You can find the complete code in the Ionic 4 Phaser Starter
Ionic platform has become one of the big names in app development in recent years. Moving from Ionic 1 to 4 in past few years, Ionic has not just gained popularity, but also improved its robustness and reliability. Today, Ionic framework is one of the easiest to make an android or iOS app with, and also Progressive web apps. 🚀
Phaser, first released in 2013, has now become a huge name in 2D HTML5 games. Based on javascript, it can easily be integrated in a variety of javascript frameworks. 👾 👾 👾
So why integrate Phaser and Ionic ? 🚀 👾
While Ionic gives you the power of creating apps and PWA at a lightening speed, you cannot really make games with it. Phaser, while great at game building, is not really about building a structured mobile or web-app. Hence, building a neat looking game app or PWA with Phaser requires an HTML + CSS wrapper, a void which Ionic perfectly fills.
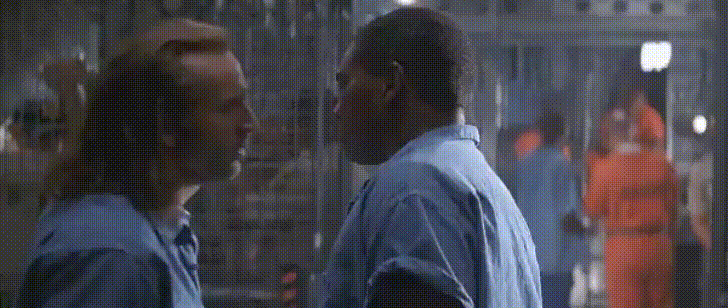
Step 1: Create an Ionic 4 app
First make sure you have latest Ionic installed
npm install -g ionic
With Ionic CLI installed, create a simple Ionic app
ionic start ioniser sidemenu
I have picked sidemenu
as a layout choice, but you can use blank
or tabs
as well. This command will generate a basic Ionic app with a sidemenu
and some basic HTML in homepage. Serve your app using
ionic serve
and check everything runs fine. For me it looks like following
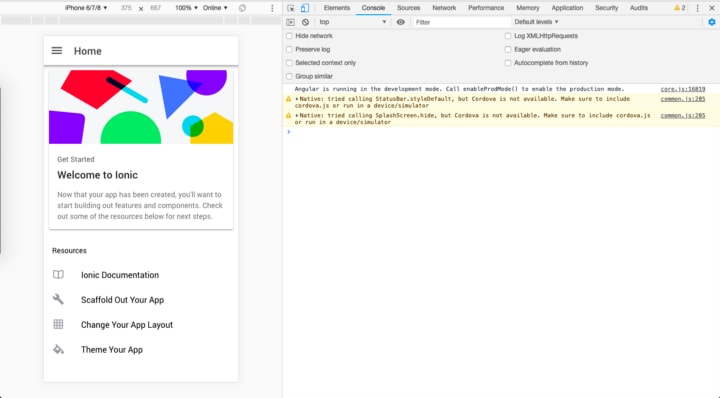
Step 2: Include Phaser in the project
Phaser is super-easy to include in the project. Essentially it is just a Javascript file that you need to include in your HTML5 project. There are two ways to do it
#1 — The cool “just-get-it-done” way (faster)
Download phaser.min.js
from Phaser official downloads and link it with your project. For demo purpose, I will keep this file in assets
folder, but you can link it from CDN as well.
Include the phaser.js file in index.html
files as follows
<script src="assets/phaser.min.js"></script>
Now you can include phaser in your component .ts
files using
declare var Phaser;
(To check what Phaser
contains, try consoling the variable or its defaultState
saved in this
and you’ll get some insights into Phaser’s built.)
#2 — The complex “I-am-a-coding-nerd” way
Download the npm package from phaser with
npm install phaser-ce
Prepare webpack to compile the phaser package so you can import
the package in the .ts file. You might have to prepare your own webpack-config for this and install additional modules. After this, you can import the variables as
import "pixi";
import "p2";
import * as Phaser from "phaser-ce";
Details are given here (I am yet to try this method at the time of writing this blog)
Step 3: Implement Phaser game
You can find the complete code in the Ionic 4 Phaser Starter
For sample purpose, we’ll use a simple Phaser example where we will make a Zombie walk.
