from
GoogleService-Info.plist
). Of course, if you omit this, yourGoogleService-Info.plist
needs to be correctly configured in XCode.
5.3 Sign In Method
The signIn
method called from the Google Login button goes like this
The userinfo
is saved in the state and hence can work to take user to next page, or change elements in the same page. For the sake of simplicity, I won’t put in another page and trouble you with concepts of routing. Instead, we’ll just change the elements of our homepage when user gets logged in. Sounds fair ? Cool !
5.4 Sign Out Method
The sing out method looks like this
5.5 Silent login
When user logs in once, on next app start you don’t want user to login again. Hence you try to silently login the user. If user is already logged in and has a valid session, the user will silently login. You can call this method as well in componentDidMount
. Remember to call configure
method before this as well. If the user does not have a valid session, this method will return null
and you can ask user to sign in
There are more methods like isSignedIn()
, getCurrentUser()
, getTokens()
etc, whose details you can get on the package’s Github.
Overall, my LoginController.js
file looks like this (a bit long, I know)
Step 6: Test your app on Android device
Let’s now build the app for Android device. I am using a device, but you can always use a simulator as well.
Run the app on the device using
$ react-native run-android
Here’s how my Google Login app works on my device
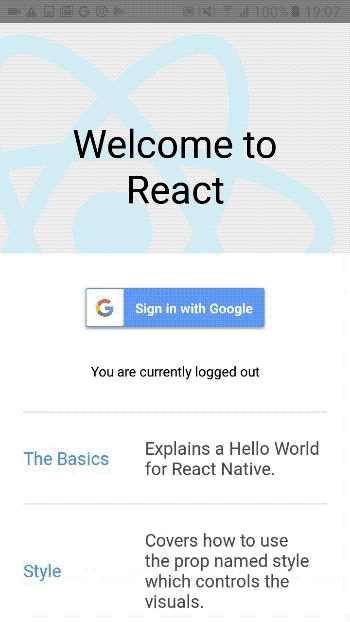
Note : If you have more scopes than just basic info, you’ll see an App Not Verified
screen. You can skip the warning and still login. But that warning won’t look good to your user. You might consider getting your app verified if you get that warning.
Troubleshooting : If you are facing any issues in the above setup, there are few good answers to some common questions here
UserInfo
After login, here’s how my user data looks like in essence
I have arranged this in the LoginController.js
in a list format for better UI.
Step 7: Use Firebase to handle user info / auto-login
We have seen above that using “Silent Login”, we can login the user silently. Also, notice that we haven’t connected Firebase directly to our app till now, and still managed to login using Google. That’s why the Firebase user table is still empty
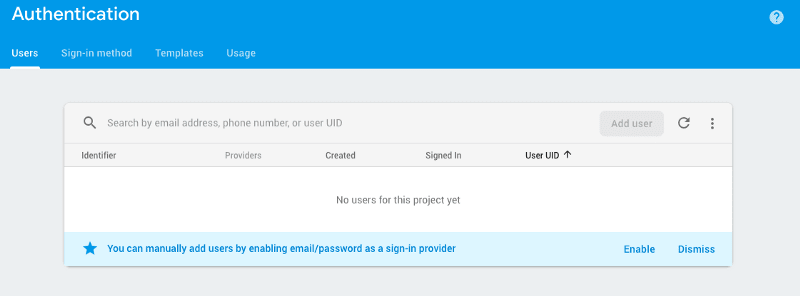
We only used Firebase for
- Creating a Google project
- Obtaining
web_client_id
and addSHA-1
key to the project
Both of which can be done in Google Developer Console as well, without touching Firebase.
So why do we need to attach Firebase at all ?
- Well, we don’t need to. If you have an app, you’re probably going to have a back-end with it as well. Generally we store auth credentials like token and ID in back-end, so the server can authorize access based on valid sessions. In case you are using Firebase as a back-end for your app (which is a great choice btw), you can attach Firebase to Google Login, and store the
token
anduserID
in Firebase. This way, Firebase will take care of your user sessions. - If you have more than one login methods e.g. Facebook login, direct email login, you would like to have the control in single place. Firebase is perfect for such a situation.
- Storing user info in Firebase is useful for analytics and so many other purposes, rather than having the info just in the user’s app.
- And because you can ! 😎
Connect Firebase to your React Native app using react-native-firebase
react-native-firebase
is a popular package to connect React Native apps to Firebase various functionalities.
