Are you a fan of console.log
when you debug (instead of using debugger
)? Or you want to make better logging for your scripts / applications?
You are in the right place! In this article, I gonna show you some console methods that you probably don't know that are going to make your logs better :)
Log with style: console.log
Okay, I'm sure you know this one. But did you know you can stylized your text.
You can do this by putting %c
and defining the style in the following parameter (inline css format) before the text you want to stylize.
console.log(
"%c This is a stylized text",
"color:red;text-decoration: underline;"
);
```
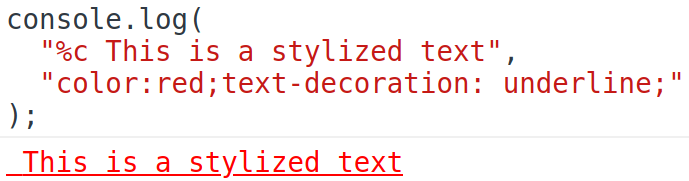
> **Note:** You can put multiple different stylized text in a same log:
```javascript
console.log(
"%c This is a red text %c and a blue text",
"color:red",
"color:blue"
);
```
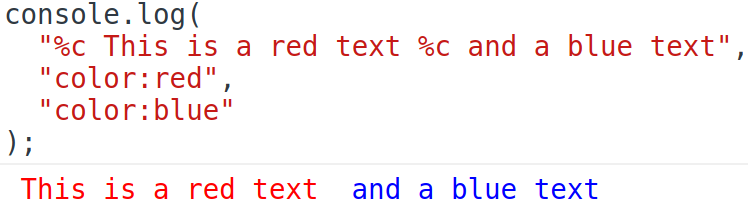
> **Note:** You can do it with other logging function like `info`, `debug`, `warn` and `error`.
----
## Make a quick counter: `console.count`
How many times when doing `React` you wanted to see how many times a component renders? Yep you can see it with the **React Developper Tools** but it's not enough quick for me :)
So you can make a counter thanks to `console.count`:
```javascript
function MyComponent() {
console.count("Render counter");
return <p>A simple component</p>;
}
```
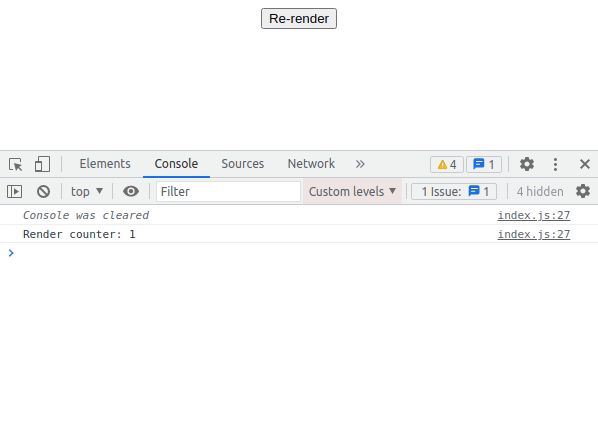
> **Note:** The label is optional, by default it will be "default".
----
## Log error with assertion: `console.assert`
If you want to display an error message when a specific assertion is false you can use `console.assert`:
```javascript
const useMyContext = () => {
const myContextValues = useContext(MyContext);
// You probably want to throw an error if it happens
// It's only an example
console.assert(
myContextValue === undefined,
"useMyContext has to be used below MyProvider"
);
return myContextValues;
};
```

> **Note:** If you want to know more about React context performances issues, do not hesitate to read [my article](https://dev.to/romaintrotard/react-context-performance-5832).
