Online coding is a crucial part of software developer job interviews. In this article, I will try to add several coding questions. These will be evaluation focused code snippets instead of task based coding questions. Additionally, I will share online code links at the end of every question.
Let’s start with one of the most well-known one:
-
In this question, closure and asynchronous code handling knowledge is assessed.
// What will be the console log of the code below? for (var i = 0; i < 4; i++) { setTimeout(() => console.log(i), 0); }
It will write 4, 4, 4, 4 in console because
setTimeout()
is a
asynchronous function and it will be executed afterfor
cycle is completed.i
is defined outside scope offor
loop and it will be equal to 4 whenconsole.log()
starts to write.
How can you fix it to write 0, 1, 2, 3? Here is the possible solutions:
// Solution 1: for (let i = 0; i < 4; i++) { setTimeout(() => console.log(i), 0); } // Solution 2: for (var i = 0; i < 4; i++) { (function (i) { setTimeout(() => console.log(i), 0); })(i); }
Solution 1: You can change declaration of
i
fromvar
tolet
becauselet
is block scoped variable butvar
is function scoped variable.
Solution 2: You can wrapsetTimeout()
with a function to limit
the scope ofi
variable. But you should passi
as parameter to your IIFE (Immediately-invoked Function Expression).You can test it in below:
-
This one is about scope of this.
// What will be the logs in console? function nameLogger() { this.name = 'halil'; console.log('first:', this.name); } console.log('second:', this.name); nameLogger(); console.log('third:', this.name);
Console output will be following:
second: undefined first: halil third: halil
While “second” is executed
this.name
is undefined but afternameLogger()
function is executed it will be defined. Because,this
refers to the global object in a function. So the others log "halil" as expected.
!! It will not work with'use strict'
because in a function, in strict mode,this
equalsundefined
instead of global object.You can test it in below:
-
The last one is about
this
andbind
// What will be written? const module = { x: 55, getX: function () { console.log('x:', this.x); } }; const unboundX = module.getX; unboundX();
Console output will be
x: undefined
becausethis
refers to current owner object. So when you assign onlygetX
method to a new objectunboundX
,this
will refers to it. And it has nox
property, that’s whythis.x
equals toundefined
.
You can fix it by usingbind()
as below:
const boundX = unboundX.bind(module); boundX();
Now you bind module object to
unboundX
function. Then it can reach thex
property of module.You can test it in below:
You can read some of my other articles from the links below:
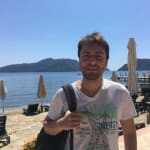
JavaScript Interview Coding Questions — 2
Halil Can Ozcelik ・ Jan 6 '20 ・ 2 min read
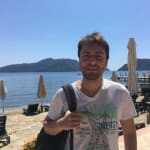
Top comments (0)