Namaste Everyone! I am thrilled to share my learning journey, transitioning from React → Angular, and finally embracing the incredible world of Next.js. As a perpetual wanderer on the quest for knowledge, I constantly seek to broaden my horizons and share the valuable lessons I've acquired along the way.
Lets have brief of what we are gonna discuss in this blog . Primarily Next.js and its rules (May be Typescript as a prerequsite). Routing in Next.js is actually good so will be explaining it . And Finally and webapp i was working on using Next.js and Github API .
What is Next.js and why it is Hyped ?
Next.js is a popular and highly regarded open-source React framework which was created by Vercel that simplifies the process of building robust and efficient web applications. Here's why it has garnered so much hype:
- React Framework with Batteries Included: Next.js is built on top of React, providing a structured and opinionated approach to building React applications. It comes with many built-in features, such as server-side rendering (SSR) and static site generation (SSG), making it easier for developers to create performant and scalable applications without having to configure everything from scratch.
- Efficient Server-Side Rendering (SSR) and Static Site Generation (SSG): One of the key strengths of Next.js is its ability to render pages on the server side, delivering a more optimized initial load time and improved SEO. It also supports static site generation, allowing you to pre-render pages at build time for even faster loading speeds.
- Intuitive Routing: Next.js provides a simple and intuitive routing system, making it easy to navigate between pages in your application. The file-based routing system allows developers to organize their code logically and facilitates a clean project structure.
- TypeScript Support: Next.js has excellent TypeScript support out of the box. This makes it a preferred choice for developers who value static typing and want to catch potential errors early in the development process.
- Developer Experience: The framework prioritizes a great developer experience with features like fast refresh, which allows for quick and efficient development without losing state. It also integrates well with popular development tools, enhancing the overall workflow.
Lets Begin with actual project
The project I did was and Github DevCard Generator.The Github Dev Card project provides a personalized and visually appealing solution to share your Github profile in a unique way. Tired of the standard Github profile pages? Now, with Github Dev Card, you can create your own custom card, making it easier and more enjoyable to share your Github information. This project simplifies the process, allowing users to generate a personalized Github card by simply entering their Github username. Additionally, users can easily download the card in high quality as a PNG image or share it instantly or share it via good old FreeShare (Yeah my own previous project 😁).
Creating new Next.js project
Creating a new Next.js project is a straightforward process. Make sure you have Node.js installed on your machine before you begin. Here are the steps:
- Initialize a new Next.js project: Open your terminal and run the following commands: ```bash
npx create-next-app your-project-name
2. **Navigate to the project directory**
```bash
cd your-project-name
- Run the development Server ```bash
npm run dev
This command will start the development server, and you can view your Next.js app by visiting http://localhost:3000 in your web browser.
## Project Structures
Next.js has a predefined project structure. Key folders include:
- **App Folder** : This folder is the main feature of Next.js 13. You can handle all the page and API routes within this folder. I prefer to create a separate folder named “api” for API routes. I feel more organized this way. There is tons of options that you can use in the “app” folder. Fore detailed information, take a look at the [offical NextJS documantation](https://nextjs.org/docs/app/building-your-application/routing).
- **Actions Folder** : In Next.js, the server actions folder is a built-in solution for server mutations. When an action is invoked, Next.js can return both the updated UI and new data in a single server roundtrip.
- **Components Folder** : The only point I would like to make is that you can include “style” and “test” files within a component folder. In this particular case, I prefer to maintain them in separate folders.
- **Types Folder** : Indeed, the nomenclature of this folder aligns with its intended purpose. Within, I have housed all the TypeScript types that are employed throughout the project.
## Github DevCard Generator
Before we dive into building our GitHub DevCard Generator, let's start by obtaining a GitHub access token. This token will allow us to make authenticated requests to the GitHub API.
### Step 1: Obtain GitHub Access Token
1. Visit [GitHub Token Settings](https://github.com/settings/tokens) to generate a personal access token.
2. Click on "Generate token" and provide the necessary scopes for your project. At least, include `read:user` and `repo` scopes for user and repository-related information.
3. Copy the generated token securely.
Now that we have our access token, let's explore different GitHub API endpoints that will help us gather the required details for our DevCard.