No, no, not that Zombie. 😆
We will focus on home
component that comes with the starter. So all the relevant javascript code will go in home.page.ts
file. Replace the whole content in home.page.html
file with the following
<ion-content>
<div id="phaser-example"></div>
</ion-content>
We will identify the phaser-example
in our javascript logic and instantiate the game in this div.
A basic Phaser game has three default functions — preload(), create() and update()
> Preload — As the name suggests, preloads assets etc. In our case, this function will load the zombie and background images.
preload() {
this.game.load.image('lazur', 'assets/thorn_lazur.png');
this.game.load.spritesheet('mummy', 'assets/metalslug_mummy37x45.png', 37, 45, 18);
}
> Create — Creates the game instance and loads the game state. In our game, this function will set the images in the scene. We will also create and set animation listeners here.
create() {
// Set background
this.back = this.game.add.image(0, -400, 'lazur');
this.back.scale.set(2);
this.back.smoothed = false;
// Set mummy from a sprite
this.mummy = this.game.add.sprite(200, 360, 'mummy', 5);
this.mummy.scale.set(4);
this.mummy.smoothed = false;
// Set mummy animation
this.anim = this.mummy.animations.add('walk');
this.anim.onStart.add(that.animationStarted, this);
this.anim.onLoop.add(that.animationLooped, this);
this.anim.onComplete.add(that.animationStopped, this);
this.anim.play(10, true);
}
> Update — Update the game state on looping, actions, physics interactions etc. For our game, this will update the animation by moving the background to create the effect of a “walk”
update() {if (this.anim.isPlaying) { this.back.x -= 1;}}
and Tada !!! 🎉 🎉🎉 We have our game running in the serve window

In the same way, you can run any Phaser game with Ionic. Trust me, there are some incredible looking games made in Phaser. We made a Mario replica some time back, which I will share in another blogpost.
⭐️️️️⭐️️️️⭐️️️️ Important️️ ⭐️️️️⭐️️️️⭐️️️️
The Phaser implementation can leave you confused with the implementation of this
. In short, once the Phaser scene is initialized, this
contains the default game state. As an Ionic developer, or if you follow Ionic documentation, you will user this
to refer to the component’s class. So you end up using conflicting this
. For a quick work-around, I assign the Ionicthis
to another variable that
(😅) and then use it to point to class functions.
A common mistake is to declare that = this
. Yes, it does reference this
via that
, but as soon as this
changes, that
also changes, and hence there is no point of doing this. You might do that = Object.assign({},this)
which is a good standard code. But you will find that this also does not result in what we want. Because in Ionic this
contains the class functions inside _prototype
, to copy those functions into that
you should define it like
that = Object.create(this.constructor.prototype);
And then use that
in place of this
to call functions
E.g. that.animationStarted
points to a function animationStarted
. Using this
here would throw an error because create()
function is a Phaser default and contains scene’s default state inside this
If you are not comfortable with the this
concept, you should read more about it here.
(As another work-around, you can put the complete javascript code outside the class implementation of Ionic, or worse even, in a <script></script>
tag in index.html
, but that is too much of a hack. You won’t be able to write huge game codes this way)
Step 4: Build the game into a Progressive Web App
It is impressive to have the game running in your ionic serve
window, but it would be a lot cooler if you can release this on your own domain. Ionic has your back.
Prepare the app for a PWA deployment by running following commands
ng add @angular/pwa
The @angular/pwa
package will automatically add a service worker and a app manifest to the app. Then run
ionic build --prod
to create a release build of the app. Now your app contains an index.html
file which can be served over any server. The quickest way is to deploy on a firebase hosting by installing firebase-tools and deploying the game on your firebase project using firebase deploy
(details here)
🎉🎉🎉 Voila !! You own game is released on your website now🎉🎉🎉
Step 5 : Build the game into a mobile app
Building an Ionic app with Phaser is exactly same as building a regular Ionic app. For those who are not familiar with the process in Ionic 4, first add the platform you want to run the app on
ionic cordova platform add android
Then run the app on a connected device or an emulator by running
ionic cordova run android
You might want to adjust the canvas
size as per the device size using window.innerWidth
and window.innerHeight
. With this, you can adjust the game canvas for all mobile devices sizes.
