So as we can see in the pictures above, despite having produced the expected output, our test case fails due to a runtime error EOFError i.e., End of File Error. Let's understand what is EOF and how to tackle it.
What is EOFError
In Python, an EOFError is an exception that gets raised when functions such as input() or raw_input() in case of python2 return end-of-file (EOF) without reading any input.
When can we expect EOFError
We can expect EOF in few cases which have to deal with input() / raw_input() such as:
Interrupt code in execution using
ctrl+d
when an input statement is being executed as shown below
-
Another possible case to encounter EOF is, when we want to take some number of inputs from user i.e., we do not know the exact number of inputs; hence we run an infinite loop for accepting inputs as below, and get a Traceback Error at the very last iteration of our infinite loop because user does not give any input at that iteration
n=int(input())
if(n>=1 and n<=10**5):
phone_book={}
for i in range(n):
feed=input()
phone_book[feed.split()[0]]=feed.split()[1]
while True:
name=input()
if name in phone_book.keys():
print(name,end="")
print("=",end="")
print(phone_book[name])
else:
print("Not found")
The code above gives EOFError because the input statement inside `while` loop raises an exception at last iteration
Do not worry if you don't understand the code or don't get context of the code, its just a solution of one of the problem statements on HackerRank 30 days of code challenge which you might want to [check](https://www.hackerrank.com/challenges/30-dictionaries-and-maps/problem)
The important part here is, that I used an infinite while loop to accept input which gave me a runtime error.
###How to tackle EOFError
We can catch EOFError as any other error, by using try-except blocks as shown below :
try:
input("Please enter something")
except:
print("EOF")
You might want to do something else instead of just printing "EOF" on the console such as:
n=int(input())
if(n>=1 and n<=10**5):
phone_book={}
for i in range(n):
feed=input()
phone_book[feed.split()[0]]=feed.split()[1]
while True:
try:
name=input()
except EOFError:
break
if name in phone_book.keys():
print(name,end="")
print("=",end="")
print(phone_book[name])
else:
print("Not found")
In the code above, python exits out of the loop if it encounters EOFError and we pass our test case, the problem due to which this discussion began...
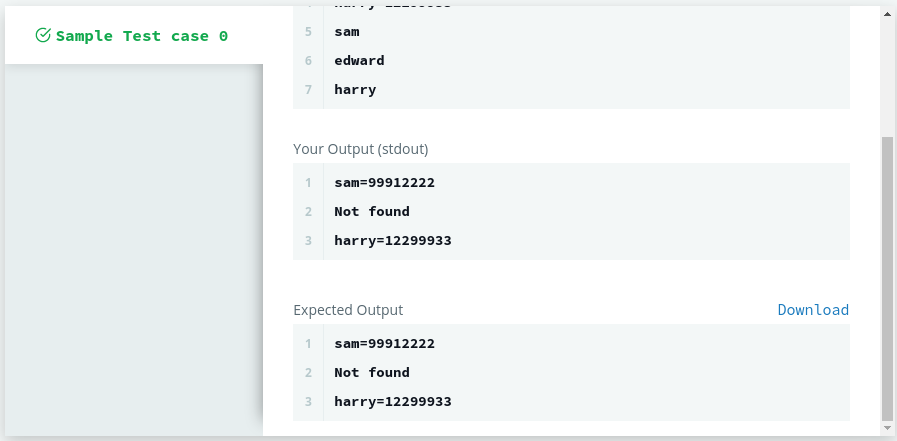
Hope this is helpful
If you know any other cases where we can expect EOFError, you might consider commenting them below.
Top comments (1)
It is, thanks!