Most of us are used to writing JavaScript code for a long time. But we might not have updated ourselves with new features which can solve your issues with minimal code. These techniques can help you write clean and optimized JavaScript Code. Today, I’ll be summarizing some optimized JavaScript code snippets which can help you develop your skills.
1. Shorthand for if with multiple || conditions
if (fruit === 'apple' || fruit === 'orange' || fruit === 'banana' || fruit ==='grapes') {
//code
}
Instead of using multiple || (OR) conditions, we can use an array with the values and use the includes() method.
if (['apple', 'orange', 'banana', 'grapes'].includes(fruit)) {
//code
}
2. Shorthand for if with multiple && conditions
if(obj && obj.address && obj.address.postalCode) {
console.log(obj.address.postalCode)
}
Use optional chaining (?.) to replace this snippet.
console.log(obj?.address?.postalCode);
3. Shorthand for null, undefined, and empty if checks
if (first !== null || first !== undefined || first !== '') {
let second = first;
}
Instead of writing so many checks, we can write it better this way using ||
(OR) operator.
const second = first || '';
4. Shorthand for switch case
switch (number) {
case 1:
return 'one';
case 2:
return 'two';
default:
return;
}
Use a map/ object to write it in a cleaner way.
const data = {
1: 'one',
2: 'two'
};
//Access it using
data[num]
5. Shorthand for functions with a single line
function doubleOf(value) {
return 2 * value;
}
Use the arrow function to shorten it.
const doubleOf = (value) => 2 * value
Top comments (39)
Do some performance testing of both codes in first example and see the difference
On both iOS Safari/Chrome/Firefox it is 90% slower, on Desktop except chrome, Safari and Firefox are 90% slower.
It's also exhausting how often claiming "premature optimization" is used to justify sloppy thinking.
Don’t pessimize prematurely:
Alexandrescu, Andrei. "Modern C++ Design: Generic Programming and Design Patterns Applied", Small Object Allocation, p.77, 2001.
Stated differently:
Rich Hickey:
V8 is an amazing piece of technology but its heuristics are so complex that the smallest thing can derail JavaScript performance, so it's very inconsistent — at least when compared to languages that are compiled prior to deployment. From that perspective it makes sense to feed it code where is doesn't have to "guess" too much.
WebAssembly for Web Developers (Google I/O ’19):
And finally in the face of the continued proliferation of low-power / low-end / low-spec / small-core devices it seems foolish to rely on the JIT having access to the necessary CPU cycles to reliably optimize the code in a reasonable amount of time at runtime (perhaps we need profiling transpilers - but establishing representative "real-world" operational profile(s) can be a challenge in itself).
Meanwhile the self-improvement industry is pushing for aggregation of marginal gains - which is all about eliminating pessimization.
In the meantime real-life users have to deal with this.
Attitudes like that play right into Apple's hands if you believe that the "state of Safari" reflects a desire to deemphasize the importance and relevance of the web.
Then for a web professional that mindset is equivalent to "sawing off the branch you're sitting on".
There is a difference between "efficient coding idioms" and "premature optimization" and those two should not be confused.
PS: Then again maybe it's Chrome that is the real problem: Breaking the web forward.
Saying it works on Chrome is the web equivalent of "it works on my machine" — and fundamentally fails to recognize the nature of the web — there is no web platform, there’s an immensely varied collection of web platforms so lots of common wisdom from the backend doesn't directly apply.
And how is giving Safari users an adequate UX playing into Apple's hands? Apple doesn't benefit if you actually poly- and ponyfill Safari's inadequacies as you help to keep the web working. Most iOS user's don't realize that iOS Chrome is just Safari with a paint job so if the web is doing poorly on their flagship device it must be the web's fault, not Apple's.
The issue is the difference between "efficient coding idioms" vs. "premature optimizations".
If your application has to only work over a cooperate intranet with a strictly standardized web browser it's easy to determine what works and what doesn't. Over the public web matters are much more complicated and much less predictable especially when JavaScript is involved. So the blanket
without consideration of any type of context is entirely inappropriate. For example the iteration mechanism that is consistently performant across the majority of situations is the plain for loop. Does that mean bad things will happen if you prefer array functions? Not likely but context matters. As always — it depends.