----
## Full description of elements: `console.dir`
`console.dir` allows you to show a better description of objects. For example when you `console.log` a function it will only stringify the function, but with `console.dir` it will show you all properties:
```javascript
function myMethod() {}
console.dir(myMethod);
```
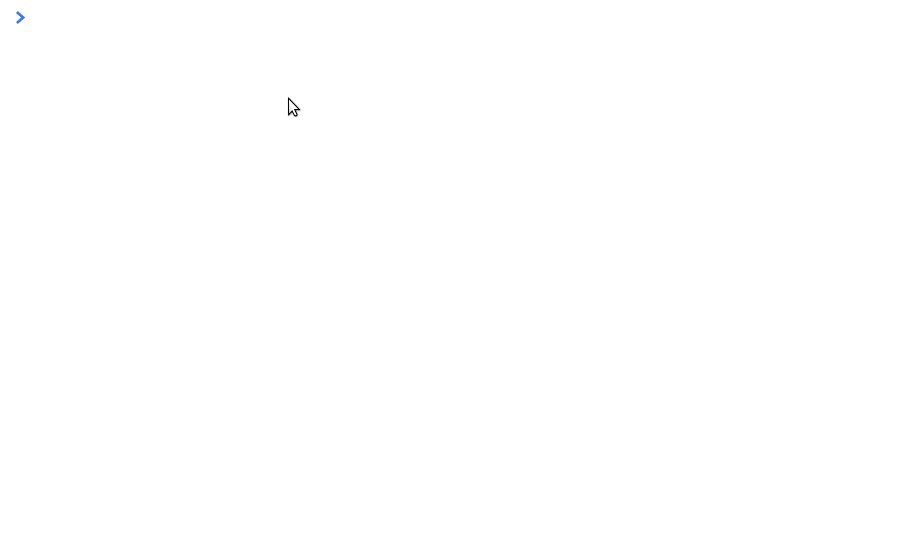
----
## Improve readability: `console.group`
If you have a lot of logs, it can be difficult to keep track of all these logs. Fortunately, `console.group` is here for you.
```javascript
function myMethod() {
console.group("My method optional label");
console.log("Log that will be group");
console.info("With this one");
console.error("And this one too");
console.groupEnd("My method optional label");
}
myMethod();
console.log('Outside log');
```
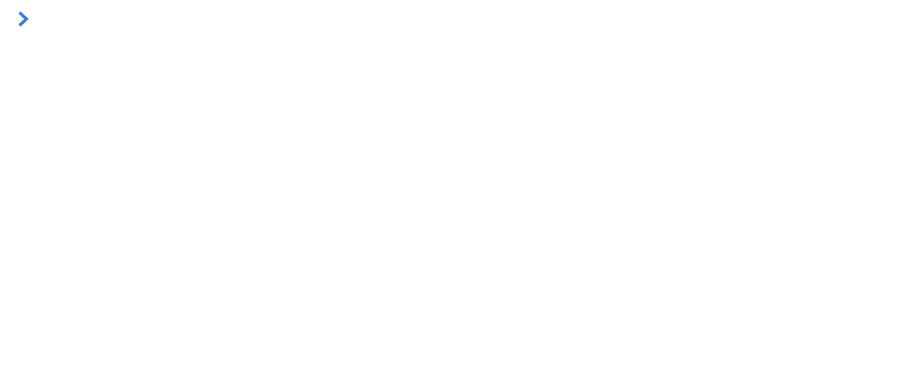
> **Note:** It's possible to nest `console.group`. The label is totally optional but can really help you for debugging.
----
## Make a nice table: `console.table`
If you want to display data inside a table, you can do it with `console.table`. The **first parameter** is the **data** to display (an array or object). The **second one** is the columns to display (optional parameter).
```javascript
console.table(
[
{
name: "First algo",
duration: "3.2s",
other: "Hello",
},
{
name: "Second algo",
duration: "4.1s",
other: "Guys and girls",
},
],
["name", "duration"]
);
```

----
## Make timers: `console.time`
When you want to see how long a method takes to run you can use `performance.now()` otherwise even easier `console.time()`, `console.timeEnd()` and `console.timeLog()`:
```javascript
function myMethod() {
console.time("A label");
// Do some process
// If you want to log the time during the process
console.timeLog("A label");
// Other process
// Will print how long the method takes to run
console.timeEnd("A label");
}
myMethod();
```

> **Note:** The label is optional, it will have the "default" label. You cannot start a timer with the same label than already running one.
----
## Display stacktrace: `console.trace`
If you want to know where is called your function then `console.trace` is your friend and will display the stack trace:
```javascript
function children() {
console.trace('Optional message');
}
function parent() {
children();
}
parent();
```
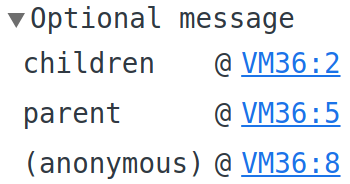
----
What is your favorite console command?
Do not hesitate to comment and if you want to see more, you can follow me on [Twitter](https://twitter.com/romain_trotard ) or go to my [Website](https://www.romaintrotard.com).
Top comments (0)