import java.util.EmptyStackException;
public class StackDynamic<T> {
private class StackNode {
private T data;
private StackNode next;
private StackNode(T data) {
this.data = data;
this.next = null;
}
}
private StackNode top;
public StackDynamic() {
this.top = null;
}
public StackDynamic(T data) {
this.top = new StackNode(data);
}
public T pop() {
if (this.top == null) throw new EmptyStackException();
T data = this.top.data;
this.top = this.top.next;
return data;
}
public void push(T data) {
StackNode temp = new StackNode(data);
temp.next = this.top;
this.top = temp;
}
public T peek() {
if (this.top == null) throw new EmptyStackException();
return this.top.data;
}
public boolean isEmpty() {
return this.top == null;
}
}
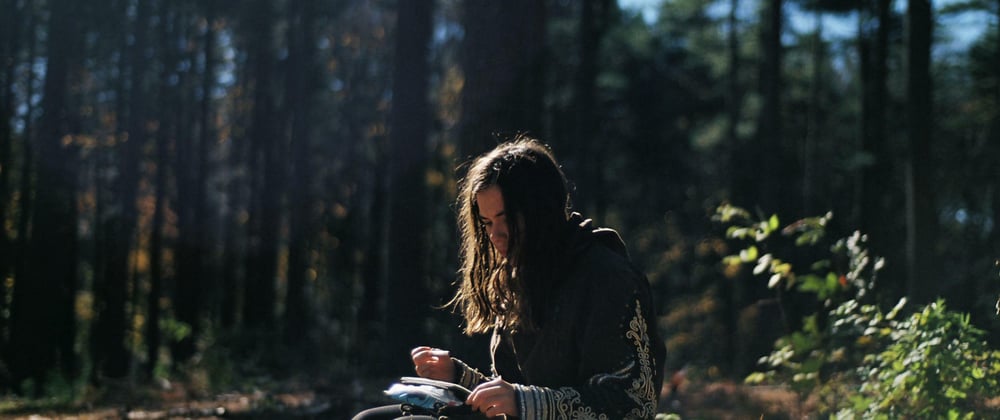
For further actions, you may consider blocking this person and/or reporting abuse
Top comments (0)