Table of Content
- Introduction
- Stage 1: Prerequisite and Dependencies
-
Stage 2: Create the Chatbot Web Application
- Web Application Workflow
- Diagram
- 1. Setting Up the Environment
- 2. Understanding the Structure of a React App
- 3. Interact with aider-chat or LLM chatbot
- 4. Building the User Interface (UI)
- 5. Integrating a Basic Chatbot Logic
- 6. Connecting the Chatbot to OpenAI
- 7. Testing and Debugging
- 8. Enhancing the Chatbot
- 9. Final Touches
Introduction
NOTE: You can follow the steps by yourself but will need your parents' help to set up the project.
NOTE: Here is a repository for your reference. Do not clone it as you have to build it from scratch!
This project will help you create a chatbot web application tailored to your needs with the help of ChatGPT or any other LLM chatbot.
My son used this project to build his own chatbot, so I thought this would be a fantastic opportunity for others to embark on the same journey of learning through hands-on experience. You may follow the steps exactly or make changes as needed. You do not need to clone any repository or code as you will have to create everything yourself by following the instructions and asking ChatGPT.
What is Coding? Think of coding like giving instructions to a computer so it can do cool things, like making a game or building a website!
What’s a Web App? A web app is something you use on the internet, like a website, but it can do more interactive things, like letting you chat with a bot.
-
Tools We’ll Use:
- Github repository: This is a code version control where you will save your code. It is like a folder of files
- VSCode: This is like a super cool notebook where you write your instructions (code).
- Linux: The computer system you’re using. It’s a bit different from Windows or Mac, but perfect for coding.
- Gitpod*: This has the Linux where you will run VScode. Cloud-based, instant access, and pre-configured workspace. It's like Minecraft world
- ChatGPT: Your coding buddy! If you get stuck, you can ask ChatGPT for help.
We will use VScode workspace running on Gitpod as an IDE, you can use VScode on your local machine but you need to skip steps or change some details related to Gitpod. We will begin by setting up the workspace, preparing the requirements, and installing the dependencies.
* You can run VScode on your machine/PC
Stage 1: Prerequisites and Dependencies
We will begin by creating a Github repository to host the code. Then, we'll log in to Gitpod to set up the VSCode workspace and create the necessary requirements and dependencies. In this project, we will utilize Aider-chat as the terminal interface to the LLM to assist in building the application and for creating and updating the code directly. Alternatively, you can use the LLM chatbot at chatgpt.com to copy code back and forth.
Create a Github Repository
- Login to Github or create an account if you do not have one.
- Create a new repository
- Click on the repository then on the green "Code" button to copy the repository url
- Copy the Https url which is similar to
https://github.com/MyAccount/MyRepo.git
Set up your workspace on Gitpod
- Login to Gitpod or create an account using the Github account.
- Open a new workspace using the Github repository url you have copied before.
- Create .gitignore file in the navigation panel
- Run the following command from the terminal
bash echo -e 'node_modules/ \n**/.env' > .gitignore
- Create .gitpod.yml file then add the following
bash tasks: - name: init before: | cd my-chatbot-app npm i cd .. ./envload python -m pip install aider-chat ports: - name: react port: 3000 onOpen: open-browser visibility: public
OpenAI API Key
In this step, we will generate an API key from OpenAI. ( you can generate API key from any other LLM)
- Create an OpenAI account if you do not have one.
- login to OpenAI platform
- Click on API keys on the left-side menu
- Generate a new API key. save this key because you'll need it in the next steps. >> Note: Keep this API key secure and do not share it publicly.
- Add OpenAI API Key to the workspace Environment Variables
- Go back to the Gitpod workspace
- Run the following commands in the terminal:
bash export OPENAI_API_KEY="your_api_key_here" gp env OPENAI_API_KEY="your_api_key_here" # this will save the API key to Gitpod Environment Variables
>> Your workspace is ready, to activate these changes start a new workspace after completing step 2 in the next stage.
