Hello, my dear followers!
If this is the first time you've visited my page, a warm welcome to you. My name is Jamshid, and I'm thrilled to share a topic that's not only fascinating but also incredibly important in our digital world: the Advanced Encryption Standard, commonly known as AES.
This encryption algorithm has been a personal favorite of mine for several years. Its robustness and versatility have made it a go-to choice in various applications, from securing personal data to protecting global communications.
In this post, I'll be diving deep into AES. We'll explore how it works, why it's used so extensively, and discuss its various use cases. I'll also shed light on its advantages and disadvantages, giving you a comprehensive understanding of why AES stands out in the world of encryption.
Whether you're a tech enthusiast, a privacy advocate, or just curious about the digital security landscape, this series will provide valuable insights into one of the most critical aspects of data protection.
AES (Advanced Encryption Standard) is a widely used symmetric encryption algorithm that provides strong security. It's used in a variety of applications, both in personal and enterprise environments. Here's an overview of its general use cases and its role in server development.
General Use Cases of AES:
Data Encryption: AES is commonly used to encrypt sensitive data, such as personal information, financial records, and confidential documents.
Secure Communications: It's used in protocols like SSL/TLS for securing internet traffic, ensuring that data transferred between servers and clients remains private.
File Encryption: AES can encrypt files and entire filesystems, protecting data at rest from unauthorized access.
VPN (Virtual Private Networks): AES is often the encryption standard of choice for VPNs, securing data transmitted over public networks.
Wireless Security: It's used in WPA2 (Wi-Fi Protected Access 2) for securing wireless networks.
Database Security: Databases can leverage AES to encrypt sensitive data stored within.
AES in Server Development:
Data Protection: In server development, AES is essential for protecting data both in transit and at rest. Servers often handle sensitive data that requires encryption to meet privacy standards and regulatory requirements.
Secure Communication: For any server that communicates over the internet, using AES within SSL/TLS protocols ensures that the data exchanged with clients is secure.
API Security: When developing APIs, especially for financial or personal data transactions, AES can be used to encrypt the payload.
Is It Always Good to Use AES in Server Development?
Yes and No. While AES is a robust encryption standard, whether it should be used in a specific scenario depends on the use case, performance requirements, and compliance needs. In most cases, using AES adds a strong layer of security, but there are considerations to be mindful of.
Pros and Cons of Using AES in Server Development:
Pros:
- Strong Security: AES is considered secure against most cryptographic attacks.
- Industry Standard: Widely accepted and used, meeting various compliance requirements.
- Versatile: Suitable for a wide range of applications, from encrypting data in transit to securing stored files.
- Performance: Generally offers good performance, especially with hardware support.
Cons:
- Key Management: Secure key management is crucial. Poorly managed keys can negate the benefits of encryption.
- Overhead: Encryption and decryption add computational overhead, which might impact performance in resource-constrained environments.
- Complexity: Implementing encryption correctly requires expertise; incorrect implementation can lead to vulnerabilities.
- Encryption Only: AES provides confidentiality but not authentication or integrity. Additional mechanisms (like HMAC or using AES in GCM mode) are needed for these.
Type Of AES
AES (Advanced Encryption Standard) is a symmetric encryption algorithm that is widely used across the globe. It has several modes of operation, each designed to serve a specific purpose and to meet different security requirements. Below is a list of some common AES modes, along with their key differences:
-
ECB (Electronic Codebook)
- Description: Simplest mode, where each block of plaintext is encrypted independently.
- Key Differences: Vulnerable to pattern attacks since identical plaintext blocks result in identical ciphertext blocks. Not recommended for encrypting multiple blocks of data that may contain repetitions.
-
CBC (Cipher Block Chaining)
- Description: Each block of plaintext is XORed with the previous ciphertext block before encryption. Requires an Initialization Vector (IV) for the first block.
- Key Differences: More secure than ECB as it introduces dependencies between blocks. However, it's susceptible to block reordering attacks and needs padding for the last block if the data isn't a multiple of the block size.
-
CFB (Cipher Feedback)
- Description: Converts AES into a stream cipher. The previous ciphertext block is encrypted and then XORed with the plaintext to produce the next block of ciphertext.
- Key Differences: Suitable for encrypting data streams of arbitrary length. However, like CBC, it requires an IV and is sensitive to bit errors in transmission.
-
OFB (Output Feedback)
- Description: Similar to CFB, but encrypts the previous output instead of the previous ciphertext. Also, turns AES into a stream cipher.
- Key Differences: Resilient to transmission errors (a bit error in ciphertext only affects the corresponding bit in plaintext). It can be used in applications where error propagation is a concern.
-
CTR (Counter)
- Description: Uses a counter value that is encrypted and then XORed with the plaintext to produce the ciphertext. Each block uses a different counter value.
- Key Differences: Highly parallelizable and can pre-compute encrypted counters. Suitable for high-speed requirements and is resilient to bit errors in transmission.
-
GCM (Galois/Counter Mode)
- Description: A mode based on CTR mode for encryption, but also provides data integrity/authentication using a technique called GMAC (Galois Message Authentication Code).
- Key Differences: Offers both confidentiality and integrity. It's widely used in network protocols like TLS due to its efficiency and security.
-
CCM (Counter with CBC-MAC)
- Description: Combines CTR mode for encryption with CBC-MAC for authentication.
- Key Differences: Provides both encryption and authentication but has stricter requirements on the size of the input data and the nonce.
Known attacks:
Read more in wiki: Side-channel attacks
Let's take a look at AES encrption and dectyption with a simple example
Setting Up the Environment
Before diving into the code, make sure you have Node.js installed. You can download it from Node.js official website.