The other issue is that current benchmarks don't typically cover memory pressure.
I agree that this is premature optimization and for a simpler reason: This use case can't be a hot-path because you always will have a small list and even in the case that it is a hot-path you will end up using a database if the list grows too much, so by that time you won't be using hard-coded variables anyway.
But I'd like to point out (not for you, but anyone reading this) that 'includes' itself time complexity IS linear O(n), as described in the spec (Step 10, tc39.es/ecma262/#sec-array.prototy... ):
It just happens to be constant in this case because our data initialization: the array is hard-coded, so it is a fixed constant (as you noted in your article), but the algorithm of the method by itself is O(n) in the scope of the algorithm (and because the worst case for the algorithm is not fixed data initialization I would still say that this is O(n) in a wider scope IMO, just in case we change our data initialization in the future which is not that uncommon).
I just wanted to clarify that to avoid any distracted developers reading this to misleadingly think that 'includes' is O(1) because it uses some kind of hash table.
And yeah, this is premature optimization.
Edit. Fix spec link.
+1
99% of the times O(1) level optimization is not required. So maintainability of the code should be preferred.
Hmm you are correct, people who built these performance metrics aren’t smart. !!
I don't think we should worry so much about performance. Yeah it's nice to know and be aware of it, but we should not be conditioned to use a "way" instead of another just because it scores 10% more in some random benchmark. Also as many people pointed out, it differs from browser to browser, and from machine to machine.
We are almost never doing so many operation that we need to worry about these minor performance improvements. User's will not even realize it. Better to worry about UX, page load speed, etc... Though if your doing some intensive work (games, machine learning, etc...) you might benefit from these improvements in some way, but there are other areas where you could benefit more (using efficient data structures that fit your needs for example, instead of just arrays).
The amount of arguing you guys have done over this when in real programs it doesn't matter which one you use. 90% slower when it executes 839Mops/s versus 25Mops/s and you're literally doing like 10ops max.... come on guys let it go, it's completely irrelevant.
||
is as outdated asfunction
. These two operator have two drastically different semantics:||
in case left-hand value is falsy.??
in case left-hand value is not defined (undefined
ornull
).Both have their usage and are not interchangeable.
??
is great whenfalse
is an intentional value.Parenthesis in functions is not something you should spend your time on, use Prettier.
On Firefox, the
||
method was almost twice as fast asincludes
. So, if there's almost no difference on Chrome, and a big difference in favour of||