Integrate with Aider Chat (Optional)
Aider is AI pair programming in your terminal. Aider lets you pair program with LLMs, to edit code in your local git repository. Start a new project or work with an existing git repo.
Aider works with most of the well-known LLM models.
Note: Google currently offers free API access to the Gemini 1.5 Pro model.
Install Aider Chat
- Run the following command inside the root directory of the workspace
python -m pip install aider-chat
- Set the LLM API key if you have not set it in the previous steps eg:
export OPENAI_API_KEY=<key>
- Now you can run
aider
inside the terminal to star - Aider uses gpt-4o by default - You can use
aider --model <model-name>
to use any other model. For example, if you want to use a specific version of GPT-4 Turbo you could doaider --model gpt-4-0125-preview
. - Example using Google Gemini: ```bash export GEMINI_API_KEY= # Mac/Linux
aider --model gemini/gemini-1.5-pro-latest
List models available from Gemini
aider --models gemini/
---
## Stage 2: Create the Chatbot Web Application
[Back to ToC](#table-of-content)
Use the following steps as a guideline, you can work with ChatGPT to change any step, code or details.
### Web Application Workflow
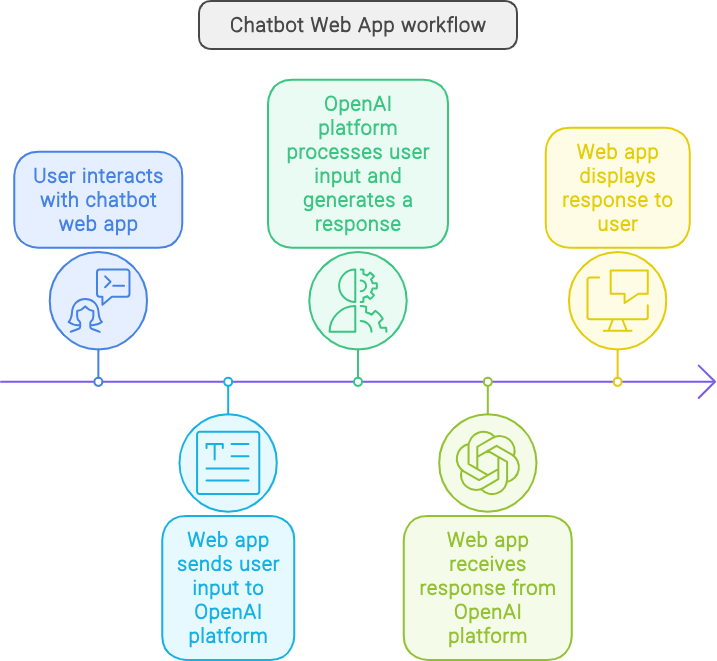
---
### Diagram
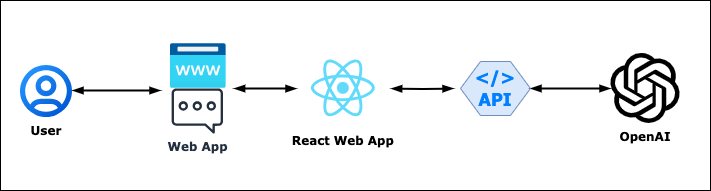
---
### 1\. Setting Up the Environment
[Back to ToC](#table-of-content)
* **Install Node.js and npm**: These are tools that help us build our app. Imagine Node.js as the engine that makes everything run, and npm is a big toolbox with lots of useful tools.
* Go to the **terminal** inside **VScode** (kind of like a text box where you type commands).
* Type: `sudo apt install nodejs npm` and press Enter. This will install Node.js and npm.
* **Install VSCode Extensions**: Extensions are like superpowers for VSCode that help you write code more easily.
* Go to the **Extensions** tab (it looks like four squares).
* Search for “JavaScript” and “React” and click “Install” on the ones you find.
