This article assumes that you have SFML configured and know how to compile and run it.
About SFML
Simple and Fast Multimedia Library or SFML provides a simple interface to the various components of your PC, to ease the development of games and multimedia applications. It is composed of five modules: system, window, graphics, audio and network. It is also cross-platform which means for simple program that you would want to run on different types of operating systems, you can without a lot of effort.
Source: sfml-dev.org
Insertion Sort
To sort an array of size n:
Step 1: Iterate from arr[1] to arr[n] over the array.
Step 2: Compare the current element to its predecessor.
Step 3: If the key element is smaller than its predecessor, compare it to the elements before. Move the greater elements one position up to make space for the swapped element.
For more info check out geeksforgeeks explanation on this.
Libraries Used
- SFML
- unistd (used for the sleep function, may vary based on one's OS)
Rendering a new window in SFML
#include<SFML/Graphics.hpp>
int main(){
sf::RenderWindow window(sf::VideoMode(600, 600), "Sample SFML App");
while (window.isOpen()){
sf::Event event;
while (window.pollEvent(event)){
switch (event.type)
{
case sf::Event::Closed:
window.close();
break;
}
}
}
}
Insertion Sort Function:
This function implements insertion sort algorithm and it sorts the recH[] array (initialization in the full code)
and also calls the dispSort() Function in every iteraton (more on this function below).
Implementing Insertion Sort
void insertionSort()
{
usleep(microsecond*5);
int i, key, j;
for (i = 0; i < n; i++)
{
key = recHs[i];
j = i - 1;
while (j >= 0 && recHs[j] > key)
{
recHs[j + 1] = recHs[j];
j = j - 1;
dispSort(j);
}
recHs[j + 1] = key;
}
sorted = true;
dispSort(i);
}
```
###The dispSort() Function
The dispSort() function is called in every iteration of the insertion sort loop, what it does is creates rectangular blocks based on the height data in the recH[] array, the same array being sorted.
```
void dispSort(int index){
window.clear();
for(int i=0; i<n; i++){
sf::RectangleShape block(sf::Vector2f(10, recHs[i]));
block.setPosition(i*12, 600-recHs[i]);
block.setFillColor(sorted || i==index? sf::Color::Green : sf::Color::White);
window.draw(block);
}
window.display();
}
```
*Note: the index perimeter is used for the indicator of the current block being sorted.*
###Full Code
```
#include<SFML/Graphics.hpp>
#include<unistd.h>
sf::RenderWindow window(sf::VideoMode(960, 600), "Sorter");
int n=80;
float recHs[80];
unsigned int microsecond = 1000000;
bool sorted=false;
void dispSort(int index){
window.clear();
for(int i=0; i<n; i++){
sf::RectangleShape block(sf::Vector2f(10, recHs[i]));
block.setPosition(i*12, 600-recHs[i]);
block.setFillColor(sorted || i==index? sf::Color::Green : sf::Color::White);
window.draw(block);
}
window.display();
}
void insertionSort()
{
usleep(microsecond*5);
int i, key, j;
for (i = 0; i < n; i++)
{
key = recHs[i];
j = i - 1;
while (j >= 0 && recHs[j] > key)
{
recHs[j + 1] = recHs[j];
j = j - 1;
dispSort(j);
}
recHs[j + 1] = key;
}
sorted = true;
dispSort(i);
}
int main(){
for(int i=0; i<n; i++){
recHs[i]=(rand()%500);
}
while(window.isOpen()){
sf::Event event;
while (window.pollEvent(event)){
switch(event.type){
case sf::Event::Closed:
window.close();
}
}
if(!sorted){
dispSort(0);
insertionSort();
}
}
}
```
###Result
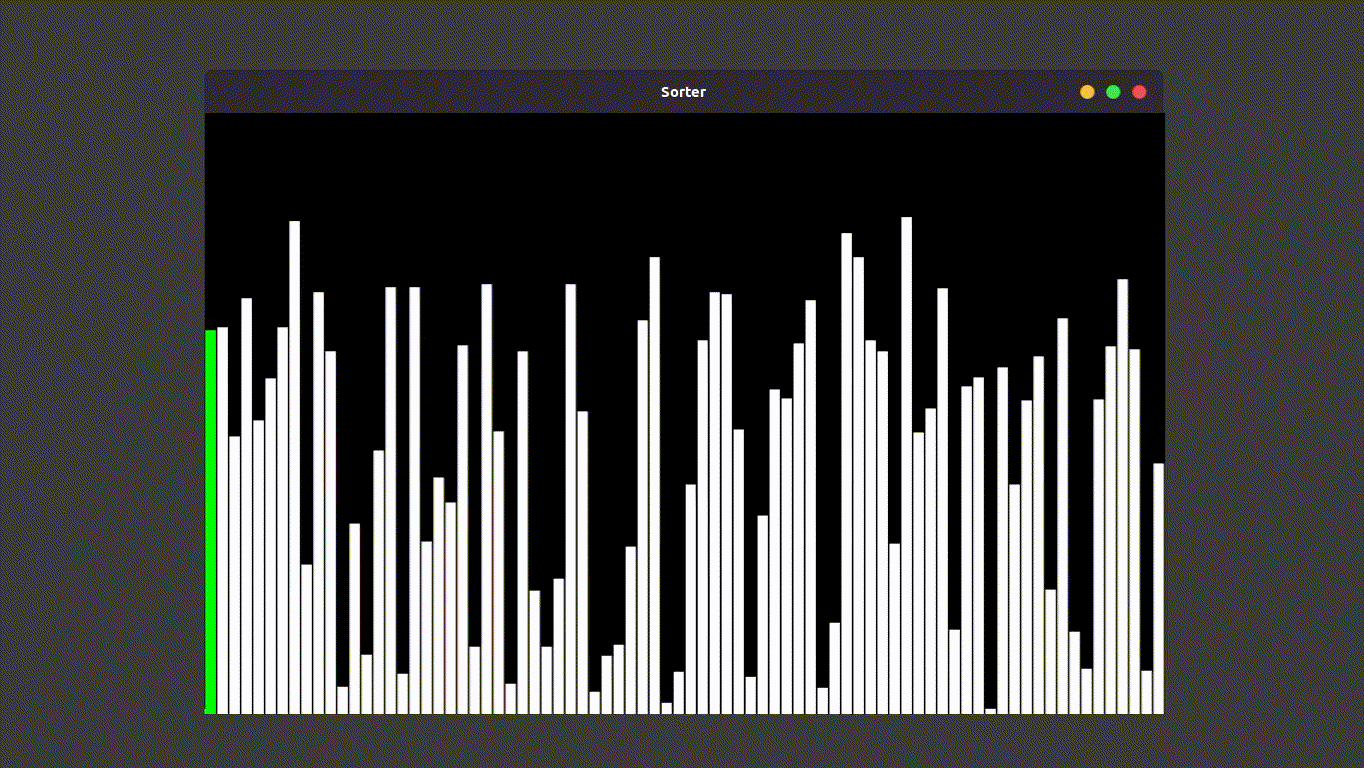
Top comments (2)
and on top of that you are using sf::rectangle which is not efficient instead use vertexArray or vertexbuffers those are more efficient ?
I am on Linux and this crashes on Linux ?