How to use Pick/Omit/Exclude/etc.. to do cool stuff..
The pick is a utility .. utility type that allows you to select specific properties from an object type and create a new type with only those properties .. cool?
// Define a User interface
interface User {
name: string;
age: number;
address: string;
email: string;
single: boolean;
job: string;
degree: string;
}
// Now we r sliccccing ๐
type UserBasicInfo = Pick<User, 'name' | 'age'>;
const newUser: UserBasicInfo = {
name: 'Taric Ov',
age: 28
};
console.log(newUser); // { name: 'Taric Ov', age: 28 }
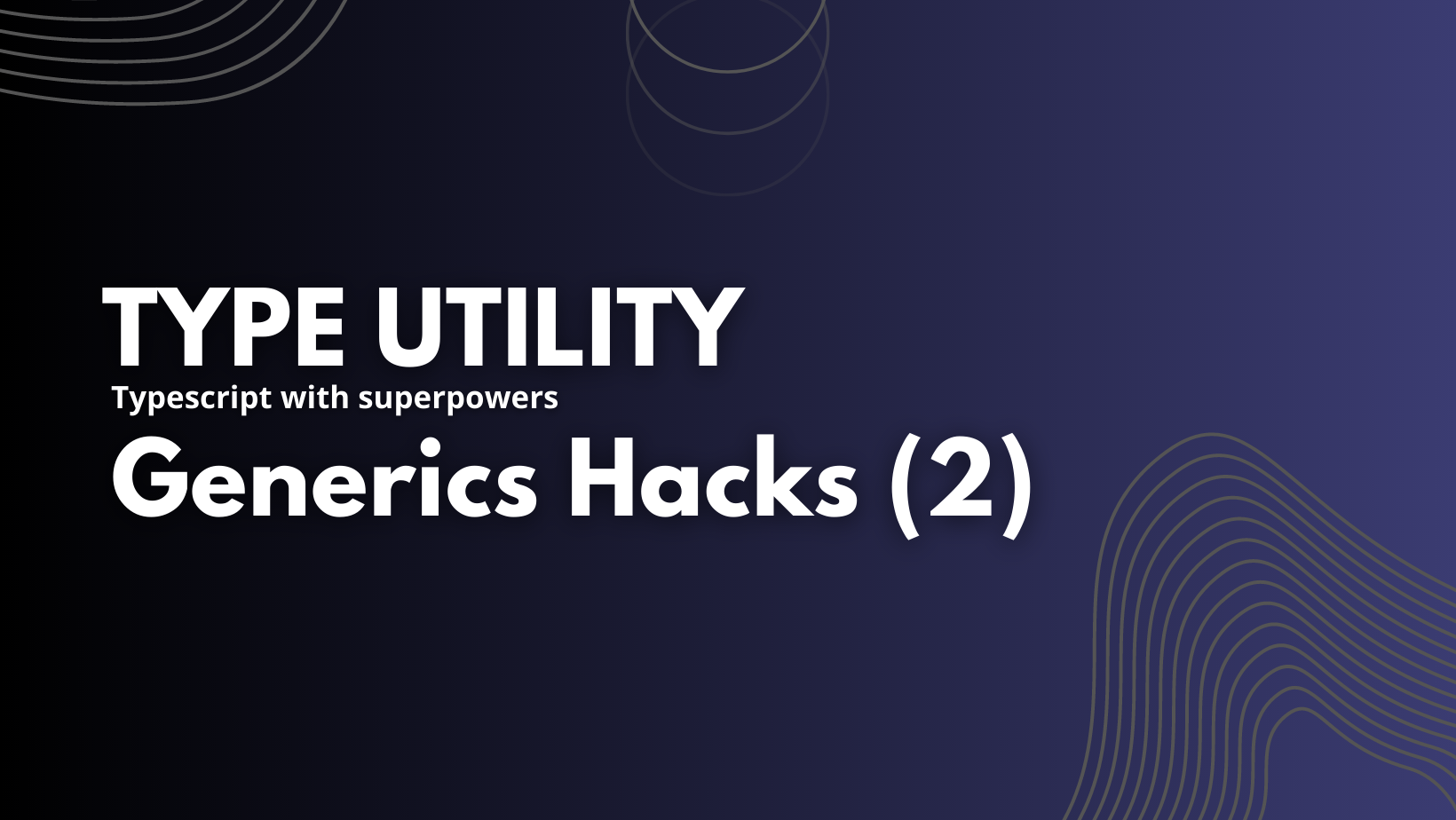
In the above example:
- We have an interface
User
that defines their properties. - We used the
Pick<User, 'name' | 'age'>
type to create a new type calledPersonBasicInfo
that only includes thename
andage
properties from the wholeUser
interface. - We then created an object of the type
UserBasicInfo
callednewUser
with the selected properties.
got it? if yes? that sounds great cuz u know what? you now got Omit/Exclude as well ๐ฎ๐ช congrats ๐
if still need more explanation? try it in code and follow the comments ๐
So..
Omit is also a utility type that does the opposite of Pick. It allows you to exclude specific properties from an object type and create a new type without those properties.
โ The terms โomitโ and โexcludeโ can be used interchangeably in the context of utility types in TypeScript. Both Omit
and Exclude
are utility types that provide similar functionality but with slight differences.
// Omitting
interface User {
name: string;
age: number;
address: string;
}
type UserWithoutAddress = Omit<User, 'address'>;
//Excluding numbers from a literal
type Numbers = 1 | 2 | 3 | 4 | 5;
type ExcludedNumbers = Exclude<Numbers, 1 | 3 | 5>;
// ExcludedNumbers: 2 | 4
What About the other utility types in TypeScript?
Partial: This utility type creates a new type that makes all properties of the original type
T
๐ optional.Required: This utility type creates a new type that makes all properties of the original type
T
๐ required.Readonly: This utility type creates a new type that makes all properties of the original type
T
๐ read-only.Record: This utility type creates a new type with keys of type
K
and values of typeT
๐ It's often used to define dictionaries or mappings.
These utility types, along with Omit
and Exclude
, provide powerful ways to manipulate and transform types and even to do cool tricks in TypeScript.. and that's what we are to explore in the next tutorial.
till then.. keep ur types tight ๐ช
Next: Type Templates ๐ค(โฆout the oven)
Top comments (2)
Thanks for sharing this.
Think many developers - even experienced ones - are not using this great features.
You very often see full typescript definition instead of simply re-using existing ones.
But the re-usage can really help to keep things consistent.
Because for example you add only one prop in one definition, and propagate it to all depending ones.
totally agree w/ Sebastian .. the perks of these utilities combined w/ generics could ease woking w/ types sooo simple, increasing readability and efficiency.