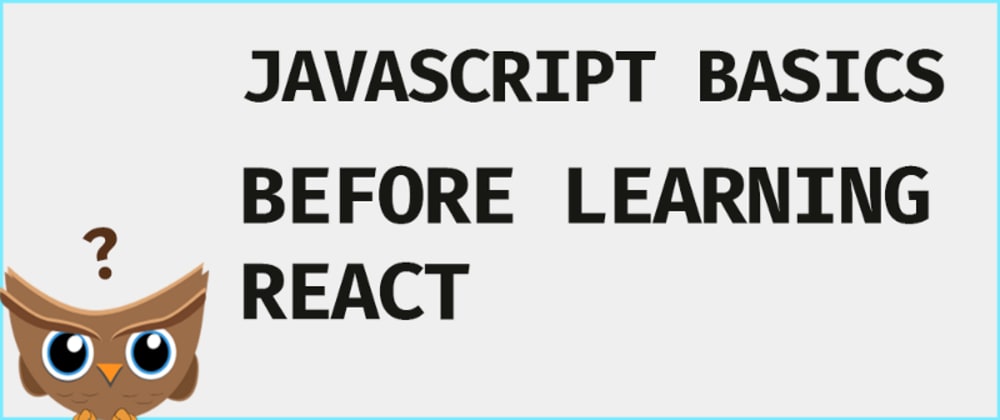
In an ideal world, you can learn all about JavaScript and web development before you dive into React.
Unfortunately, we live in a not-perfect wor...
For further actions, you may consider blocking this person and/or reporting abuse
Arrow functions, apart from their aesthetics, have this property called
lexical scoping
. This explains lexical scoping better than I ever will :)In short, arrow functions follow the scope of the caller's scope, rather than having its own. function() {} functions' scope can change to whatever by calling .bind() - basically how JS prototype works.
Maybe you deliberately omitted this because in React world arrow functions are mostly used for shorter declaration. But hey, I figured the post might give a wrong impression that arrow function is only for cleaner code :P
Short test code:
`
Ah yes, sorry if I skip the part about this. Thanks for adding it here, Jesse :)
Incredibly helpful for someone who's just getting into React and only knows the basics of JavaScript like me. I'm definitely going to keep practicing JS before actually building something with React, and all of these concepts help a lot
Thank you!
'Both declarations are local, meaning if you declare let inside a function scope, you can't call it outside of the function.'
a var declared inside a function is also not global. this is not what is different about let. the difference is that let has block scope for example:
let x = 1;
if (x === 1) {
let x = 2;
console.log(x);
// expected output: 2
}
console.log(x);
// expected output: 1
Oh sorry, what I actually meant is block scope not function scope. If you call x outside of the if scope it will return 2 with var. I'll fix that as soon possible. Thanks David :)
yap...its super important to Understand JavaScript Fundamental before using any frameworks. One should learn how JavaScript works, its life cycle, What Prototypal inheritance is, Classical vs Prototypal model, What Closure is etc...
Great post Nathan. Easy to understand. Only thing i couldn't get my head around is the Destructuring concept coz i haven't used or heard it before. Hopefully i'll get better understanding after some use.
Thanks.
Thanks dan, don't worry too much about destructuring, in simple application, we used it for shortening the syntax for getting state value only. For example, if you have this state initialized
Then when you want to get the value, do:
Instead of:
Now in my example tutorial, I have also included destructuring assignment into a new variable, like:
But to tell you the truth, I never used this kind of assignment, so just consider it an extra knowledge that might be useful sometime 😆
My friend, hello. You have an error in the section "class inheritance", Because when using extend in the ReactDeveloper class, you should use the constructor and super to pass the "Nath" object as an argument and return the Hello and installReact function.
A exceptión de eso, muy bueno tú post. Muchas gracias.
Hi Nathan!
Great article. Helps to be focused on the fundamentals for learning React. I'm wondering if it's not necessary any previous knowledge of asynchronous JS, like promises or async/await.
Thanks
Excellent Blog! I would Thanks for sharing this wonderful content.its very useful to us.I gained many unknown information, the way you have clearly explained is really fantastic.keep posting such useful information.
MongoDB Interview Question & Answers
Node js Interview Question & Answers
flutter Interview Question & Answers
Html Interview Question & Answers
CSS Interview Question & Answers
JAVASCRIPT Interview Question & Answers
REACT JS Interview Question & Answers
ANGULAR JS Interview Question & Answers
Redux Interview Question & Answers
VUE Js Interview Question & Answers
Thank you for this post! I am actually working on a new web project, and this time I decided to use React instead of JS vanilla. It helped me a lot :D
You're welcome Rospars. Glad could help you out. Good luck with your project :)
Could I translate your post into Korean? I'm working as a front-end developer but mainly maintaining the old legacy. Next version would use React. So, I'm studying React.
Sure, go ahead
Hi, Sebhastian. I've translated your article in Korean and posted on my Medium story. It became the most famous article among my stories. Thanks :)
medium.com/@violetboralee/react%EB...
Hi Lee, wow that's great! Your welcome and thanks for translating the article :)
Thanks :)
Great selection of features. Great article! I would add the spread operator as well. It's useful for making shallow copies of arrays and objects.
I've used
{...this.props}
to pass a copy of props in react without naming them all, and then using destructuring to extract them in the component. See this stackoverflow question for a good description.Ah certainly Steve, spread and rest operator would be a great addition. I'm just afraid the article would be too long when I wrote this. Thanks for your comment :)
@nathan Thanks for articles.
Can you put some light on below code
Good article for the beginner who want to learn reactJS.
Thanks Nathan.
@nathan Thanks for articles.
Can you put some light on below code
Thanks for donating your free time buddy.
Hey, great article, I knew most of this, but the part of modules have clarified me all I didn't have end up understanding.
BTW, I think there is an error here, no?
// in util.js
export default function times(x) {
return x * x;
}
// in app.js
export k from './util.js';
console.log(k(4)); // returns 16
That second export should be an import, shouldn't be?
ah yes, thanks for the correction Alex :) glad could help you learn something
Hey thanks, I just have to jump right into React, and this was useful, although you come across with it daily you don't think the whys sometimes...
Thanks again.
Great post!
I'd like to also mention .forEach() and .reduce() which are not quite as commonly used as .map() and .filter() but still worth noting. :)
Thank you Nathan for a great and informative post
I am begginer to javascript and know some basics of it so from where should I start to learn react native. I mean prerequisite.