### 2\. Understanding the Structure of a React App
[Back to ToC](#table-of-content)
* **Create a New React App**:
* In the **terminal**, type: `npx create-react-app my-chatbot-app` and press Enter. This will create a new project folder with all the files you need.
* Create envload script at the root directory which will generate **.env** file inside your working directory **"my-chatbot-app"**
```bash
#! /bin/bash
# Create the environment variable file using the local variable
echo -e "REACT_APP_OPENAI_API_KEY=$OPENAI_API_KEY" > my-chatbot-app/.env
```
* change the mode of envload by running this command: `chmod +x envload`
* run envload: `./envload`
* **Key Files in Your Project**:
* **index.js**: This is like the main door to your app. It’s where everything starts.
* **App.js**: Think of this as the brain of your app. It decides what’s shown on the screen.
* **public/index.html**: This is the basic page where your app will appear.
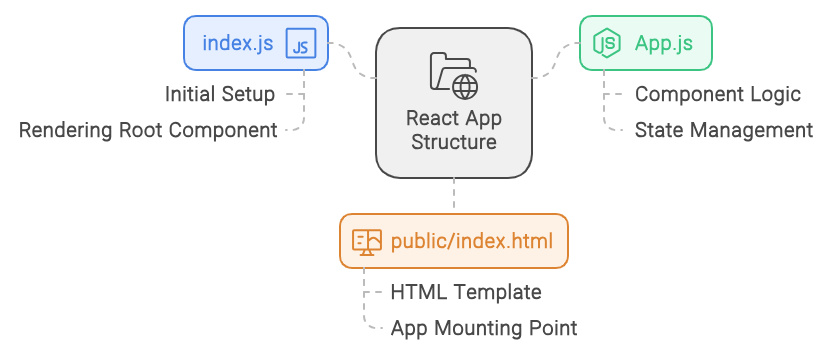
### 3\. Interact with aider-chat or LLM chatbot
[Back to ToC](#table-of-content)
As mentioned earlier, Aider-Chat will assist you in interacting with the LLM. This will enable you to create and update the code directly within the workspace without having to copy the code from ChatGP or any other LLM chatbot. You also have the option to use the LLM chatbot website to interact with the LLM.
Start by asking the LLM the questions in the following steps to help you create the code.
### 4\. Building the User Interface (UI)
[Back to ToC](#table-of-content)
* **Run the App**
* Let's run the application, type this in the terminal: `npm start`
* **Gitpod** will open a link to the app in another window (make sure popup-window is allowed)
* You will not see much, but you can refresh the page as you do changes in the code.
* **Let's start, make the App Look Cool**:
* Open App.js in VSCode.
* Create a simple box where people can type something (this is called an “input”) and a button they can press.
* Add some color and style using CSS. Imagine you’re decorating a cake – CSS makes your app look yummy!
1. Clear Out Unnecessary Code: If there’s any default code in App.js, you can remove it to start with a blank slate. Your App.js file should look something like this to start:
```js
import React, { useState } from 'react';
function App() {
return (
<div className="App">
{/* Your code will go here */}
</div>
);
}
export default App;
- Create an Input box - Add an Input Element: This is where users will type their messages.
function App() {
const [input, setInput] = useState(""); // This is where we store what the user types
return (
<div className="App">
<input
type="text"
value={input}
onChange={(e) => setInput(e.target.value)}
placeholder="Type your message here..."
/>
<button>Send</button>
</div>
);
}
-
Explanation:
- input is a state variable that holds the current text in the input box.
- setInput is a function that updates the input value whenever the user types something.
- The onChange event handler listens for changes in the input box and updates the input state.
- Add a Button to Send the Message
- Modify the Button: Add a click event to the button that will handle sending the message.
function App() {
const [input, setInput] = useState("");
const [messages, setMessages] = useState([]); // This is where we store all messages
const handleSend = () => {
if (input.trim() !== "") {
setMessages([...messages, { text: input, sender: 'User' }]);
setInput(""); // Clear the input box after sending the message
}
};
return (
<div className="App">
<input
type="text"
value={input}
onChange={(e) => setInput(e.target.value)}
placeholder="Type your message here..."
