This blog post is providing some more insight and the source code to a youtube tutorial, that I consider being worthwile looking at more closely.
Original Youtube video that inspired me:
Github code repo: https://github.com/zoltanhalasz/CoreRDLCReport.git
Live sample application: https://rdlc-aspnetcore.zoltanhalasz.net/
Prerequisites:
- Asp.Net Core MVC basics
- Basic RDLC knowledge
Steps To follow:
- Create an Asp.Net Core 3.1 MVC project
- Add following Nuget Packages: "AspNetCore.Reporting", "System.CodeDom", "System.Data.SqlClient"
- Modify the Program.cs Class, by making sure it has the below function as: ```csharp
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseContentRoot(Directory.GetCurrentDirectory());
webBuilder.UseWebRoot("wwwroot");
webBuilder.UseStartup();
});
* Add a new project to the existing solution, a WinForm application. Create an empty RDLC report there, and also a DataSet for the report.
If your Visual Studio does not include the extension for RDLC reports, please take a look
* Move the empty report to your MVC project, into wwwroot/Reports folder, and the dataset to a ReportDataset folder in the MVC project.
* Configure the dataset and then the RDLC report:
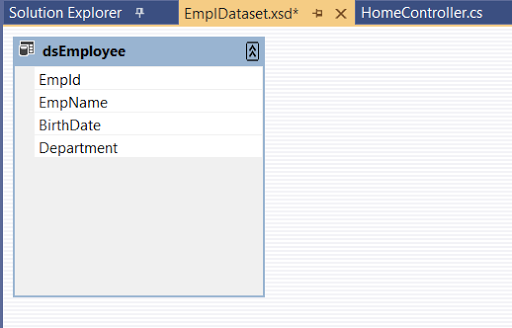
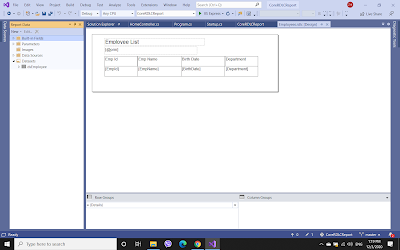
The above report will have a string (text parameter) "prm" in it.
* Create the HomeController in the following way:
```csharp
public class HomeController : Controller
{
private readonly ILogger<HomeController> _logger;
private readonly IWebHostEnvironment _webHostEnvironment;
public HomeController(ILogger<HomeController> logger, IWebHostEnvironment webHostEnvironment)
{
_logger = logger;
_webHostEnvironment = webHostEnvironment;
System.Text.Encoding.RegisterProvider(System.Text.CodePagesEncodingProvider.Instance);
}
public IActionResult Index()
{
return View();
}
public IActionResult Print()
{
var dt = new DataTable();
dt = GetEmployeeList();
string mimetype = "";
int extension = 1;
var path = $"{this._webHostEnvironment.WebRootPath}\\Reports\\Employees.rdlc";
Dictionary<string, string> parameters = new Dictionary<string, string>();
parameters.Add("prm", "RDLC report (Set as parameter)");
LocalReport lr = new LocalReport(path);
lr.AddDataSource("dsEmployee", dt);
var result = lr.Execute(RenderType.Pdf, extension, parameters, mimetype);
return File(result.MainStream,"application/pdf");
}
public IActionResult Export()
{
var dt = new DataTable();
dt = GetEmployeeList();
string mimetype = "";
int extension = 1;
var path = $"{this._webHostEnvironment.WebRootPath}\\Reports\\Employees.rdlc";
Dictionary<string, string> parameters = new Dictionary<string, string>();
parameters.Add("prm", "RDLC report (Set as parameter)");
LocalReport lr = new LocalReport(path);
lr.AddDataSource("dsEmployee", dt);
var result = lr.Execute(RenderType.Excel, extension, parameters, mimetype);
return File(result.MainStream, "application/msexcel", "Export.xls");
}
private DataTable GetEmployeeList()
{
var dt = new DataTable();
dt.Columns.Add("EmpId");
dt.Columns.Add("EmpName");
dt.Columns.Add("Department");
dt.Columns.Add("BirthDate");
DataRow row;
for (int i = 1; i< 100; i++)
{
row = dt.NewRow();
row["EmpId"] = i;
row["EmpName"] = i.ToString() + " Empl";
row["Department"] = "XXYY";
row["BirthDate"] = DateTime.Now.AddDays(-10000);
dt.Rows.Add(row);
}
return dt;
}
}
- Then, add the view for the Home controller (Index.cshtml) ```html
<h1>RDLC Print</h1>
<a href="../home/print">Print PDF</a>
<a href="../home/export">Export Excel</a>
This will generate PDF and export excel, based on the dummy data we populated the report.
* The result will be:
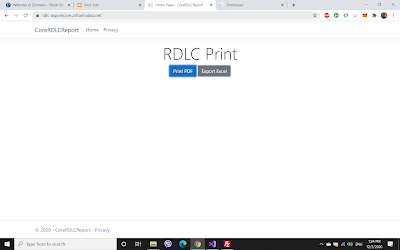
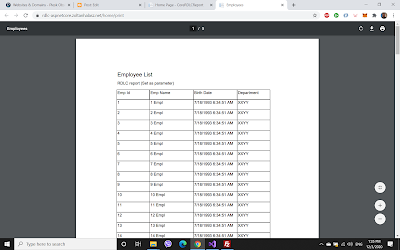
Top comments (13)
I have a Problem with 2 different report types in one application.
It works only with the report type that I call first.
For the second I got the following exception:
NullReferenceException: Object reference not set to an instance of an object.
AspNetCore.ReportingServices.ReportIntermediateFormat.Paragraph.SetExprHost(TextBoxExprHost textBoxExprHost, ObjectModelImpl reportObjectModel)
ReportProcessingException: An unexpected error occurred in Report Processing.
Object reference not set to an instance of an object.
AspNetCore.ReportingServices.ReportProcessing.Execution.RenderReport.Execute(IRenderingExtension newRenderer)
I would really appreciate any idea.
Thanks
Could you manage to solve this? I Have the same problem, I lost months in solving it. THank you
I moved to github.com/lkosson/reportviewercore.
It works fine.
Thanks for answering. If you have time to help, please send some call of .rdlc report using this "reportviewercore" package.. I am struggling with it. THanks!
We are trying to use microsoft graphs in the rdlc report and getting error below
"Could not load file or assembly 'System.Windows.Forms. version=4.0.0.0. Culture=neutral, PublicKeyToken=b77a5c561934e089i. The system cannot find the file specified. "
Did anyone ran in this issue?
Thanks in Advance.
Whast this Error => AspNetCore.Reporting.LocalProcessingException: 'An error occurred during local report processing.;An unexpected error occurred in Report Processing.
Index was outside the bounds of the array.'
i have the same issue, well i have areound 7 rdlc files, if i run the application and execute any report that work fine, but when i try to execute another report then throw the following error: AspNetCore.Reporting.LocalProcessingException: An error occurred during local report processing.;An unexpected error occurred in Report Processing.
I have lost several days on this, I would appreciate if you help me with this
So far this worked great for me. The only issue I ran into is I have External Images for one of my reports. I keep getting EnableExternalImages property has not been set for this report. I'm not sure if this is possible in this example.
Can this solution be deployed on Linux?
I didn't try it. I heard some doubts. You can try it though.
Can't This Project Work On .Net 6.0 Framework?
sometimes it throws an exception
github.com/amh1979/AspNetCore.Repo...
Hey great job, thanks. Have you seen an issue with external images?