If you are building a project that involves customer interactions, then probably at some point you would have to send them an email as well.
For example, on successful form submits, you need to send a confirmation email. Or on every purchase, a receipt or order details.
You could hook up some of the existing apis like send in blue, mail chimp etc, but you can do it for free in nodejs itself.
Node Mailer is a nodejs module, that makes it easy to send emails.
Here's how you do it;
-> First, install Node Mailer
npm install nodemailer
-> Then require('nodemailer')
-> Create a transporter
Transporter is the object that is able to send the email. It contains data about the connection.
I'm using gmail to send emails and this is how the transporter looks for me:
const transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: myemail@gmail.com,
pass: password
}
});
-> We also need an object containing the message to be sent
const mailOptions = {
from: 'The Idea project',
to: toAddress,
subject: 'My first Email!!!',
text: "This is my first email. I am so excited!"
};
You can send html emails with html key instead of text.
-> Next, to actually send the email, use
transporter.sendMail(mailOptions, callback)
The call back takes error and info arguments and is executed once the sending process is complete. You can use this to log errors if any.
You can customise the emails you send, where you send from and how you send it anyway you want. Read the docs here.
The complete code should look like this,
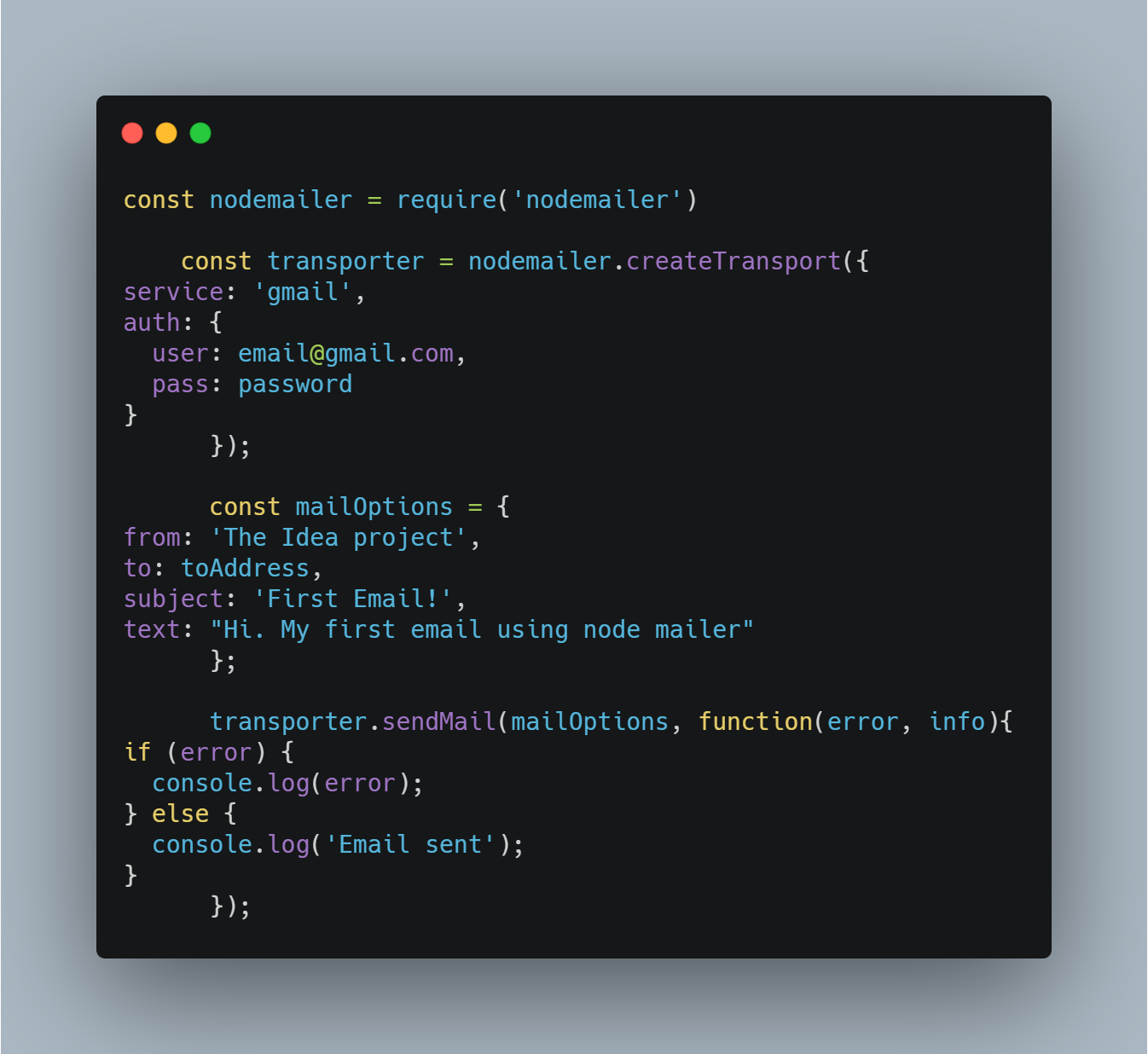
Top comments (12)
I think this article should come with a disclaimer. :)
The reason people might wanna use MailChimp or other services is that their IP's are not blacklisted, known to email-providers, properly encrypted etc.
Without all of that, there's a really good chance the email's will end up blackholed or in the spam folder.
Not bashing on the article here, but just so everyone understands that this should not be used in a production environment with customers if you want them to get your emails.
For personal projects & prototypes then this approach is fine. :)
Thanks Sebastian. Is there a way to avoid my servers being black listed?
It's not something I have delved into personally. :)
I would probably look the other way around, and look to get whitelisted with the various larger email providers somehow.
It's also possible they have a common third-party one can get whitelisted with such as spamhaus.org/
There's bound to be some articles on how to not get spam'canned out there that would be worth looking up. :)
Yup. Makes sense not reinventing the wheel!
Can this be done with vanilla JS? Like in React?
Hey! To solve your problem you can create a nodejs server and create an endpoint that sends the email like in this post. In your React app you can use axios to hit the endpoint and thus sending the email. Just send the message in the parameters so it can be dynamic.
Yeah got it. Thanks for answering!😄
I guess no. you need a server to send email via nodemailer
You can't.
What you can do is to use cloud functions such as firebase functions, aws lambda or azure functions.
No it cant be react is a frontend library not a backend library but you can use expressjs to setup a API and acess it from react
You could use mailer.reaper.im
I'm trying to implement a welcome mailer function but I'm stock for 2 hours right now.