React-native-firebase also recommends using react-native-google-signin
for Google authentication, which we just used above. The react-native-google-signin
library allows us to login (using GoogleSignin
) which returns an optional accessToken
and idToken
. Once we have the two tokens we need to create a credential using GoogleAuthProvider#credential and then sign in with that credential using auth#signInWithCredential
- Install
react-native-firebase
package using
$ npm install --save react-native-firebase
This installs the package, but it still needs to be connected to your android app properly
Modify Android config files
If you have done the previous steps (in Step 4.4) correctly, then you only need to add these things further
- Add following Firebase modules in dependencies in
android/app/build.gradle
dependencies {
.....
implementation project(':react-native-firebase')
implementation "com.google.firebase:firebase-auth:17.0.0"
implementation "com.google.android.gms:play-services-base:16.1.0"
implementation "com.google.firebase:firebase-core:16.0.9"
....
}
Troubleshooting — If you face issues in above settings, visit the documentation of react-native-firebase
to get detailed steps for your RN versions
Link the react-native-firebase package with Android platform
Link the react-native-firebase package with Android platform using
$ react-native link react-native-firebase
However, from RN ≥0.60, you don’t need to manually link packages. It is done automatically when packaging the platform for build.
Changes in LoginController.js
Now that we are going to save the login info in Firebase, we’ll call a Fierbase method to store the required data in back-end. Following function logs the user in , and then saves the info in Firebase
Note, firebase.auth().signInWithCredential
is the method used to store user credentials in back-end.
Now, run the app again using CLI or Android Studio, log in as usual and you’ll see that a user pops up in Firebase Users list
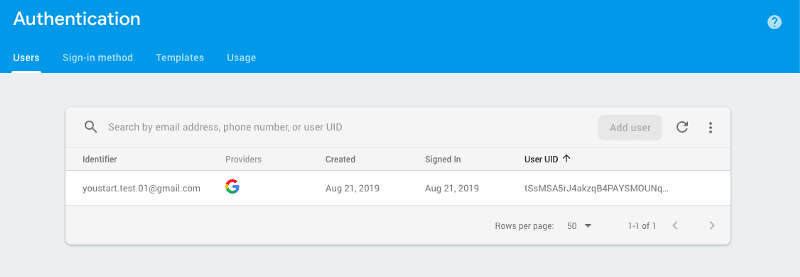
You have successfully
- Logged in a user using Google Login in React Native
- Attached the authentication to Firebase to store user credentials
🎉 🎉 🎉 🎉 🎉 🎉 🎉
Firebase, further
You can now go ahead and attach more Firebase functions like logout, silent login, userinfo etc. since you have user credentials in Firebase.
Note, not all userInfo that we receive after Google Login gets stored in Firebase by default. If you want all the information to be stored, then you should use the FireStore database to store it, and retrieve it when required.
For more details on functions like
- CRUD operations in Firestore
- Image Upload
- Phone authentication
check out this blog.
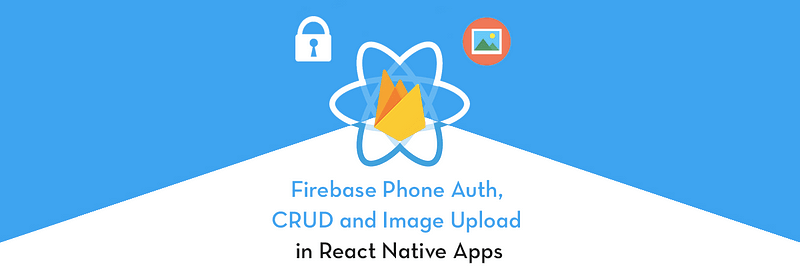
Conclusion
In this blog, you learnt how to implement Google Login in React Native apps for Android. You also learnt how to attach this authentication to Firebase for better handling using back-end. We also built the app in Android and ran on a device.
Complete source code of this tutorial is available here — React-Native-google-login (android branch)
Next Steps
Now that you have learnt about setting up Google login in React Native apps, here are some other topics you can look into
- Firebase — Integrate Firebase | Analytics | Push notifications | Firebase CRUD
- How To in React Native — Geolocation | Life cycle hooks | Image Picker | Redux implementation | Make API calls | Navigation | Translation | Barcode & QR code scan | Send & Read SMS | Google Vision
- Payments — Apple Pay | Stripe payments
- Authentication — Google Login| Facebook login | Phone Auth | Twitter login | Anonymous Login
- Create Instagram / Whatsapp Story Feature in React Native
- React Native life cycle hooks | Implement Redux | Async actions with Redux
- Create Awesome Apps in React Native using Full App
If you need a base to start your next React Native app, you can make your next awesome app using React Native Full App

Top comments (0)