Today, I am going to drop a simple hint, how to implement search functionality in your crud app, this will allow you to just get a specific item or items that have a similar name from the search result in a list of hundreds or even thousands of data from the database.
I am going to modify our index method from our previous app Laravel 8 CRUD, in case you just need the code, you can get it from the GitHub repo.
Click on my profile to follow me to get more updates.
Step 1: Modify the index method in the project controller
- Add Request $request as a parameter to the Index method
- Edit the query to get all the projects
- In the first parameter for our “where” clause, we are going to only query the projects that the name is not null.
- In the second parameter, we are going to query the projects that the request is bringing, in our case where the request is similar to the name of the project.
- You can choose to order how your search results show up, I order mine according to the descending order of id.
Step 2: add the form that will send the request to the controller in index.blade.php
I added the following
- An input tag (where the user will enter the text to search).
- A submit button (This will trigger the search functionality after the text has been added).
- Another button to refresh our search result. ```
<div class="input-group">
<span class="input-group-btn mr-5 mt-1">
<button class="btn btn-info" type="submit" title="Search projects">
<span class="fas fa-search"></span>
</button>
</span>
<input type="text" class="form-control mr-2" name="term" placeholder="Search projects" id="term">
<a href="{{ route('projects.index') }}" class=" mt-1">
<span class="input-group-btn">
<button class="btn btn-danger" type="button" title="Refresh page">
<span class="fas fa-sync-alt"></span>
</button>
</span>
</a>
</div>
</form>
</div>
</div>
</div>
