For further actions, you may consider blocking this person and/or reporting abuse
Read next

Level Up Your JavaScript Error Handling: From `try...catch` to Custom Errors
Zakir -

Understanding the "use client" Directive in Next.js 13
codenextgen -

Project Ideas Don’t Have to Be Unique: Here’s Why
Md. Maruf Sarker -
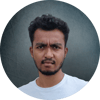
Building a CRUD Application with NestJS and MongoDB
Manthan Ankolekar -
Top comments (1)
Option-1 You can upload images to your server using multer(npm package) and then store your image link to mongodb.
Option-2 Upload image to firebase, get download link and store it to mongodb.
Option -3 AWS S3 storage but for beginners it's not recommended.