UPDATED version of this tutorial available here
CREATE AND INSTALL
Create new react project
npx create-react-app react-data-to-excel
Now start project
npm start
Project successfully created.
Lets dive into installing neccassry libraries for our project. Install these three libraries.
npm install xlsx file-saver axios --save-dev
👉xlsx - library for parsing and writing various spreadsheet formats
👉file-saver - library for saving files on the client-side
👉axios - promise based HTTP client for the browser and node.js. We will use it for fetching data from server
UI AND LOGIC
Create a component namely ExportToExcel.js
import React from 'react'
import * as FileSaver from "file-saver";
import * as XLSX from "xlsx";
export const ExportToExcel = ({ apiData, fileName }) => {
const fileType =
"application/vnd.openxmlformats-officedocument.spreadsheetml.sheet;charset=UTF-8";
const fileExtension = ".xlsx";
const exportToCSV = (apiData, fileName) => {
const ws = XLSX.utils.json_to_sheet(apiData);
const wb = { Sheets: { data: ws }, SheetNames: ["data"] };
const excelBuffer = XLSX.write(wb, { bookType: "xlsx", type: "array" });
const data = new Blob([excelBuffer], { type: fileType });
FileSaver.saveAs(data, fileName + fileExtension);
};
return (
<button onClick={(e) => exportToCSV(apiData, fileName)}>Export</button>
);
};
Sample data we will get from https://jsonplaceholder.typicode.com/posts
App.js
import React from 'react'
import axios from 'axios'
import './App.css';
import {ExportToExcel} from './ExportToExcel'
function App() {
const [data, setData] = React.useState([])
const fileName = "myfile"; // here enter filename for your excel file
React.useEffect(() => {
const fetchData = () =>{
axios.get('https://jsonplaceholder.typicode.com/posts').then(r => setData(r.data) )
}
fetchData()
}, [])
return (
<div className="App">
<ExportToExcel apiData={data} fileName={fileName} />
</div>
);
}
export default App;
Start project
npm start
Once it successfully started. You can see one button. By pressing this button you download excel file with data filled from https://jsonplaceholder.typicode.com/posts
Output
Excel File
Github repo for this project link
Top comments (17)
Thanks. Amazing explaination. For those looking for multiple sheet writing, here is the code snippet.
const exportToCSV = () => {
const ws = XLSX.utils.json_to_sheet(sheet_data1);
var ws2 = XLSX.utils.json_to_sheet(sheet_data2);
const wb = XLSX.utils.book_new();
const sheet1 = XLSX.utils.book_append_sheet(wb, ws, "Name of Sheet1");
const shee2 = XLSX.utils.book_append_sheet(wb, ws2, "Name of Sheet2");
XLSX.writeFile(wb, "Output_filename.xlsx");
};
Thanks for the article but I don't think you need
👉file-saver - library
XLSX.writeFile(wb, 'sheetjs.xlsx')
does the job...Hi, I'm very intersting with your solution but how can I export columns that I want to export them. Example: I have id, displayID, name, age which of them are columns and I just want to export data from name, age.
Thanks for your supporting.
You need to change place where I am retrieving data from server. Add this code and analyze what I did. I put comments.
Screenshot after exporing data. Checkout
dev-to-uploads.s3.amazonaws.com/up...
P.s
Regarding code style, I did simple solution in order to give you idea. Obviously, you can do code more beautiful and shorter.
Hi, if to say data in json being exported is a boolean from, i tried writing a function to convert it from true/false to "yes" /"no". this is the result "TypeError: js.forEach is not a function" can you help explain why and what do you think i can do.
1) Download this repo github.com/jasurkurbanov/react-api...
2) Inside
App.js
replace useEffect to the code below3) Result
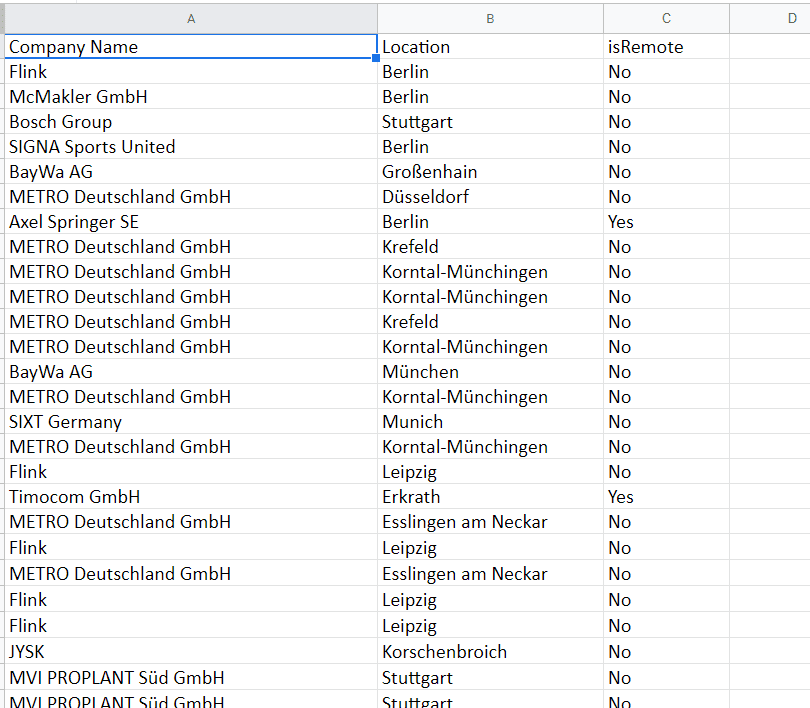
Hope it helped to you)
How can I put custom headers?
Can you show where you want to add headers ?
in the first row a title and in the second row are the column headings
Sorry I did not get, what you mean. If it possible please provide some screenshots , in order to figure out.
I wonder if it's possible to send styled excel? I mean at least to adjust columns width to fit data. Also it would be nice to make table borders like this:
Nice idea, thank you. I need to do research about your idea
Thank you buddy
Any update?
Checkout my new post here
Are there any updates on this? How can we add headers and style to the sheet?
Checkout my new post here