Have you ever felt lost and clueless in an interview when asked about Angular design patterns? Or perhaps you are new to Angular and wondering what these design patterns are all about. Well, let us jump in.
As developers, we often encounter common problems that require efficient and scalable solutions. This is where design patterns come in. Design patterns in Angular provide structured approaches to solving these challenges, promoting code organization, maintainability, and scalability. In this blog post, we will discuss the definitions, and their importance, and focus specifically on data-sharing patterns. I hope you enjoy this post :)
Design Patterns
Design patterns are a solution to common problems that developers encounter during application development. They provide a structured approach to solving certain challenges, promoting code organization and maintainability. So there are two categories of design patterns in Angular, that is Component-based Patterns and Structural Patterns.
A. Component-based Patterns
Singleton Pattern:
Singleton Pattern ensures that there is just one class throughout the application's lifecycle, which provides a global point of access to it. This works well when creating services that should have a single state. For example, let's check out the authentication service, this instance is shared among all components and services that inject it.
@Injectable()
export class AuthenticationService {
private isLoggedIn = false;
login() {
this.isLoggedIn = true;
}
logout() {
this.isLoggedIn = false;
}
}
Decorator Pattern:
The decorator pattern adds behavior or responsibilities to individual objects, either statically or dynamically, without affecting other instances. This can be achieved through decorators like @Component and @Directive in Angular, which enhance the functionality of components.
For instance, '@Component' decorator is used to define an Angular component.
@Component({
selector: 'app-store',
template: '<p>Decorated Component</p>',
})
export class StoreComponent {
}
Observer Pattern:
The observer pattern allows data sharing and event-driven communication among components. It establishes a one-to-many dependency between objects, allowing components to listen for data changes and update accordingly. Components can listen to data changes.
export class DataService {
private data: string;
private observers: Observer[] = [];
add(observer: Observer) {
this.observers.push(observer);
}
notify() {
this.observers.forEach(observer => observer.update(this.data));
}
}
B. Structural Patterns
Module Pattern:
The module pattern is the composer, organizing our code into modular pieces, each with its own purpose, making it easier to manage and update specific functionalities. This makes it reusable and promotes encapsulation and maintainability.
@NgModule({
declarations: [AppComponent, ExampleComponent],
imports: [BrowserModule],
providers: [DataService],
bootstrap: [AppComponent],
})
export class AppModule {}
Dependency Injection Pattern:
The dependency Injection Pattern enables components and services to request and share dependencies from the Angular Injector. It is the core concept in Angular and simplifies managing component dependencies and improves testability:
@Component({
selector: 'app-root',
template: '<p>{{ message }}</p>',
})
export class AppComponent {
constructor(private dataService: DataService) {}
message = this.dataService.getData();
}
Conclusion
By understanding and effectively utilizing Angular design patterns, developers can greatly enhance their skills and create robust, scalable, and maintainable applications. These patterns provide proven solutions to recurring problems, promote code organization and maintainability, improve code reusability and scalability, simplify managing component dependencies, and enhance code readability. Happy coding!😊
Top comments (8)
Can anyone help me with my Angular code?
Yah sure.
thanks for the response. how do I get to share a screenshot with you?
I guess you can post it right here and we try to solve the problem
okay I'm trying to create a sidebar navigation pane that when any of the navigation is clicked on, it will switch to another component with data init.
WHAT I HAVE DONE SO FAR
WHAT I'M TRYING TO DO
SCREENSHOTS OF MY CODE, SERVICE AND TEMPLATE

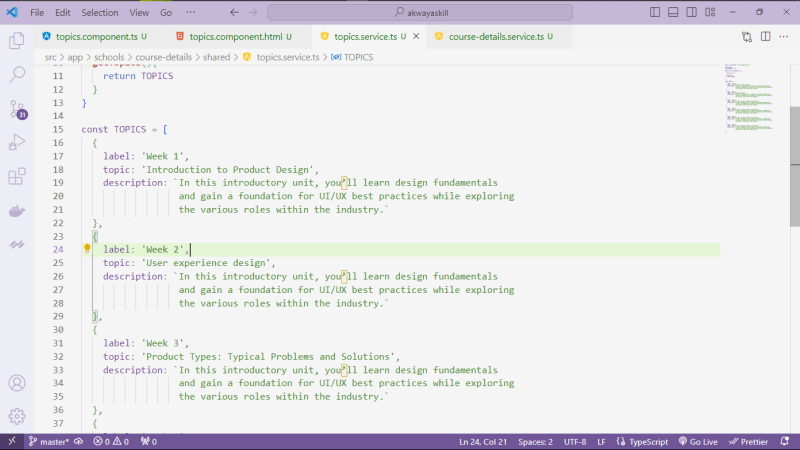
above are the screenshots
Hi, sorry for the late reply, is there a platform we can use to communicate easily?
How about twitter.com
Here's my handle twitter.com/big_zetsu, pls share yours so I can follow you right away. Thanks for being responsive. I really appreciate this.
Hi there,
Maybe you need this?
Link.
Cheers!