/>
<button onClick={handleSend}>Send</button>
</div>
);
}
-
Explanation:
- messages is a state variable that holds an array of all the messages in the conversation.
- handleSend is a function that adds the current message to the messages array and clears the input box.
- Display the Conversation History
- Add a Display Area: We’ll add a section to show the messages that have been sent.
function App() {
const [input, setInput] = useState("");
const [messages, setMessages] = useState([]);
const handleSend = () => {
if (input.trim() !== "") {
setMessages([...messages, { text: input, sender: 'User' }]);
setInput("");
}
};
return (
<div className="App">
<div className="chat-box">
{messages.map((message, index) => (
<div key={index} className={`message ${message.sender}`}>
{message.text}
</div>
))}
</div>
<input
type="text"
value={input}
onChange={(e) => setInput(e.target.value)}
placeholder="Type your message here..."
/>
<button onClick={handleSend}>Send</button>
</div>
);
}
-
Explanation:
- The chat-box div will display each message in the messages array.
- We use map to loop over each message and display it in a div.
- The className allows us to style messages differently based on whether they were sent by the user or the chatbot.
- Add Some Basic CSS for Styling
- Style the App: You can add CSS in a file like App.css to make the chat box look nice.
.App {
font-family: Arial, sans-serif;
padding: 20px;
max-width: 600px;
margin: auto;
}
.chat-box {
border: 1px solid #ccc;
padding: 10px;
height: 300px;
overflow-y: scroll;
margin-bottom: 10px;
background-color: #f9f9f9;
}
.message {
padding: 5px;
margin: 5px 0;
border-radius: 5px;
}
.User {
text-align: right;
background-color: #d1e7dd;
}
.Bot {
text-align: left;
background-color: #f8d7da;
}
input[type="text"] {
width: 80%;
padding: 10px;
margin-right: 10px;
border-radius: 5px;
border: 1px solid #ccc;
}
button {
padding: 10px 20px;
border-radius: 5px;
background-color: #007bff;
color: white;
border: none;
cursor: pointer;
}
button:hover {
background-color: #0056b3;
}
-
Explanation:
- .App centers the app and gives it some padding.
- .chat-box styles the area where the conversation is displayed, with a scroll bar for long conversations.
- .message styles each message bubble, and .User and .Bot give different colors to user and bot messages.
- input[type="text"] and button style the input box and send button to make them look nice and clickable.
5. Integrating a Basic Chatbot Logic
-
Making the Chatbot Talk:
- We’ll teach the chatbot to respond to what people type.
- Use something called “state” in React. State is like a memory for your app, where it can remember what the user typed.
- ChatGPT can help you write a simple script where the chatbot says something back when you type a message.
Understanding State in React
In React, "state" is a way to keep track of information in your app that can change over time. For a chatbot, we'll use state to store the conversation history (messages) and the user's input.-
Setting Up State Variables
We already have two state variables in place: input for the user's current input and messages for the conversation history. Let's review these:const [input, setInput] = useState(""); // Stores the current input from the user const [messages, setMessages] = useState([]); // Stores all the messages in the conversation
-
Creating a Function to Handle User Input
Ask ChatGPT to help you with this step!
When the user types a message and hits the "Send" button (or presses Enter), we need to:
Add the User's Message to the Conversation: We'll add the user's message to the messages array.
Generate a Chatbot Response: We'll simulate a chatbot response by adding another message to the messages array.
