I overshot the goal on a homework assignment and came up with a function that would handle edge cases. It was just supposed to take in three distinct numerical inputs and determine which one was the largest. But I built my function to account for the possibility that two or even all three of the numbers might be identical, plus the possibility that one or all of the inputs might be non-numerical. It may have been overkill, but I’m pretty proud of how much I nerded out here.
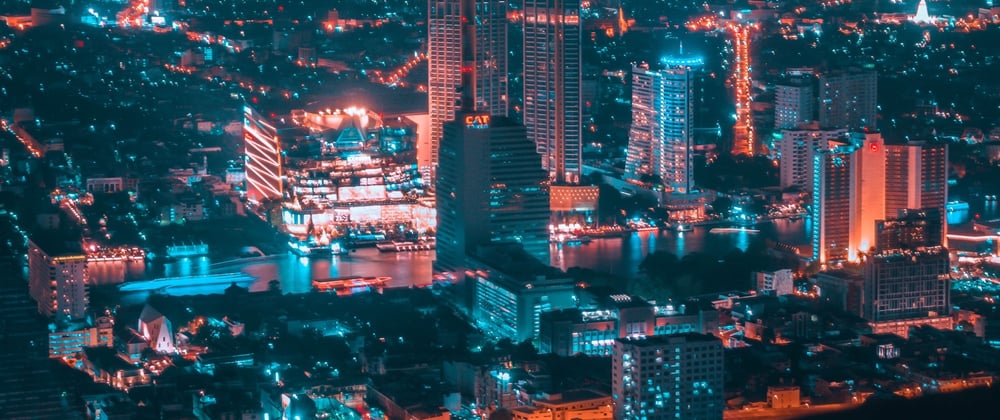
For further actions, you may consider blocking this person and/or reporting abuse
Top comments (0)