### Step 2: Explore GitHub API Endpoints
Here are some key GitHub API endpoints you might find useful:
#### 1. Get User Information
```bash
GET https://api.github.com/users/{username}
2. Get Repositories for a User
GET https://api.github.com/users/{username}/repos
3. Get Repository Information
GET https://api.github.com/repos/{owner}/{repo}
4. Get Repository Languages
GET https://api.github.com/repos/{owner}/{repo}/languages
5. Get User's Starred Repositories
GET https://api.github.com/users/{username}/starred
Make sure to include your GitHub access token in the request headers for authentication.
Code Overview
Firstly need to get the user data of User using github username so need to cll the api and store the data .
Getting UserData and RepoData of a user
In the provided useEffect block, a function named fetchData is defined to asynchronously fetch data from the GitHub API for a specified user (dataUser). The function encapsulates two asynchronous operations: fetching the user data using the fetchUserData function and the user's repositories data using the fetchUserRepos function. Upon successful data retrieval, the user and repositories data are set using the setUserData and setReposData state update functions, respectively.
The fetchUserData and fetchUserRepos functions are responsible for making the actual API requests. They use the fetch function to send GET requests to the GitHub API endpoints for user information and user repositories. These requests include an Authorization header with a Bearer token (authToken) to authenticate the API calls.
Error handling is incorporated within a try-catch block, where any errors during the data fetching process are caught and logged to the console using console.error. The entire fetchData function is invoked when the component mounts or when the dependencies (dataUser and authToken) change, ensuring that the data is fetched and updated accordingly.
Make sure you have proper Authtoken and also for good pratice use it in .env file and then use.
useEffect(() => {
const fetchData = async () => {
try {
const userData = await fetchUserData(dataUser);
setUserData(userData);
const reposData = await fetchUserRepos(dataUser);
setReposData(reposData)
} catch (error) {
console.error('Error fetching data:', error);
}
};
const fetchUserData = async (username) => {
const response = await fetch(`https://api.github.com/users/${username}`, {
headers: {
Authorization: `Bearer ${authToken}`,
},
});
return response.json();
};
const fetchUserRepos = async (username) => {
const response = await fetch(`https://api.github.com/users/${username}/repos`, {
headers: {
Authorization: `Bearer ${authToken}`,
},
});
return response.json();
};
fetchData();
}, [dataUser, authToken]);
For Advanced data like total number of Stars earned and total languages
In below code block, a React useEffect hook is employed to fetch data related to a GitHub user and their repositories. The fetchData function orchestrates asynchronous requests to the GitHub API for user data and repository information associated with a specified GitHub username (dataUser).This code extends its functionality to gather advanced data, including the total number of stars earned across all repositories (totalStars) and the list of unique programming languages used (uniqueLanguages). These advanced data points are calculated and set using the setTotalStars and setUniqueLanguages state update functions.
useEffect(() => {
const fetchData = async () => {
try {
const userData = await fetchUserData(dataUser);
setUserData(userData);
const reposData = await fetchUserRepos(dataUser);
setReposData(reposData);
// For getting Total stars earned by user for all his projects
const totalStars = reposData.reduce((acc, repo) => acc + repo.stargazers_count, 0);
setTotalStars(totalStars);
// Total Languages User worked on
const languagesSet = new Set();
reposData.forEach((repo) => {
if (repo.language) {
languagesSet.add(repo.language);
}
});
const uniqueLanguages = Array.from(languagesSet);
setUniqueLanguages(uniqueLanguages);
} catch (error) {
console.error('Error fetching data:', error);
}
};
const fetchUserData = async (username) => {
// As given in previous block
};
const fetchUserRepos = async (username) => {
// As given in previous block
};
fetchData();
}, [dataUser, authToken]);
Routing in Next.js
The App Router works in a new directory named app. The app directory works alongside the pages directory to allow for incremental adoption. This allows you to opt some routes of your application into the new behavior while keeping other routes in the pages directory for previous behavior.
Note : The App Router takes priority over the Pages Router. Routes across directories should not resolve to the same URL path and will cause a build-time error to prevent a conflict.
- Folders are used to define routes. A route is a single path of nested folders, following the file-system hierarchy from the root folder down to a final leaf folder that includes a page.js file.
