Predicting the result of an equality check of two or more values in JavaScript has been a part of the language that trips many developers; but not anymore, as this article stops at nothing in providing you with a straightforward and understandable approach to it.
This article does not include explanations for edge case comparisons such as
[] == ""
and[undefined] == [null]
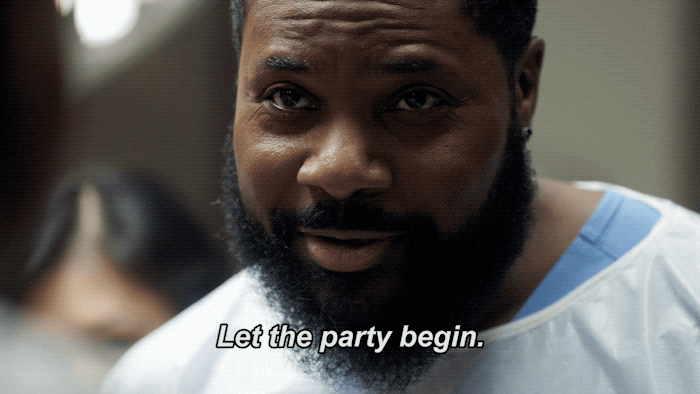
Introduction
Determining if any set of values are equal in JavaScript is achieved using any one of these:
- The Abstract Equality Operator (
==
) - The Strict Equality Operator (
===
) Object.is
The major difference between the strict equality operator and the abstract equality operator is NOT that the strict equality operator checks for the equality of the value types being compared and the abstract equality operator does not; but that the strict equality operator does not allow coercion before comparison, while the abstract equality operator allows coercion before comparison.
Irrespective of the operator used, the result of checking the equality of any set of values is either true
(if the values compared are equal) or false
(if the values compared are not equal).
Comparison with the Abstract Equality Operator (==
)
When comparing the equality of any set of values using ==
,
There are seven value types here:
undefined
,null
,Boolean
,Number
,String
,Object
andSymbol
.
if the value types of any of the set of values to be compared are the same, there is no need for coercion; hence, a strict equality comparison is performed and the result is returned, otherwise;
undefined
andnull
values are coercively equal to each other; in other words, testing ifundefined == null
will returntrue
.undefined
andnull
will not coerce to any other type (Boolean
,Number
,String
,Object
, orSymbol
) when==
is used to compare their equality with these other types;all
String
andBoolean
values are first coerced toNumber
values before a check for the equality of the values is determined. (TheBoolean false
is coerced to+0
, whiletrue
is coerced to+1
.);all
object
s (remember thatnull
is not anobject
in this case) are coerced to their primitive values before an equality check is carried out.
The primitive value of an object is determined internally by the JavaScript engine, however, depending on the object hint, the object is either coerced to a String
(in the case of arrays) or to a Number
.
Comparison with the Strict Equality Operator (===
)
When comparing the equality of any set of values using ===
,
if the value types (
Number
,String
e.t.c) of the set of values under comparison are different, the JavaScript engine avoids coercion immediately and returnsfalse
; otherwise,if the set of values under comparison are of type
String
and they are exactly the same sequence of code units (same length and same code units at corresponding indices), the JavaScript engine returnstrue
; otherwise, it returnsfalse
.if the set of values under comparison are of the
Boolean
type, the JavaScript engine returnstrue
if the values are the same, otherwise it returnsfalse
if the set of values under comparison are of the type
Symbol
and they have the sameSymbol
value, the JavaScript engine returnstrue
, otherwise, it returnsfalse
;if the set of values under comparison are of the
Object
type and they reference the same object in memory, the JavaScript engine returnstrue
, otherwise, it returnsfalse
.if any of the values under comparison is
NaN
, the JavaScript engine returnsfalse
;+0 and -0 Number values are equal to each other, therefore, return
true
.
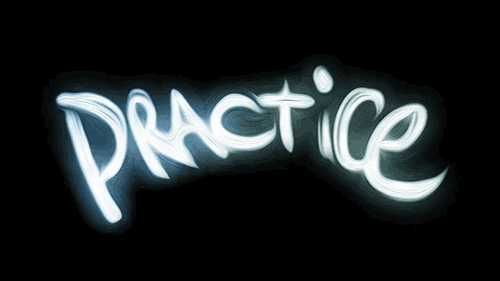
Practice Time!
What will be the result of the following comparisons?
0 == null
false == undefined
true == 1
'JavaScript' == true
'JavaScript' == false
Answers
false
.null
will not coerce to any other value exceptundefined
false
.false
gets coerced to its Number value (+0), butundefined
does not get coerced to its Number value (which is NaN). Since +0 is not the same asundefined
, false is returned.
You can answer the rest in the comments section, with an explanation for your answer, and also feel free to add your own questions too 😉.
Top comments (0)