Edited to add demo of cart working upon request.
How I built a functional shopping cart:
TLDR: A react shopping cart! Code is on github here.
Shameless plug for my digital portfolio.
See cart in action (site under construction).
I know there are probably some easier ways to do things, but I couldn't find a shopping cart that just worked, so I decided to build my own. I am still really new to using React and programming in general, so any input or suggestions is greatly appreciated. This little project taught me a lot about callback functions, which are fun!
- My app calls the shopping cart with a list of items the user has chosen, via an "Add to Cart" button with event handler.
- I started with a stateless component which has a sole purpose to show my stateful components.
- To display each item, ProductDisplay took in the list of items, then mapped them on another "Display" Component.
- ProductDisplay has a state component that created a product list with default size, type, and quantity once mounted. This list will later be sent to checkout.
- The Display component shows each image and gives you the opportunity to change the type of print, size of print, and quantity to order.
- I created a size array and a type array to capture all the size and type options.
- We will retrace to the callback functions in a moment, but I want to quickly show the selectors change event handler. Type selector just renders the type by iterating through the Type Array.
- Do the above steps for any other options that require user input, such as size and options!
- As you can see, this initiates the props.callback function, which sends the child's state data to the parent. Here is the parent, the Display component, callback function.
- Another callback function, this time to ProductDisplay. Here is ProductDisplay's callback which updates the array that was created in step 4.

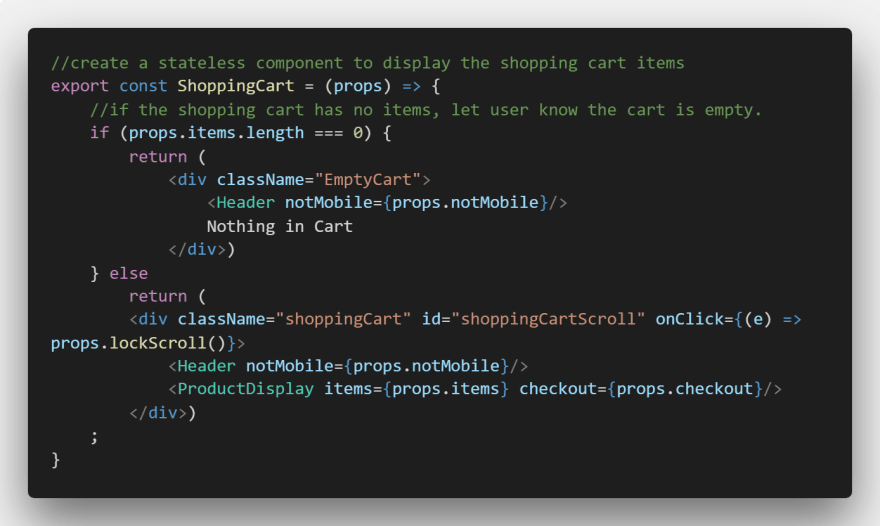







There you have it, if you do the above, you'll have a shopping cart. Feel free to steal my code and improve upon it. I'd also love to hear how you think it can be improved.
Top comments (6)
This is excellent! Your demo site works pretty much exactly like I think a shopping cart should work - I've been soooo disappointed lately, looking everywhere for help with this but just about every tutorial and article I found until now, most of them had such basic dumb carts that do not allow for removing items (only emptying the entire cart, all or nothing, like, who does that, really?) or not having options like variations for the item, i.e. small medium large, etc., so, I'm quite happy that yours actually works and has all the functionality.
This is a good starting point for my project. I will have to tweak it a little, because I'm using Gatsby and my data must be accessed via a Graphql query, but that shouldn't be a show stopper. Shouldn't. lol.
That being said, I wonder if you might be getting interested in using hooks or maybe even
useState
oruseEffect
? I'm currently learning on my own, and I have not quite gotten it to work yet.Take a look at this if you are interested; it's really well written and very thorough, and introduced those ideas to me just yesterday: Overreacted
Anyway, great job! ๐
Thanks Dre!
I've considered rebuilding this using hooks, I may do that if I have the time. Also, I have been thinking my rewrite will push all the shopping cart onto the Firebase server, then attach a listener that updates the render when the user adds or removes an item. Some firebase tools that make this easier are arrayUnion and arrayRemove.
Thanks for the feedback! I'm excited so many people have found use for this tool.
Thanks for this post! I have a question, you mentioned the async/await is so that the state can change upon update. Is it used so that the state can change without the use of buttons?
Yikes. There is no reason to use await when setting the state. That was a noob mistake by me. Whenever you use the this.setState function, that should trigger a re-render.
I think I was doing this because I had tried to use the state in a function directly after setting the state. The official React docs warn away from this use of state, noting that the state change is not instantaneous.
Edited to add: I have pushed a new version of the shopping cart that is much more robust, has fewer errors like the one described above, and includes the use of "Firestripe" payments. Feel free to check out the github link!
2nd edit: Text directly from the React docs on setState():
setState() does not always immediately update the component. It may batch or defer the update until later. This makes reading this.state right after calling setState() a potential pitfall. Instead, use componentDidUpdate or a setState callback (setState(updater, callback)), either of which are guaranteed to fire after the update has been applied. If you need to set the state based on the previous state, read about the updater argument below.
Thanks for the post andersjr1984 ๐
May I ask if you could post syntax highlighted codes next time?
(It'd make copy & paste easier like a boss ๐)
Awesome. I'll look that up and definitely do it next time!