- For creating Dynamic Routes you can use folder with "[<-folder-name->]" and inside that add page.jsx file.To get the dynamic value we can use below : ```typescript
export default function User({ params }: { params: { userName: string } }) {
console.log(userName,"userName");
}
Below is the folder structure for that :
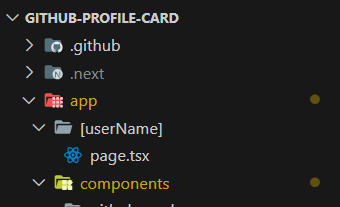
### Using any UI Library ft. CharkaUI
Using a UI library in your web development project offers several benefits that can significantly enhance the development process and the quality of the user interface.
For this project i am using **[Chakra UI](https://chakra-ui.com/getting-started/nextjs-app-guide)**.
Chakra UI is a popular React component library designed to streamline the process of building modern and visually appealing user interfaces. Its main advantages lie in its simplicity, customization capabilities, and accessibility features.
1. Install Chakra UI :
**npm**
```bash
npm i @chakra-ui/react @chakra-ui/next-js @emotion/react @emotion/styled framer-motion
yarn
yarn add @chakra-ui/react @chakra-ui/next-js @emotion/react @emotion/styled framer-motion
- Setup ChakraProvider Next.js 13 introduced a new app/ directory / folder structure. By default it uses Server Components. However, Chakra UI only works in client-side components. To use Chakra UI in server components, you need to convert them into client-side component by adding a 'use client'; at the top of your file.
// app/providers.tsx
'use client'
import { ChakraProvider } from '@chakra-ui/react'
export function Providers({ children }: { children: React.ReactNode }) {
return <ChakraProvider>{children}</ChakraProvider>
}
- Setup Layout Inside app/layout.tsx add below code ```typescript
// app/layout.tsx
import { Providers } from './providers'
export default function RootLayout({
children,
}: {
children: React.ReactNode,
}) {
return (
{children}
)
}
## Usage of DevCard

Using Github Dev Card is a straightforward process:
1. Visit the [Github Dev Card](https://github-devcard.vercel.app) website.
2. Enter your Github username.
3. Select the preferred style (Minimalistic, Minimalistic Dark Mode, or Futuristic).
4. Click on the "Submit" button.
Your personalized Github Dev Card will be generated instantly. From here, you can download the card as a PNG image or share it directly.
## Features
1. **Easy Generation of Github Dev Card**
The primary feature of this project is the ability to create a custom Github Dev Card effortlessly. Users only need to input their Github username, and the card is automatically generated with all relevant details.
2. **High-Quality PNG Download**
Users can download their generated Github Dev Card as a high-quality PNG image. This feature is useful for those who want to save or share the card locally.
3. **Instant Share**
In addition to downloading, users can instantly share their Github Dev Card without the need for a download. The project incorporates the FreeShare functionality, making sharing seamless.
4. **Multiple Stylish Options**
Github Dev Card offers users a choice of styles for their cards, including Minimalistic, Minimalistic Dark Mode, and Futuristic. This customization allows users to select a style that suits their preferences.
5. **3D Tilt View**
One of the Card offeres 3D tilt view moreover it works with gravity (in Mobile View) and Mouse Movement (Desktop View).
## Installation
To set up Github Dev Card locally or contribute to the project, follow these steps:
1. Clone the repository:
```bash
git clone https://github.com/Varshithvhegde/Github-Profile-Card.git
- Install dependencies: ```bash
cd Github-Profile-Card
npm install
3. Environment Variables
Create a file **.env** in the root folder and add your own [github api token](https://github.com/settings/tokens) as below
NEXT_PUBLIC_GITHUB_TOKEN=YOUR_GITHUB_TOKEN
4. Run the development server:
```bash
npm run dev
Open http://localhost:3000 in your browser to view the project.
So this is the end please enjoy yourself using this devcard. If you liked it please post it on Social Media Tagging Me(Social Media links are below). You have created something like this yourself please let me know in the comments . And also I know I may have not used good practices since I am also an begineer in Next.js . So please let me know if something is wrong or could have been done better .
Finally, There is a small easter egg try find it and let me know if you find that 😉.
Links
- Github DevCard Generator
- FreeShare
- Github Repo
- Instagram - varshithvh
- X(Formerly Twitter) - VarshithVhegde1
Sayonara 👋, Happy Coding 🚀.
Top comments (2)
Nice One
Thank You 🙌