- Initialize a new Node.js project:
mkdir aes-nodejs-typescript
cd aes-nodejs-typescript
npm init -y
- Install TypeScript and necessary types:
npm install typescript @types/node --save-dev
- Create a
tsconfig.json
file for TypeScript configuration:
npx tsc --init
Modify the tsconfig.json
as needed for your project.
- Install crypto module:
Node.js has a built-in module called crypto
which we will use for AES encryption. So no need separate installation.
npm install crypto // install if your node can`t see crypto module
```
#### Implementing AES in TypeScript
Now, let's implement AES encryption and decryption in TypeScript.
1. **Create a file `aes.ts`:**
```typescript
import { createCipheriv, createDecipheriv, randomBytes, scryptSync } from 'crypto';
const algorithm = 'aes-256-cbc';
// Generate a secure, random key
const key = randomBytes(32);
// Generate an initialization vector
const iv = randomBytes(16);
export function encrypt(text: string): string {
const cipher = createCipheriv(algorithm, key, iv);
let encrypted = cipher.update(text, 'utf8', 'hex');
encrypted += cipher.final('hex');
return encrypted;
}
export function decrypt(encryptedText: string): string {
const decipher = createDecipheriv(algorithm, key, iv);
let decrypted = decipher.update(encryptedText, 'hex', 'utf8');
decrypted += decipher.final('utf8');
return decrypted;
}
```
2. **Using the AES functions:**
Create a new file `index.ts` and use the `encrypt` and `decrypt` functions.
```typescript
import { encrypt, decrypt } from './aes';
const originalText = 'Hello World!';
const encryptedText = encrypt(originalText);
const decryptedText = decrypt(encryptedText);
console.log(`Original Text: ${originalText}`);
console.log(`Encrypted Text: ${encryptedText}`);
console.log(`Decrypted Text: ${decryptedText}`);
```
3. **Compile and Run:**
Compile the TypeScript code to JavaScript and then run the program.
```bash
npx tsc
node dist/index.js
```
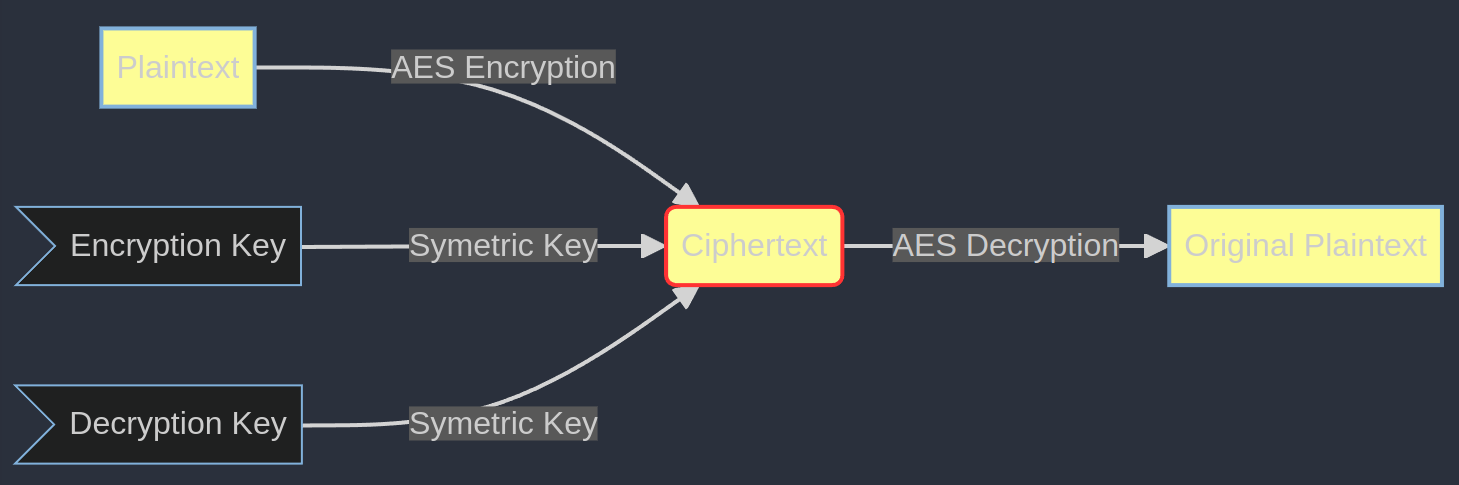
4.**Explanation:**
1. The flow starts with the Plaintext, goes through AES Encryption to become Ciphertext, and then goes through AES Decryption to return to the Original Plaintext.
2. The Encryption Key and Decryption Key are shown to be used in the encryption and decryption processes. (In AES symmetric encryption, these keys are typically the same.)
## 🔐 Encryption Algorithms & Vulnerabilities 🔐
Did you know that even some of the most renowned encryption algorithms, including AES, aren't entirely immune to threats? 🤔 In today's rapidly evolving digital landscape, nothing is truly hack-proof.
> 💡 Curious to unravel the mysteries behind these vulnerabilities? If this post gets a whopping 1K likes, I'll delve deep into this topic in my upcoming articles.
🚀 To kickstart this exciting series, I'm setting a goal: 1,000 likes. Yes, you heard it right! Once we hit that magic number, I'll start unraveling the mysteries of encryption vulnerabilities.
❤️👍 Hit that like button!
💬 Drop your thoughts in the comments below.
🚀 Share this post far and wide with your tech-savvy friends and encryption enthusiasts.
Let's embark on this cryptographic journey together and explore the unknown realms of encryption! 🌍✨
Stay curious and connected! 🌟
Top comments (0)