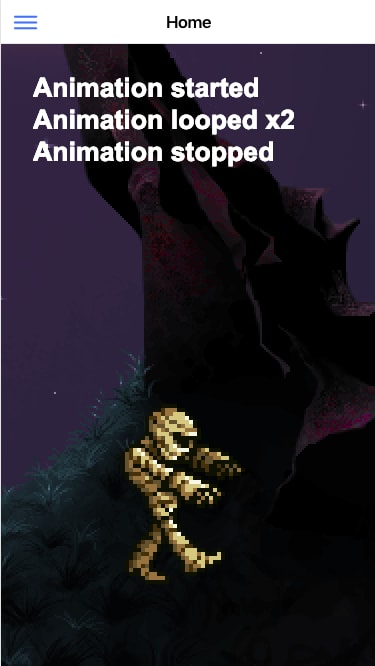
With these tweaks, the game looks like above. Not bad, huh. 👻
Cool thing is, you can still have the sidemenu, or tabs like a mobile app and put in all regular functionalities of the app in the game (or vice versa) like login, signup etc. making use of Ionic plugins.
Thinking of making your own PUBG or space invader game in Ionic ? Try making this one
Conclusion
Ionic is super fast in making mobile apps, while Phaser is great for making 2D HTML5 games. Follow this blog to combine these two to make a quick mobile game app or game PWA. Make the game feature rich by using Ionic’s powerful plugins. Have fun !
We do more such fun stuff at Enappd on a regular basis. Feel free to browse through our interesting app starters.
Update
Space-Invaders game now included in space-invaders
branch on the same repo. This is how it looks like
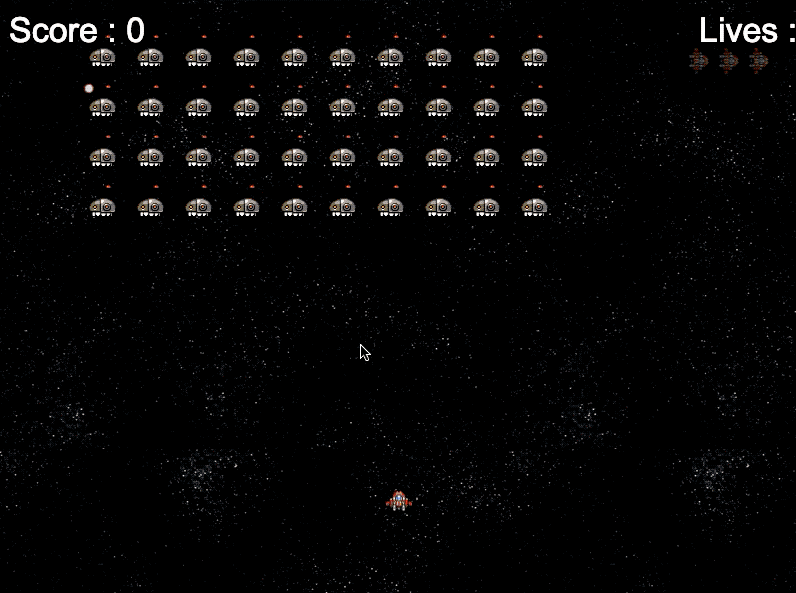
FOUND THIS POST INTERESTING ?
Also check out our other blog posts related to Firebase in Ionic 4, Geolocation in Ionic 4, QR Code and scanners in Ionic 4 and Payment gateways in Ionic 4
NEED FREE IONIC 4 STARTERS?
You can also find free Ionic 4 starters on our website enappd.com
You can also make your next awesome app using Ionic 4 Full App
Top comments (1)
Thanks for sharing! Try using Phaser 3 with this WebComponent and let me know what you think :) github.com/proyecto26/ion-phaser