- Creating a Simple Chatbot Response Function Now, let’s create a basic function that simulates a chatbot response. This function can be as simple or complex as you like. For now, we'll keep it simple by making the bot respond with a predefined set of responses based on certain keywords.
const generateBotResponse = (userInput) => {
const lowercasedInput = userInput.toLowerCase();
if (lowercasedInput.includes("hello")) {
return "Hi there! How can I help you today?";
} else if (lowercasedInput.includes("how are you")) {
return "I'm just a bot, but I'm doing great! Thanks for asking.";
} else if (lowercasedInput.includes("bye")) {
return "Goodbye! Have a great day!";
} else {
return "I'm not sure how to respond to that. Can you ask me something else?";
}
};
-
Explanation of the Chatbot Logic:
- handleSend Function:
- Checks if the input is not empty.
- Adds the user's message to the messages array.
- Generates a response from the bot using generateBotResponse.
- Updates the messages array to include the bot's response.
- Clears the input field.
- generateBotResponse Function:
- Takes the user's input and checks for specific keywords.
- Returns a response based on the keyword found (e.g., "hello", "how are you", "bye").
- If no keyword is matched, it returns a default response.
6. Connecting the Chatbot to OpenAI
-
Chatting with ChatGPT:
- You’ll learn how to send what you type to OpenAI and get a response back. This is called calling an API (which is just a way to talk to other computers).
- ChatGPT will help you write this part. You’ll type something in the chatbox, and OpenAI will answer, just like ChatGPT!
Set Up an API Key
React should be able to load the API key saved as REACT_APP_OPENAI_API_KEY in the .env fileInstall Axios for API Requests
We'll use Axios, a popular JavaScript library, to make HTTP requests to the ChatGPT API. You can install it using npm:
npm install axios
-
Set Up the API Call in Your React App
Now, let's modify the handleSend function to send the user's input to OpenAI and receive a response.Here’s how to do it:
- Import Axios at the top of your App.js:
import axios from 'axios';
- Modify the handleSend Function to include the API call, update the code as required:
// Call the ChatGPT API
try {
const response = await axios.post('https://api.openai.com/v1/chat/completions',
{
model: "gpt-4o-mini", // Use "gpt-3.5-turbo" if you have access to GPT-4
messages: [{ role: "user", content: input }],
},
{
headers: {
'Authorization': `Bearer ${process.env.REACT_APP_OPENAI_API_KEY}`, // OpenAI API key
'Content-Type': 'application/json'
}
});
-
Explanation of the API Call
- API Endpoint: We are sending a POST request to https://api.openai.com/v1/chat/completions.
- Model: The model parameter specifies which version of GPT you are using. You can use "gpt-3.5-turbo" or "gpt-4o-mini" depending on your access.
- Messages: This contains the conversation history, with the user’s latest input being sent to the API.
- Headers: The request headers include the - Authorization header with your API key and specify that the content is JSON.
- Bot Response: Once the API responds, we extract the content of the bot’s response and add it to the messages array.
7. Testing and Debugging
-
Test Your Chatbot:
- Run your app by typing
npm start
in the terminal. - Try typing different things in the chatbox to see how it works.
- If something doesn’t work, don’t worry! Debugging is like solving a mystery. You’ll look for clues (called “errors”) in the console and figure out how to fix them.
- Run your app by typing
8. Enhancing the Chatbot
-
Add More Fun Features:
- Want your chatbot to do more? Maybe show a spinning wheel while it’s thinking or keep a history of the conversation.
- Ask ChatGPT for help with these ideas. You can keep adding new things to make your chatbot cooler!
9. Final Touches
-
Polish Your App:
- Clean up any messy code, and make sure everything looks just right.
In the next blog post, we will guide you through the steps to deploy the web application on the cloud so you can access it from anywhere.
Top comments (7)
What an awesome project, thanks for sharing!
Thank you for your usual support, this project was inspired by the community :)
Is there a github repo for this? Thanks for your work.
Hi John, here is the repo github.com/astroveny/kids-chatbot-...
The young builders will get the best experience if they follow the steps in the article, and work with the LLM from scratch. However, I will create a new repo as a reference and share it
Thank you so much for sharing this
You are welcome, hope it helps