Originally published on bendyworks.com.
I don't have a full theme designed yet but I am planning on going in a clean and minimalist direction. So let's take the current default design and clean it up a little.
There are a couple of changes to the code for this theme cleanup. The first we'll cover is changing the status bar and navigation bar colors. The SystemChrome
change will go in the main
function. We'll also have to import services
to get access to SystemChrome
.
import 'package:flutter/services.dart';
void main() {
runApp(MyApp());
SystemChrome.setSystemUIOverlayStyle(
const SystemUiOverlayStyle(
statusBarColor: Colors.white,
systemNavigationBarColor: Colors.white,
systemNavigationBarDividerColor: Colors.black,
systemNavigationBarIconBrightness: Brightness.dark,
),
);
}
~~~{% endraw %}
_Note that a full restart of the app is needed for the system UI color changes._
The next change is customizing the [theme](https://flutter.io/docs/cookbook/design/themes) on {% raw %}`MaterialApp`. These changes disable the debug banner, tell `MaterialApp` to be in `light` [brightness mode](https://docs.flutter.io/flutter/material/ThemeData/brightness.html), and set a few colors to white. The title is also changed to just `Birb`.
~~~dart
MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Birb',
theme: ThemeData(
brightness: Brightness.light,
primaryColor: Colors.white,
scaffoldBackgroundColor: Colors.white,
),
home: const MyHomePage(title: 'Birb'),
);
~~~{% endraw %}
The next change is to center the title of the {% raw %}`AppBar`{% endraw %} and remove it's {% raw %}`elevation` (shadow).
~~~dart
AppBar(
title: Center(
child: Text(widget.title),
),
elevation: 0.0,
),
~~~
And the final change is to update and center the placeholder text. This will eventually turn into a widget to display when there are no images to show.
~~~dart
const Center(
child: Text('No Birbs a birbing'),
)
~~~
Now we have this nice clean base theme to continue building on.
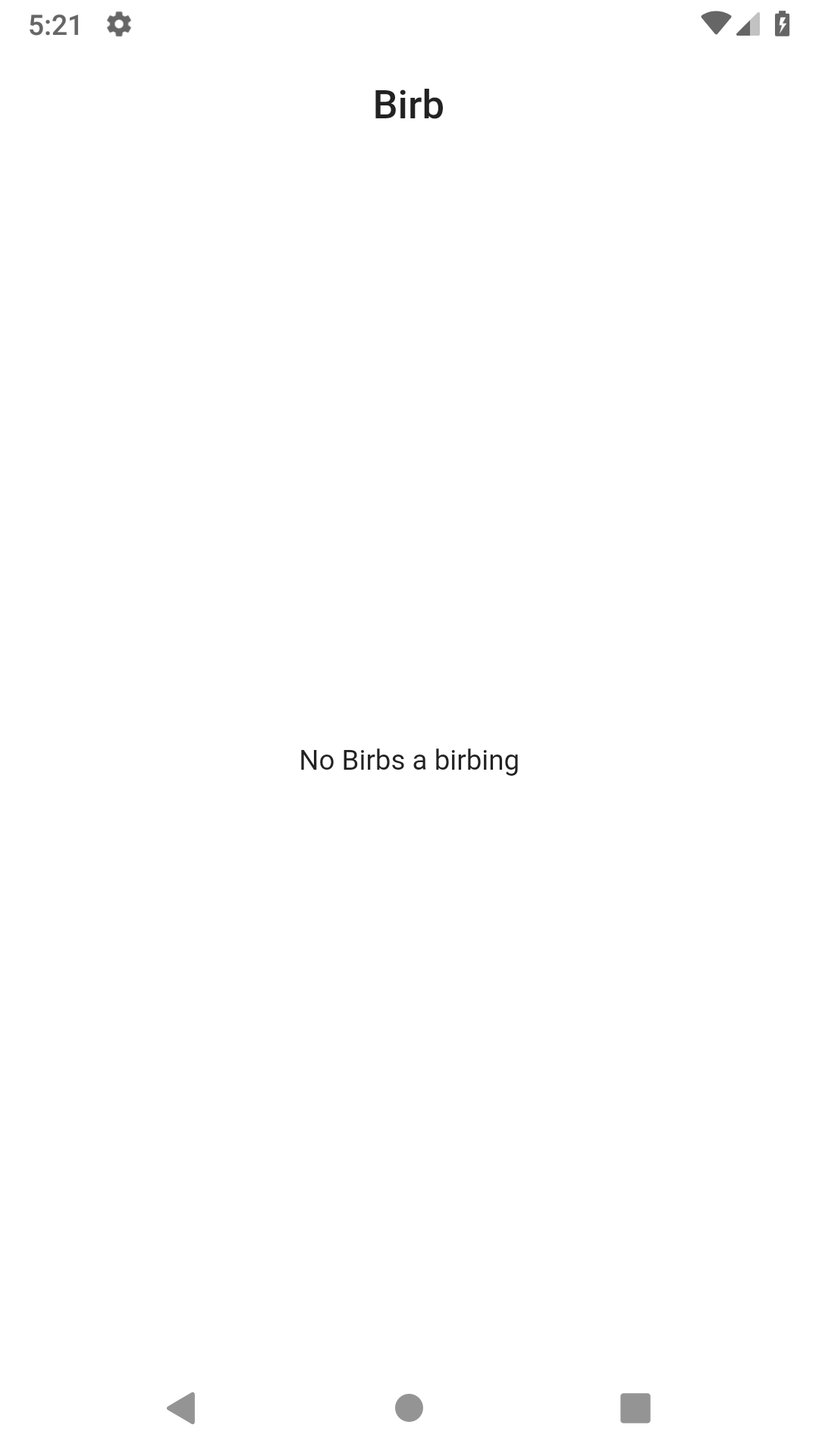
If you want to learn more about theming Flutter apps, check out the [Building Beautiful UIs with Flutter](https://codelabs.developers.google.com/codelabs/flutter/index.html) codelab.
## Code changes
- [#15 Implement a clean theme](https://github.com/abraham/birb/pull/15)
Top comments (2)
Thanks for this tiny insight of themeing, I believe many people will find it useful as I expect Flutter to gain a lot of momentum once they announced 1.0.
Will this month-of-flutter finish with some sort of standalone app like to-do? I'm just curious where are you going with it?
I talked a little bit about what I'm building in the original post. It's basically an Instagram clone.