on Firefox,||
would seem the better choice overall for performance? jsbench.me/njkuruuurr/1On mobile, difference is 90%, try it and then see. You are creating an array, unnecessary memory allocation and calling a method, which are multiple cpu cycles. Compound it multiple time used in single script it is by far the worst practice.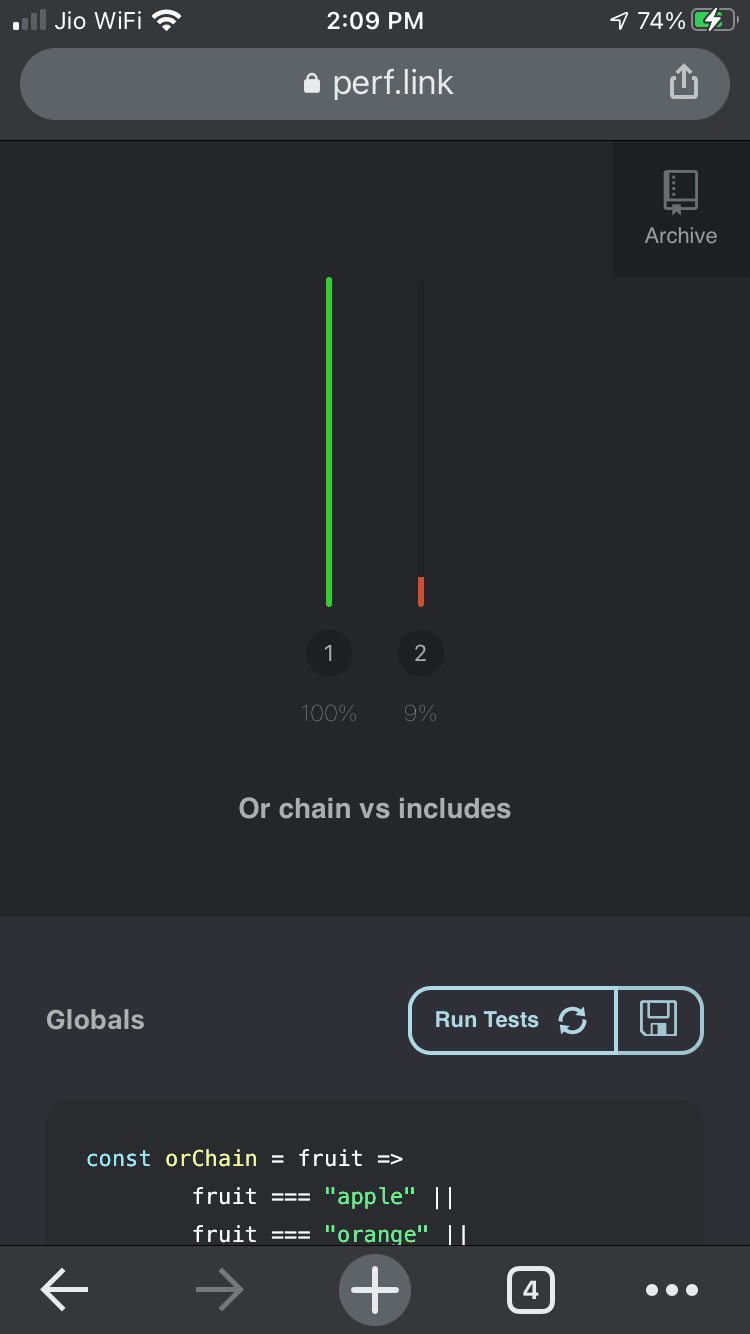
90% ? are you kidding me ? talk is cheap, show me your testing.
Globally Apple has a 15% Marketshare, 53% in North America, 32% in Europe — those users are locked into a "shitty browser" and not willing to forego their other creature comforts they are not going to switch brands on your account.
Meanwhile Chrome even on desktop has a reputation for being CPU and memory hungry. Apparently some people want lighter-weight alternatives. One has to wonder how pessimized JavaScript performs on Google GO on a "shitty phone".
Strong disagree on using
=>
overfunction
. You'll have a confusing time withthis
.You should not use "this" in modern JavaScript in the first place.
Because?
Because it doesn't promote arguably better immutable state.
But hey, it's a bold statement and I wouldn't say it always applies.
For #1, another option is to use
find
instead. That way it stops execution on the first found match.iPhone 6s Plus iOS 14.7.1


While Safari is primarily to blame here, this phone's CPU is still better than what's on a lot of contemporary run-of-mill android phones.
Would be interesting to also see performance benchmarks on each pair
These are good tips:
The performance difference is irrelevant, the array includes way feels cleaner but is actually harder to read because you don't immediately see what you're comparing the value to, and the more items in the list, the farther away the variable is visually so it actually makes the code harder to understand for even slightly more complex lists.
Optional chaining is terrible terrible and let me say it again, terrible.
See. If obj?.address?.postalCode fails, you don't know if obj doesn't exist, or obj.address doesn't exist or if it's just the obj.address.postalCode that's missing.
This WILL [not can] lead to problems where no error happens even though an important piece of information is missing and debugging it is a mess where you end up splitting it up anyway so it's better to validate each part of the object exists before using it.
You'll never do second=first....if so you would just use "first" in the first place.
That scenario literally never happens unless you're writing extra code and creating extra variables you don't need.
I like this one, i usually use arrays for it, but objects work too.
If a function has one line in it...it shouldn't be a damn function. That level of abstraction makes systems a bloody nightmare to debug because you're jumping 20 functions deep to find the culprit and often in 20 different files. Just KISS dammit.
Some comments may only be visible to logged-in visitors. Sign in to view all comments.