That is all, this is our result below
**all the projects in the database**
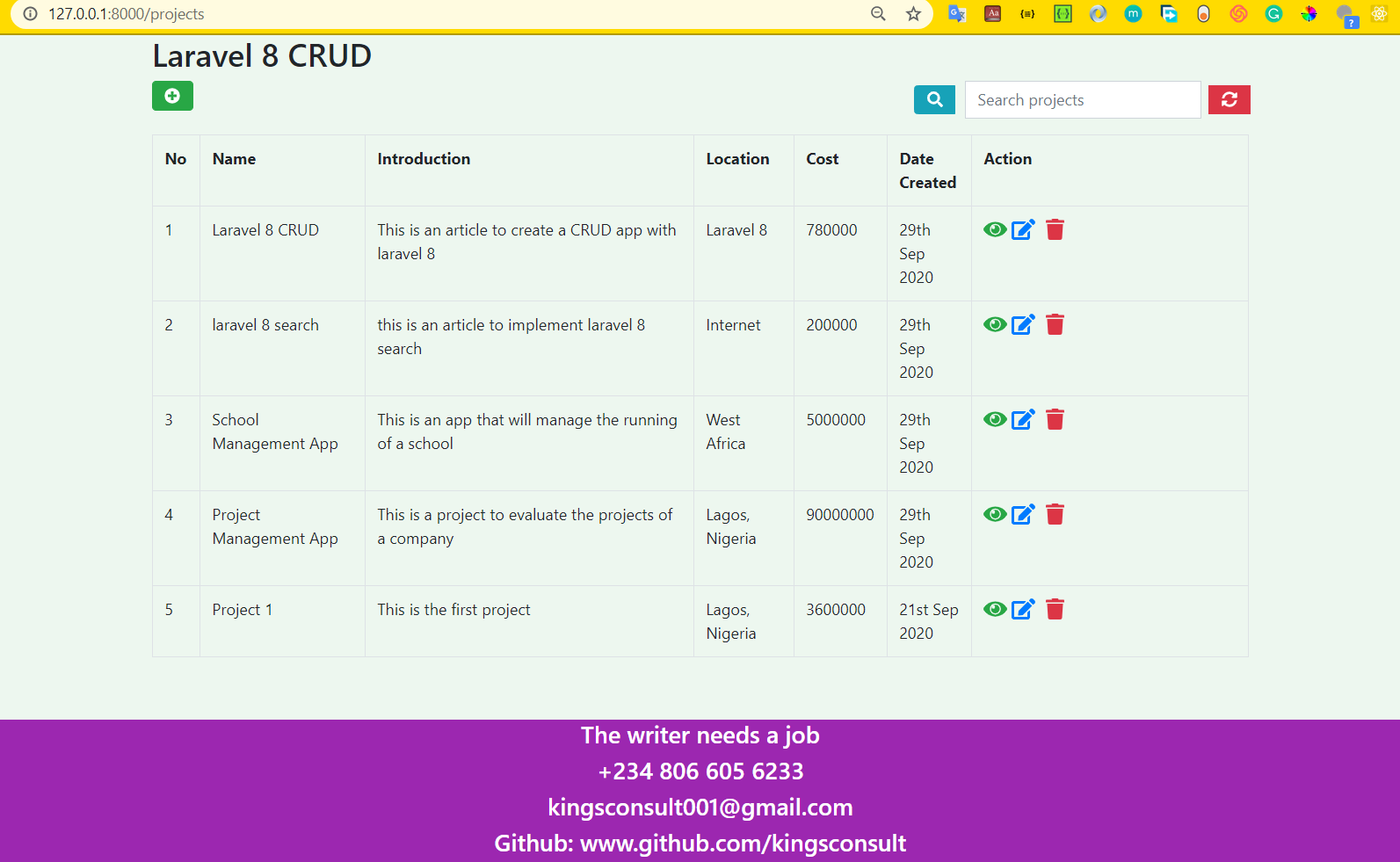
**only projects with laravel in the name**
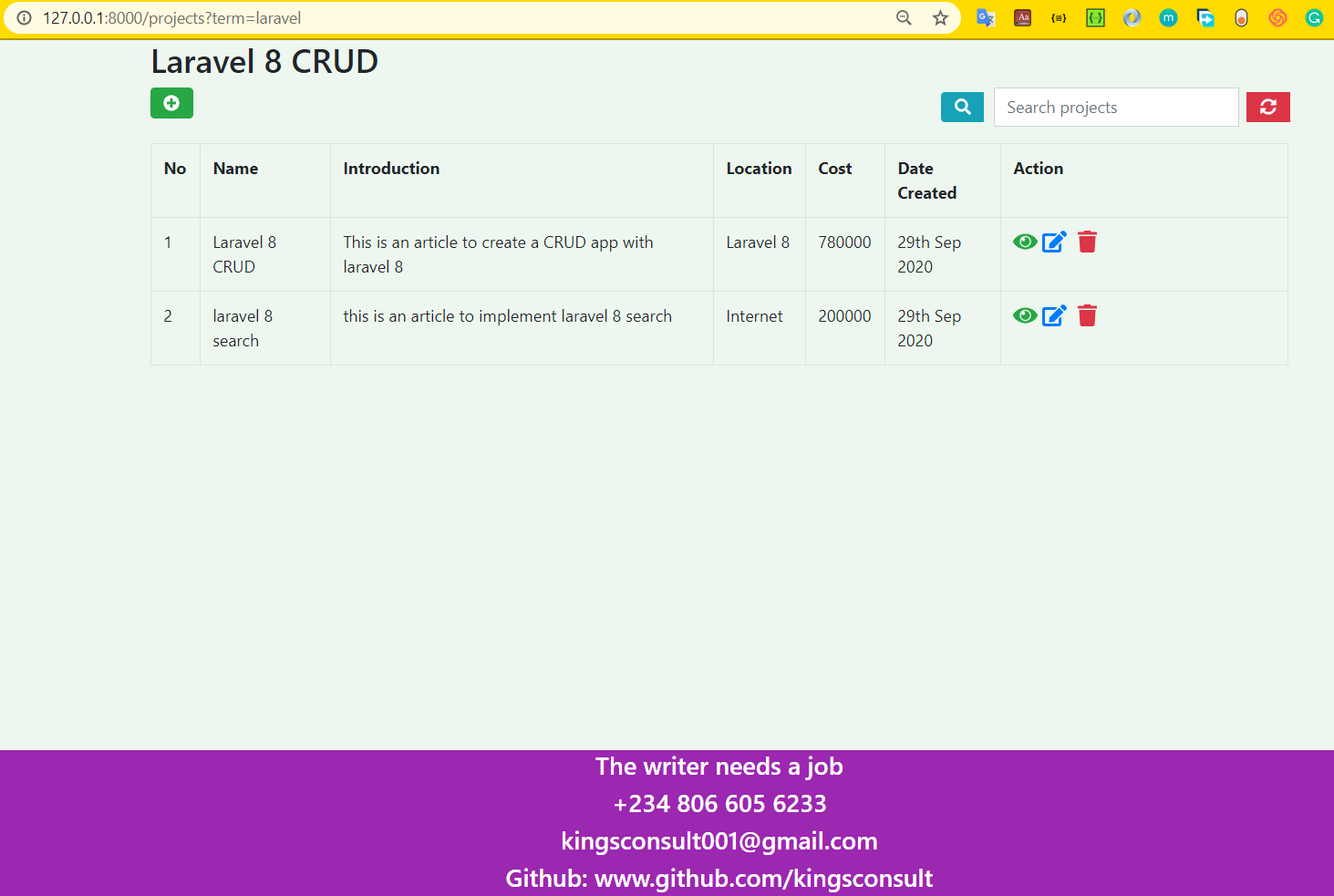
**only projects with mana in the name**
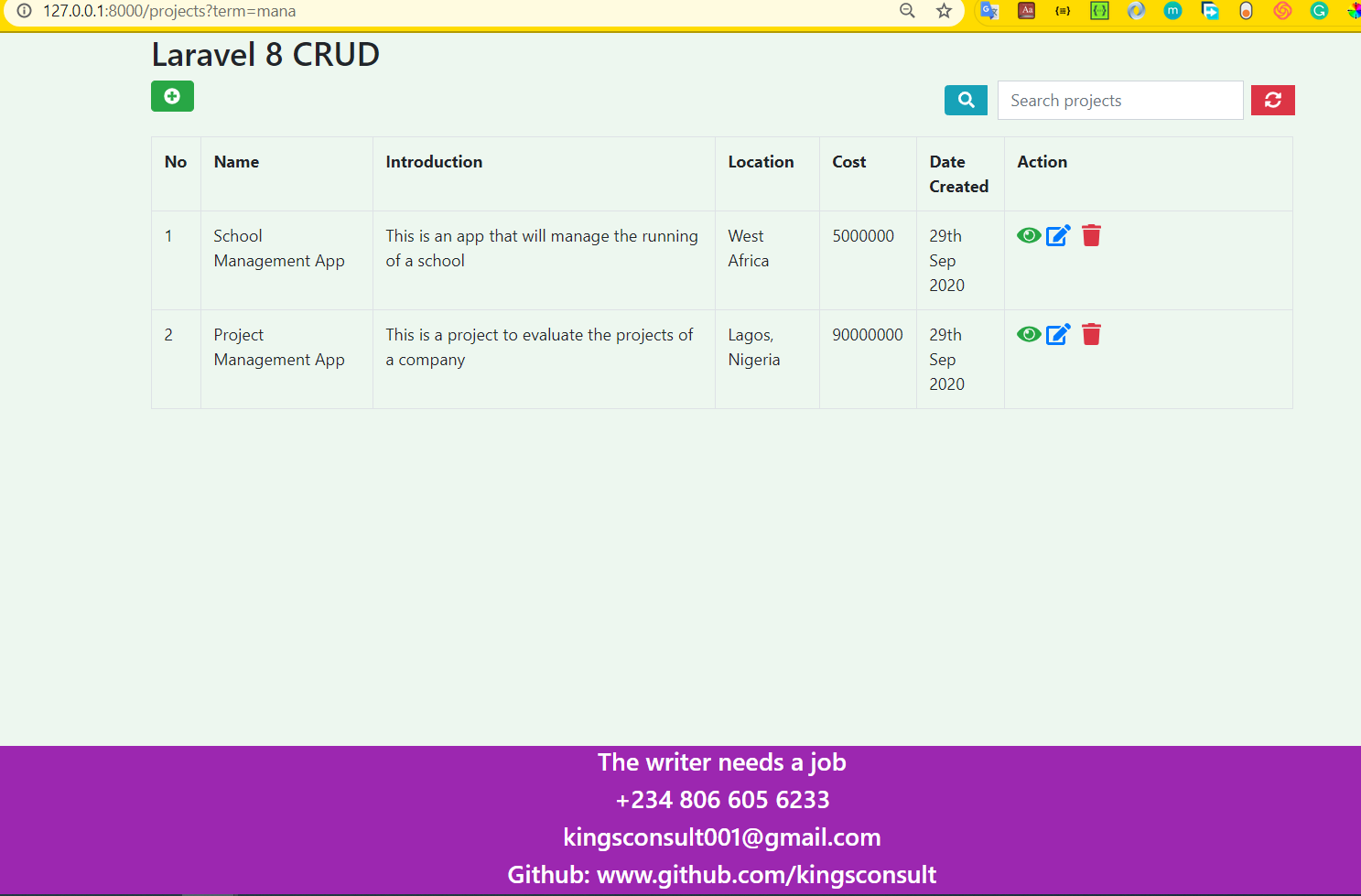
**only projects with 1 in the name**
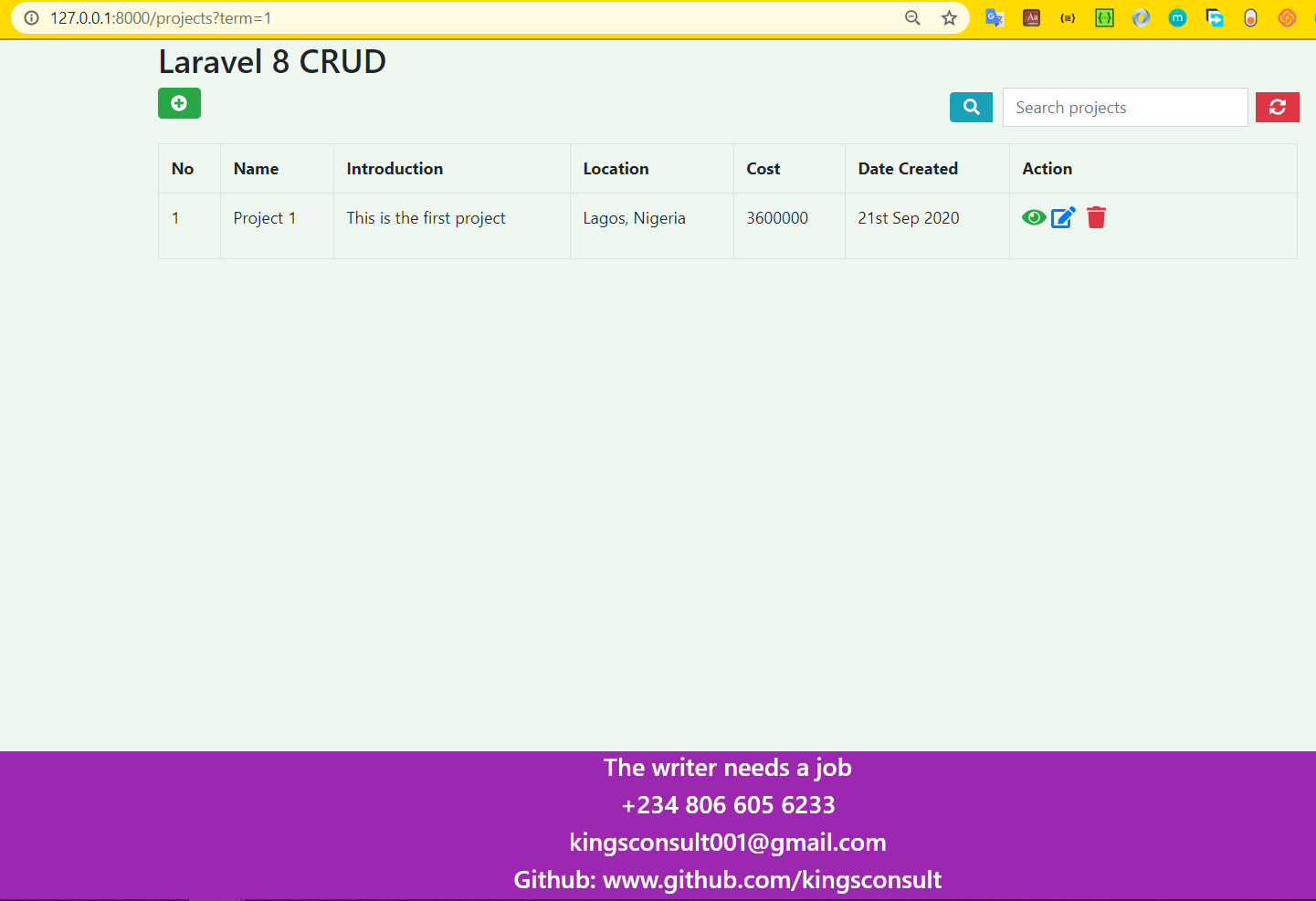
as usual, You can follow me, leave a comment below, suggestion, reaction, or email me.
Click on [my profile](https://dev.to/kingsconsult), to see my other post and contacts
Top comments (9)
nice article. good job.
but a much easier way can be as follows.
IN laravel you can break the query in pieces.
Hi Kings, Love your article, it really helps.
I'm a beginner although not a total beginner(lol), I'm actually trying to accomplish this with Laravel 7, I'm working on a blog site(my first Laravel site actually), after doing all you said and replacing the projects with post for my own project, I'm getting an error of "Route [post.index] not defined, Please how can I solve this, waiting for your response, cheers
you don't need to change your route from GET to POST, if you are using resource route, just add the Request $request to the index, it will work
I didn't change the method, I'm still using get, I only changed where you had projects.index to posts.index because mine is a blog, I want the search to work users blog posts
Unless I see your code, I can't be sure
If you still getting the errors, you can contact me through my contacts, so we can go through it together
Nice!
If you want to have a advanced filter in Laravel, you can check out this package:
github.com/mohammad-fouladgar/eloq...
hello, thanks for the tutorial.... but why is the search button position in my project not the same as yours? Please help 😅
Hey good job Kings,
Loved the article. Helped me to learn something new with query building in laravel.